- Python »
- 3.8.18 Documentation »
- The Python Standard Library »
- Theme Auto Light Dark |

Built-in Constants ¶
A small number of constants live in the built-in namespace. They are:
The false value of the bool type. Assignments to False are illegal and raise a SyntaxError .
The true value of the bool type. Assignments to True are illegal and raise a SyntaxError .
The sole value of the type NoneType . None is frequently used to represent the absence of a value, as when default arguments are not passed to a function. Assignments to None are illegal and raise a SyntaxError .
Special value which should be returned by the binary special methods (e.g. __eq__() , __lt__() , __add__() , __rsub__() , etc.) to indicate that the operation is not implemented with respect to the other type; may be returned by the in-place binary special methods (e.g. __imul__() , __iand__() , etc.) for the same purpose. Its truth value is true.
When a binary (or in-place) method returns NotImplemented the interpreter will try the reflected operation on the other type (or some other fallback, depending on the operator). If all attempts return NotImplemented , the interpreter will raise an appropriate exception. Incorrectly returning NotImplemented will result in a misleading error message or the NotImplemented value being returned to Python code.
See Implementing the arithmetic operations for examples.
NotImplementedError and NotImplemented are not interchangeable, even though they have similar names and purposes. See NotImplementedError for details on when to use it.
The same as the ellipsis literal “ ... ”. Special value used mostly in conjunction with extended slicing syntax for user-defined container data types.
This constant is true if Python was not started with an -O option. See also the assert statement.
The names None , False , True and __debug__ cannot be reassigned (assignments to them, even as an attribute name, raise SyntaxError ), so they can be considered “true” constants.
Constants added by the site module ¶
The site module (which is imported automatically during startup, except if the -S command-line option is given) adds several constants to the built-in namespace. They are useful for the interactive interpreter shell and should not be used in programs.
Objects that when printed, print a message like “Use quit() or Ctrl-D (i.e. EOF) to exit”, and when called, raise SystemExit with the specified exit code.
Objects that when printed or called, print the text of copyright or credits, respectively.
Object that when printed, prints the message “Type license() to see the full license text”, and when called, displays the full license text in a pager-like fashion (one screen at a time).
Table of Contents
- Constants added by the site module
Previous topic
Built-in Functions
Built-in Types
- Report a Bug
- Show Source
An Introduction to Python Built in Constants: True, False, and None in Python
Three commonly used built-in constants:
- True : The true value of the bool type. Assignments to True raise a SyntaxError .
- False : The false value of the bool type. Assignments to False raise a SyntaxError .
- None : The sole value of the type NoneType . None is frequently used to represent the absence of a value, as when default arguments are not passed to a function. Assignments to None raise a SyntaxError .
Other built-in constants:
- NotImplemented : Special value which should be returned by the binary special methods, such as __eg__() , __add__() , __rsub__() , etc.) to indicate that the operation is not implemented with respect to the other type.
- Ellipsis : Special value used mostly in conjunction with extended slicing syntax for user-defined container data types.
- __debug__ : True if Python was not started with an -o option.
Constants added by the site module The site module (which is imported automatically during startup, except if the -S command-line option is given) adds several constants to the built-in namespace. They are useful for the interactive interpreter shell and should not be used in programs.
Objects that when printed, print a message like “Use quit() or Ctrl-D (i.e. EOF) to exit”, and when called, raise SystemExit with the specified exit code:
- quit(code=None)
- exit(code=None)
Objects that when printed, print a message like “Type license() to see the full license text”, and when called, display the corresponding text in a pager-like fashion (one screen at a time):
Proxy locations
North America
South America
See all locations
English (EN)
Back to blog
Python Syntax Errors: Common Mistakes and How to Fix Them
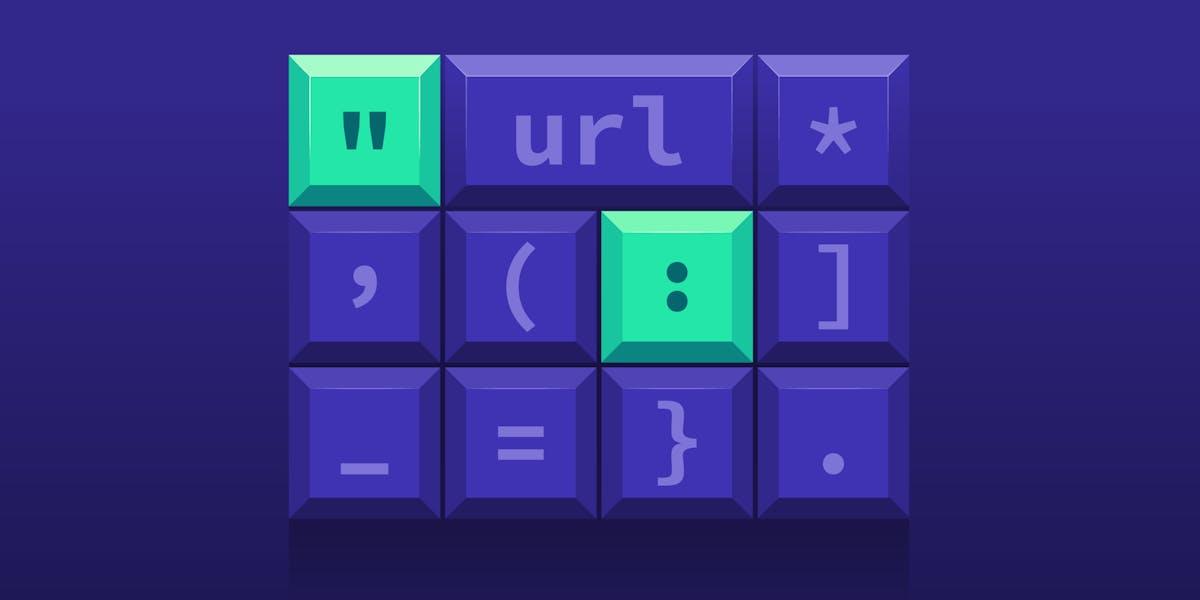
Vytenis Kaubrė
Whether you’re a true Pythonista or you’ve just dipped your toes into Python programming, you’ve probably had your battles with syntax errors. It takes a mere missing punctuation mark for the code to crash, and sometimes the error message doesn’t even give enough clues.
Read this article to learn how to understand, avoid, and fix Python syntax errors with the help of practical examples.
What are syntax errors in Python?
Syntax errors are invalidities in the code's structure. In other words, Python syntax errors happen when the Python interpreter is unable to recognize the structure of statements in code.
Every language follows certain rules to make sense of what’s being told and written. We can’t omit certain words and letters, as that can change the meaning entirely. Programming languages, including Python, are no exception. Your code must follow Python’s syntax rules for it to make sense.
Any programming language acts as a translator of human language to machine language. Hence, there are specific steps to code execution. When you run the code, the Python interpreter first parses your code into smaller components, then translates them into byte code, and eventually executes it.
During the parsing process, the interpreter analyzes your code for invalid syntax, and when it finds the first instance of incorrect syntax use, it gives you that unwelcome SyntaxError message.
How to read Python syntax errors
When you get an error message, Python tries to point to the root cause of the error. Sometimes, the message tells exactly what’s the problem, but other times it’s unclear and even confusing. This happens because Python locates the first place where it couldn’t understand the syntax; therefore, it might show an error in a code line that goes after the actual error.
Knowing how to read Python error messages goes a long way to save both time and effort. So let’s examine a Python web scraping code sample that raises two syntax errors:
In this example, we have a dictionary of different prices . We use a for loop to find and print the prices between $10 and $14.99. The price_found variable uses a boolean value to determine whether such a price was found in the dictionary.
When executed, Python points to the first invalid syntax error it came upon, even though there are two more errors along the way. The first error message looks like this:

Information in the yellow box helps us determine the location of the error, and the green box includes more details about the error itself. The full message can be separated into four main elements:
The path directory and name of the file where the error occurred;
The line number and the faulty code line where the error was first encountered;
The carets (^) that pinpoint the place of the error;
The error message determines the error type, followed by additional information that may help fix the problem.
The code sample produced a syntax error found in the first line of code – the prices dictionary. The carets indicate that the error occurred between “price2”: 13.48 and “price3”: 10.99 , and the invalid syntax message says that perhaps we forgot to add a comma between the items in our dictionary. That’s exactly it! The Python interpreter suggested the correct solution, so let’s update the code:
Now, rerun the code to see what’s the second syntax error:

This time, the carets fail to pinpoint the exact location of the error, and the SyntaxError message doesn’t include additional information about the possible solution. In such cases, the rule of thumb would be to examine the code that comes just before the carets. In the code sample, the syntax error is raised because there’s a missing comma between the variables key and value in the for loop. The syntactically correct code line should look like this:
When it comes to the causes of Python syntax errors, sometimes there’s just not enough information to get you on the right track. Case in point the above example of an invalid syntax error. Thus, it helps to know some of the common reasons that bring about syntax errors in Python to resolve them easily.
Common causes of syntax errors
Generally, you can expect common Python syntax errors to be caused by these invalidities in code:
Misplaced, missing, or mismatched punctuation (parentheses, brackets, braces, quotes, commas, colons, etc.);
Misspelled, misplaced, or missing Python keywords;
Illegal characters in variable names;
Incorrect indentation;
Incorrect use of the assignment operator (=).
The lack of experience with Python, copy-pasting errors, and typographical errors play a big role here. Especially the latter, as typos are easy to make and hard to spot, so it’s a great practice to double-check your code during production.
In any case, there are additional steps you can take when coding to decrease the chances of making a syntax error.
How to avoid Python syntax errors?
Use an integrated development environment (IDE) or a code editor that highlights the reserved Python keywords and errors, as well as suggests edits as you write your code. This is the first and foremost step you should take to improve your code and avoid invalid syntax errors in Python.
Frequently test your code as you write it. This helps to find syntax errors from the get-go and minimizes the risk of repeating the same mistakes down the road.
Use consistent indentation. Incorrect indentation is one of the more frequent causes of syntax errors in Python, which can be avoided by consciously checking your code and using IDEs or code editors that highlight indentation errors.
Use linting and formatting modules that check your code for errors and style. Some modules can fix your code automatically, saving you time and effort. Linters and formatters are great when you only want to run Python in the Terminal without an IDE or a code editor. A few notable mentions include pycodestyle , pylint , pyflakes , Flake8 , and Black .
Read Python documentation. It’s indeed an obvious tip to give, but the official Python documentation offers lots of useful information that will not only deepen your knowledge but also help you understand Python’s syntax and the meaning of syntax error messages.
Remember that these steps can help you avoid common Python syntax errors but not eliminate them.
Even if your code is syntactically correct but something is off when the code runs – an exception occurs. Python runtime errors happen during code execution and change the normal flow of the program. Unlike syntax errors, which terminate the program once the error occurs, runtime errors can continue to run the program if proper exception handlers are used.
Furthermore, logical errors happen when the code is syntactically valid and runs without errors, but the output isn't what was expected. While this article examines invalid syntax problems, it should be noted that the SyntaxError exception is part of a wider group of exceptions . For advanced Python programming, learning about other errors and how to handle them is recommended.
How to fix syntax errors
Now that we’ve discussed the common causes of Python syntax errors and the general tips on avoiding them let’s see some actual code samples and fix them.
Misplaced, missing, or mismatched punctuation
1. Ensure that parentheses () , brackets [] , and braces {} are properly closed. When left unclosed, the Python interpreter treats everything following the first parenthesis, bracket, or brace as a single statement. Take a look at this web scraping code sample that sends a set of crawling instructions to our Web Crawler tool :
At first glance, it looks like the payload was closed with braces, but the Python interpreter raises a syntax error that says otherwise. In this particular case, the “filters” parameter isn’t closed with braces, which the interpreter, unfortunately, doesn’t show in its traceback. You can fix the error by closing the “filters” parameter:
2. Make sure you close a string with proper quotes. For example, if you started your string with a single quote ‘, then use a single quote again at the end of your string. The below code snippet illustrates this:
This example has two errors, but as you can see, the interpreter shows only the first syntax error. It pinpoints the issue precisely, which is the use of a single quote at the start, and a double quote at the end to close the string.
The second error is in the third example URL, which isn’t closed with a quotation mark at all. The syntactically correct version would look like this:
When the string content itself contains quotation marks, use single ‘ , double “ , and/or triple ‘’’ quotes to specify where the string starts and ends. For instance:
The interpreter shows where the error occurred, and you can see that the carets end within the second double quotation mark. To fix the syntax error, you can wrap the whole string in triple quotes (either ’’’ or “”” ):
3. When passing multiple arguments or values, make sure to separate them with commas. Consider the following web scraping example that encapsulates HTTP headers in the headers dictionary:
Again, the interpreter fails to show precisely where the issue is, but as a rule of thumb, you can expect the actual invalid syntax error to be before where the caret points. You can fix the error by adding the missing comma after the ‘Accept-Language’ argument:
4. Don’t forget to add a colon : at the end of a function or a compound statement, like if , for , while , def , etc. Let’s see an example of web scraping:
This time, the interpreter shows the exact place where the error occurred and hints as to what could be done to fix the issue. In the above example, the def function and the for loop are missing a colon, so we can update our code:
Misspelled, misplaced, or missing Python keywords
1. Make sure you’re not using the reserved Python keywords to name variables and functions. If you’re unsure whether a word is or isn’t a Python keyword, check it with the keyword module in Python or look it up in the reserved keywords list . Many IDEs, like PyCharm and VS Code, highlight the reserved keywords, which is extremely helpful. The code snippet below uses the reserved keyword pass to hold the password value, which causes the syntax error message:
2. Ensure that you haven’t misspelled a Python keyword. For instance:
This code sample tries to import the Session object from the requests library. However, the Python keyword import is misspelled as impotr , which raises an invalid syntax error.
3. Placing a Python keyword where it shouldn't be will also raise an error. Make sure that the Python keyword is used in the correct syntactical order and follows the rules specific to that keyword. Consider the following example:
Here, we see an invalid syntax error because the Python keyword from doesn’t follow the correct syntactical order. The fixed code should look like this:
Illegal characters in variable names
Python variables have to follow certain naming conventions:
1. You can’t use blank spaces in variable names. The best solution is to use the underscore character. For example, if you want a variable named “two words”, it should be written as two_words , twowords , TwoWords , twoWords , or Twowords .
2. Variables are case-sensitive, meaning example1 and Example1 are two different variables. Take this into account when creating variables and calling them later in your code.
3. Don’t start a variable with a number. Python will give you a syntax error:
As you can see, the interpreter allows using numbers in variable names but not when the variable names start with a number.
4. Variable names can only use letters, numbers, and underscores. Any other characters used in the name will produce a syntax error.
Incorrect indentation
1. Remember that certain Python commands, like compound statements and functions, require indentation to define the scope of the command. So, ensure that such commands in your code are indented properly. For instance:
The first error message indicates that the if statement requires an indented block. After fixing that and running the code, we encounter the second error message that tells us the print statement is outside the if statement and requires another indent. Fix the code with the correct indentation:
2. Use consistent indentation marks: either all spaces or all tabs. Don’t mix them up, as it can reduce the readability of your code, in turn making it difficult to find the incorrect indentation just by looking at the code. Most Python IDEs highlight indentation errors before running the code, so you can reformat the file automatically to fix the indentation.
Let’s take the above code sample and fix the first error message by adding a single space in front of the if statement:
The code works without errors and prints the correct result. However, you can see how the mix of spaces and tabs makes the code a little harder to read. Using this method can bring about unnecessary syntax errors when they can be avoided by sticking to either spaces or tabs throughout the code.
Incorrect use of the assignment operator (=)
1. Ensure you aren’t assigning values to functions or literals with the assignment operator = . You can only assign values to variables. Here’s an overview of some examples:
The string "price" can't act as a variable, therefore the quotation marks have to be removed:
In the following example, we want to check whether the value 10.98 is a float type:
The Python interpreter raises an error since the assignment operator can’t be used to assign a value to a function. The correct way to accomplish this is with one the following code samples:
2. Assign values in a dictionary with a colon : and not an assignment operator = . Let’s take a previous code sample and modify it to incorrectly use the assignment operator instead of colons:
3. Use == when comparing objects based on their values. For instance:
You can fix the issue by using the double equal sign == between price_1 and price_2 instead of = , which will print the correct result.
Final thoughts
Syntax errors in Python can be tough to crack, depending on the error. When you have a clear understanding of how error tracebacks work, along with the common reasons behind these errors and their possible solutions, you can significantly reduce the time and effort required to debug issues. Hopefully, this article helped you get on the right track to start producing syntactically flawless Python code.
You can find the source code files together with their respective fixes in our GitHub repository . In case you’re interested to learn more about web scraping with Python, check out our in-depth tutorial here .
If you have any questions about our products, our support team is always here to assist you 24/7. Reach out via email or live chat.
About the author
Vytenis Kaubrė is a Copywriter at Oxylabs. His love for creative writing and a growing interest in technology fuels his daily work, where he crafts technical content and web scrapers with Oxylabs’ solutions. Off duty, you might catch him working on personal projects, coding with Python, or jamming on his electric guitar.
Learn more about Vytenis Kaubrė
All information on Oxylabs Blog is provided on an "as is" basis and for informational purposes only. We make no representation and disclaim all liability with respect to your use of any information contained on Oxylabs Blog or any third-party websites that may be linked therein. Before engaging in scraping activities of any kind you should consult your legal advisors and carefully read the particular website's terms of service or receive a scraping license.
Related articles
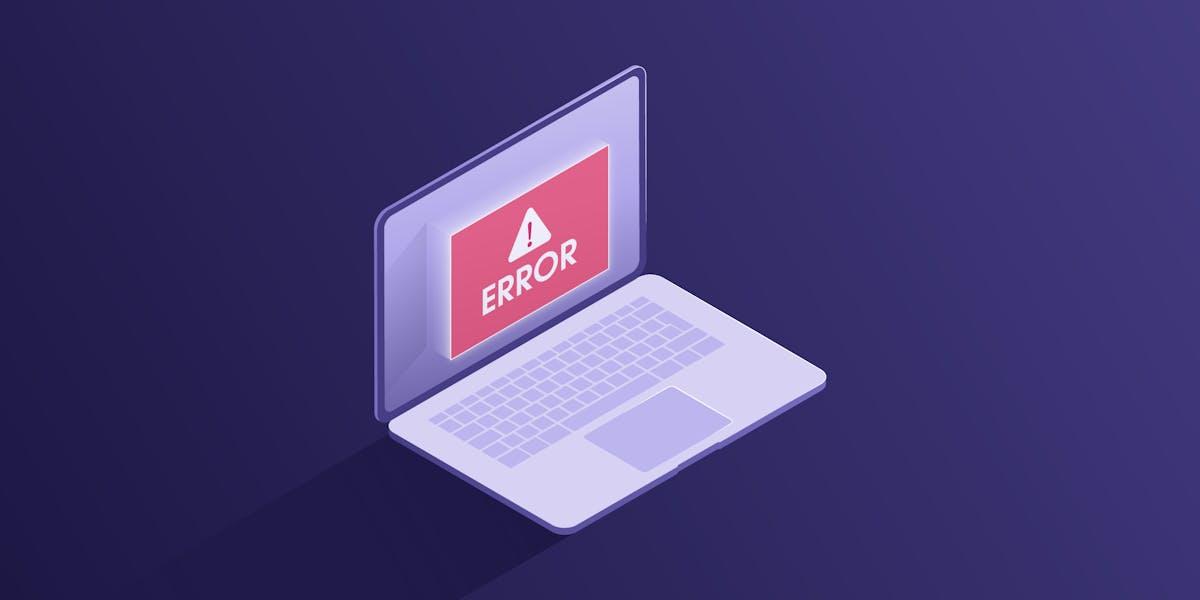
How to Solve Proxy Status Error Codes
Maryia Stsiopkina
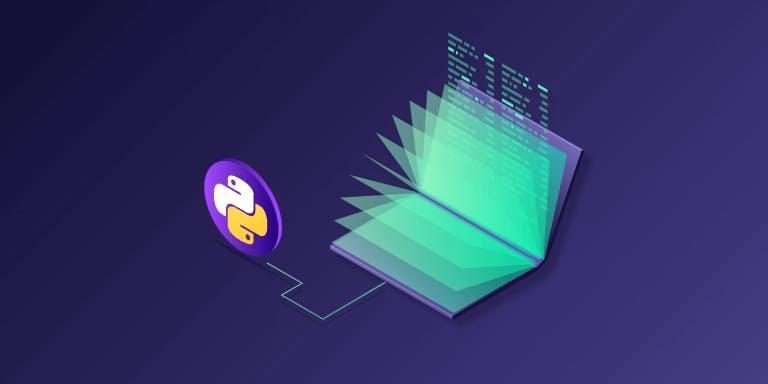
Python Requests Library: 2024 Guide
Adomas Sulcas
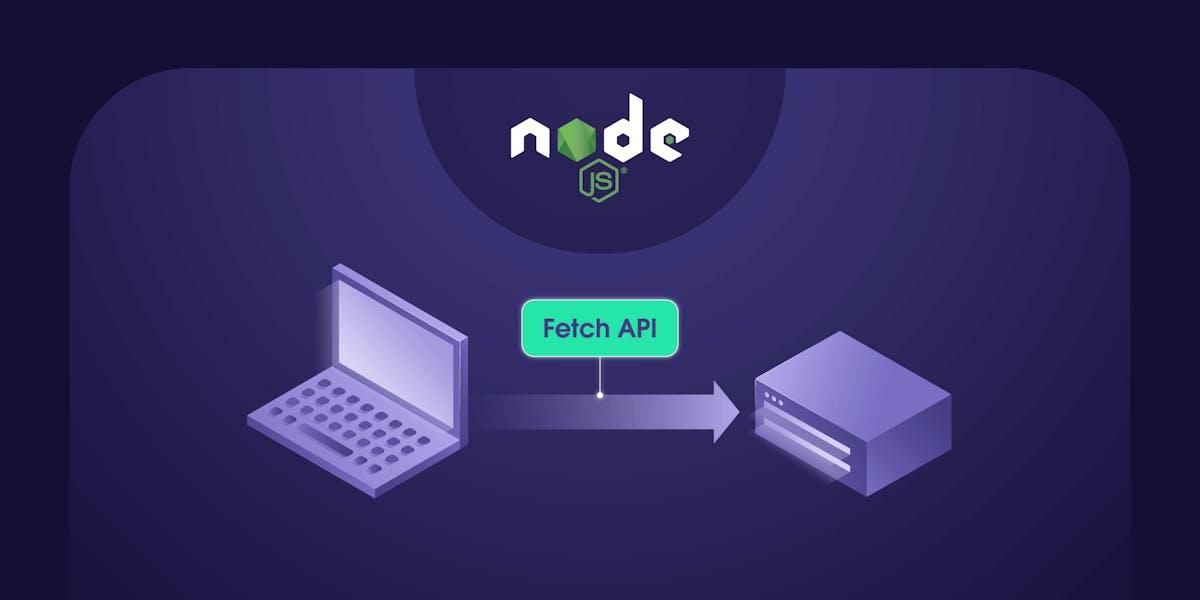
How to Make HTTP Requests in Node.js With Fetch API
Augustas Pelakauskas
Get the latest news from data gathering world
IN THIS ARTICLE:
Forget about complex web scraping processes
Choose Oxylabs' advanced web intelligence collection solutions to gather real-time public data hassle-free.
Scale up your business with Oxylabs ®
GET IN TOUCH
Certified data centers and upstream providers
Connect with us
- Affiliate program
- Service partners
- Residential Proxies sourcing
- Our products
- Project 4beta
- Sustainability
- Trust & Safety
- Datacenter Proxies
- Shared Datacenter Proxies
- Dedicated Datacenter Proxies
- Residential Proxies
- Static Residential Proxies
- SOCKS5 Proxies
- Mobile Proxies
- Rotating ISP Proxies
Advanced proxy solutions
- Web Unblocker
Top locations
- United States
- United Kingdom
- All locations
- Developers Hub
- Documentation
Scraper APIs
- SERP Scraper API
- E-Commerce Scraper API
- Web Scraper API
Innovation hub
- Adaptive Parser
- Intellectual Property
oxylabs.io © 2024 All Rights Reserved
- SyntaxError: cannot assign to expression here. Maybe you meant '==' instead of '='?
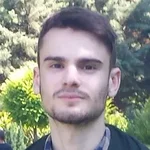
Last updated: Apr 8, 2024 Reading time · 6 min
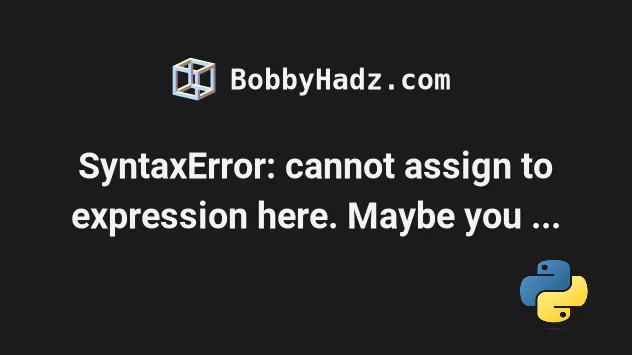
# Table of Contents
- SyntaxError: cannot assign to literal here (Python)
Note: If you got the error: "SyntaxError: cannot assign to literal here" , click on the second subheading.
# SyntaxError: cannot assign to expression here. Maybe you meant '==' instead of '='?
The Python "SyntaxError: cannot assign to expression here. Maybe you meant '==' instead of '='?" occurs when we have an expression on the left-hand side of an assignment.
To solve the error, specify the variable name on the left and the expression on the right-hand side.

Here is an example of how the error occurs.
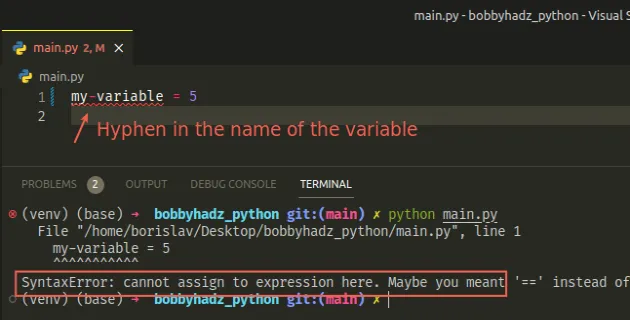
# Don't use hyphens in variable names
If this is how you got the error, use an underscore instead of a hyphen.
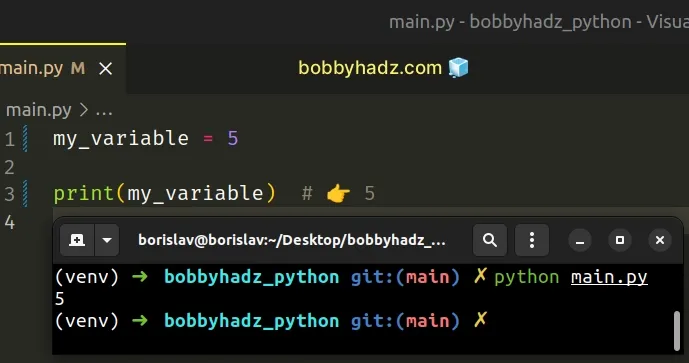
The name of a variable must start with a letter or an underscore.
A variable name can contain alpha-numeric characters ( a-z , A-Z , 0-9 ) and underscores _ .
Variable names cannot contain any other characters than the aforementioned.
# Don't use expressions on the left-hand side of an assignment
Here is another example of how the error occurs.
We have an expression on the left-hand side which is not allowed.
The variable name has to be specified on the left-hand side, and the expression on the right-hand side.
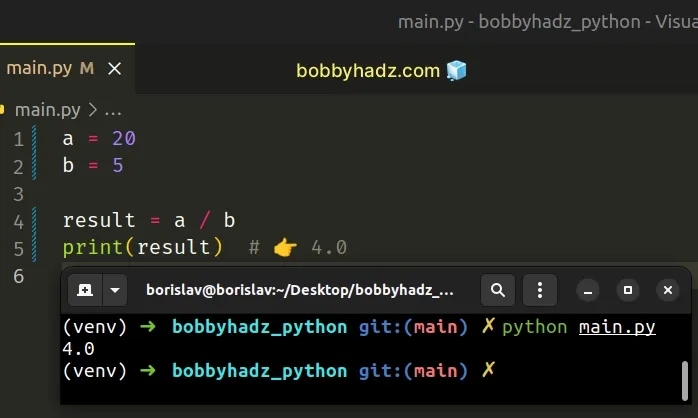
Now that the division is moved to the right-hand side, the error is resolved.
# Use double equals (==) when comparing values
If you mean to compare two values, use the double equals (==) sign.
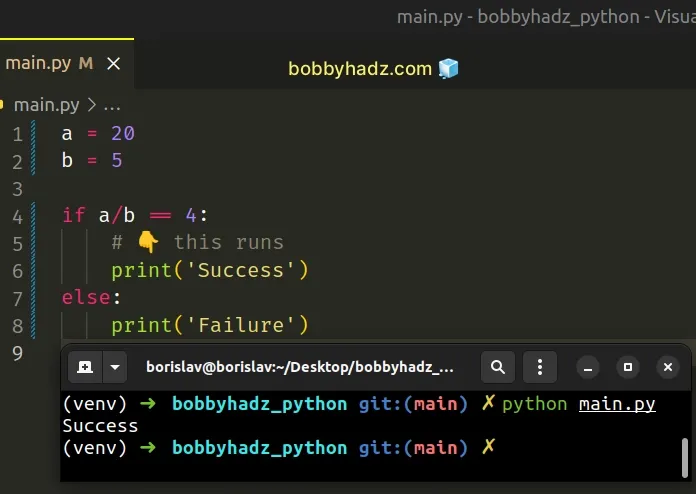
Notice that we use double equals == when comparing two values and a single equal = sign for assignment.
Double equals (==) is used for comparison and single equals (=) is used for assignment.
If you use a single equal (=) sign when comparing values, the error is raised.
# Declaring a dictionary
If you get the error when declaring a variable that stores a dictionary, use the following syntax.
Notice that each key and value are separated by a colon and each key-value pair is separated by a comma.
The error is sometimes raised if you have a missing comma between the key-value pairs of a dictionary.
# SyntaxError: cannot assign to literal here (Python)
The Python "SyntaxError: cannot assign to literal here. Maybe you meant '==' instead of '='?" occurs when we try to assign to a literal (e.g. a string or a number).
To solve the error, specify the variable name on the left and the value on the right-hand side of the assignment.
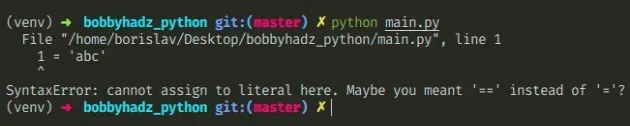
Here are 2 examples of how the error occurs.
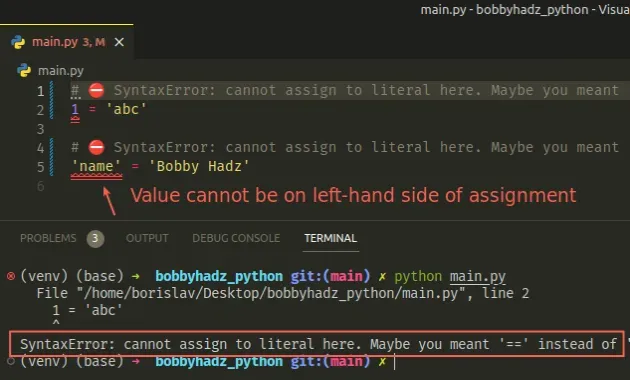
Literal values are strings, integers, booleans and floating-point numbers.
# Variable names on the left and values on the right-hand side
When declaring a variable make sure the variable name is on the left-hand side and the value is on the right-hand side of the assignment ( = ).
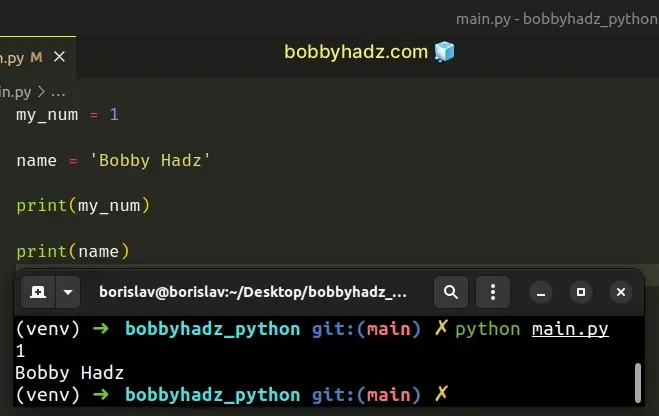
Notice that variable names should be wrapped in quotes as that is a string literal.
The string "name" is always going to be equal to the string "name" , and the number 100 is always going to be equal to the number 100 , so we cannot assign a value to a literal.
# A variable is a container that stores a specific value
You can think of a variable as a container that stores a specific value.
Variable names should not be wrapped in quotes.
# Declaring multiple variables on the same line
If you got the error while declaring multiple variables on the same line, use the following syntax.
The variable names are still on the left, and the values are on the right-hand side.
You can also use a semicolon to declare multiple variables on the same line.
However, this is uncommon and unnecessary.

# Performing an equality comparison
If you meant to perform an equality comparison, use double equals.
We use double equals == for comparison and single equals = for assignment.
If you need to check if a value is less than or equal to another, use <= .
Similarly, if you need to check if a value is greater than or equal to another, use >= operator.
Make sure you don't use a single equals = sign to compare values because single equals = is used for assignment and not for comparison.
# Assigning to a literal in a for loop
The error also occurs if you try to assign a value to a literal in a for loop by mistake.
Notice that we wrapped the item variable in quotes which makes it a string literal.
Instead, remove the quotes to declare the variable correctly.
Now we declared an item variable that gets set to the current list item on each iteration.
# Using a dictionary
If you meant to declare a dictionary, use curly braces.
A dictionary is a mapping of key-value pairs.
You can use square brackets if you need to add a key-value pair to a dictionary.
If you need to iterate over a dictionary, use a for loop with dict.items() .
The dict.items method returns a new view of the dictionary's items ((key, value) pairs).
# Valid variable names in Python
Note that variable names cannot start with numbers or be wrapped in quotes.
Variable names in Python are case-sensitive.
The 2 variables in the example are completely different and are stored in different locations in memory.
# Additional Resources
You can learn more about the related topics by checking out the following tutorials:
- SyntaxError: cannot assign to function call here in Python
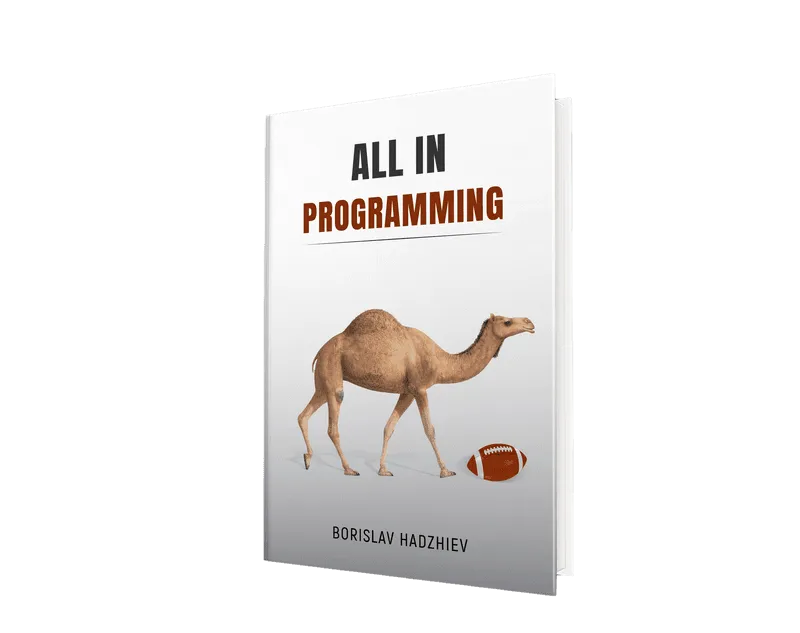
Borislav Hadzhiev
Web Developer
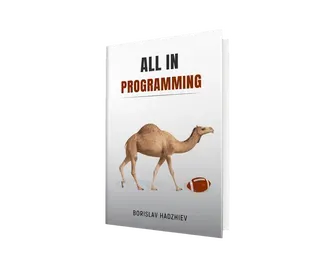
Copyright © 2024 Borislav Hadzhiev

- Python »
- 2.7.6 Documentation »
- The Python Standard Library »
4. Built-in Constants ¶
A small number of constants live in the built-in namespace. They are:
The false value of the bool type.
New in version 2.3.
The true value of the bool type.
The sole value of types.NoneType . None is frequently used to represent the absence of a value, as when default arguments are not passed to a function.
Changed in version 2.4: Assignments to None are illegal and raise a SyntaxError .
Special value which can be returned by the “rich comparison” special methods ( __eq__() , __lt__() , and friends), to indicate that the comparison is not implemented with respect to the other type.
Special value used in conjunction with extended slicing syntax.
This constant is true if Python was not started with an -O option. See also the assert statement.
The names None and __debug__ cannot be reassigned (assignments to them, even as an attribute name, raise SyntaxError ), so they can be considered “true” constants.
Changed in version 2.7: Assignments to __debug__ as an attribute became illegal.
4.1. Constants added by the site module ¶
The site module (which is imported automatically during startup, except if the -S command-line option is given) adds several constants to the built-in namespace. They are useful for the interactive interpreter shell and should not be used in programs.
Objects that when printed, print a message like “Use quit() or Ctrl-D (i.e. EOF) to exit”, and when called, raise SystemExit with the specified exit code.
Objects that when printed, print a message like “Type license() to see the full license text”, and when called, display the corresponding text in a pager-like fashion (one screen at a time).
Table Of Contents
- 4.1. Constants added by the site module
Previous topic
2. Built-in Functions
5. Built-in Types
- Report a Bug
- Show Source
Quick search
Enter search terms or a module, class or function name.
Assignments to True are illegal and raise a SyntaxError.
Expected either a logical, char, int, fi, single, or double. found an mxarray. mxarrays are returned from calls to the matlab interpreter and are not supported inside expressions. they may only be used on the right-hand side of assignments and as arguments to extrinsic functions., pgsql中multiple assignments to same column.
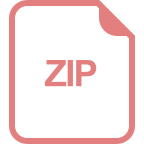
Personal Solutions to Programming Assignments on Matlab.zip
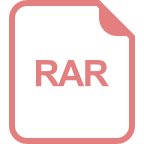
Assignments.rar
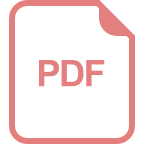
Eercise+Assignments+Class1A.pdf

parsing arror:Shorthand property assignments are valid only in
为什么会报错# cause: org.postgresql.util.psqlexception: error: multiple assignments to same column "rec pay id", assume you have four datasets: "employees", "departments", "projects", and "assignments". the scheme of these databases are as follows: the "employees" dataset contains employee information including a unique "employee_id", "employee_name", and "department_id". the "departments" dataset contains department information including a unique "department_id" and "department_name". the "projects" dataset contains project information including a unique "project_id", "project_name", and "department_id". the "assignments" dataset contains information about which employees are assigned to which projects, including the "employee_id" and "project_id" associated with each assignment. write a sql query to retrieve the name and department of all employees who are assigned to a project that is not in the same department as their own department., please rename our assignments uniformly and calculate a list of missing students. (some student assignments have been randomly deleted, only experimental examples, not actual results.)(code + result screenshot), the sleeping teaching assistant a university computer science department has a teaching assistant (ta) who helps undergraduate students with their programming assignments during regular office hours. the ta’s office is rather small and has room for only one desk with a chair and computer. there are three chairs in the hallway outside the office where students can sit and wait if the ta is currently helping another student. when there are no students who need help during office hours, the ta sits at the desk and takes a nap. if a student arrives during office hours and finds the ta sleeping, the student must awaken the ta to ask for help. if a student arrives and finds the ta currently helping another student, the student sits on one of the chairs in the hallway and waits. if no chairs are available, the student will come back at a later time. using posix threads, mutex locks, and/or semaphores, implement a solution that coordinates the activities of the ta and the students. details for this assignment are provided below. using pthreads, begin by creating n students. each will run as a separate thread. the ta will run as a separate thread as well. student threads will alternate between programming for a period of time and seeking help from the ta. if the ta is available, they will obtain help. otherwise, they will either sit in a chair in the hallway or, if no chairs are available, will resume programming and will seek help at a later time. if a student arrives and notices that the ta is sleeping, the student must notify the ta using a semaphore. when the ta finishes helping a student, the ta must check to see if there are students waiting for help in the hallway. if so, the ta must help each of these students in turn. if no students are present, the ta may return to napping. perhaps the best option for simulating students programming—as well as the ta providing help to a student—is to have the appropriate threads sleep for a random period of time using the sleep() api:, error in[<-.data. frame (*tmp* ,missing cols, value = 0) missing values are not allowed in subscripted assignments of data frames, 按照以下要求写出python代码,suppose there are n assignments a= [a1, a2 …ai …an]that you need to complete before the deadline. an assignment ai= [durationi, deadlinei] need durationi days to complete and must be completed before or on deadlinei. you can only do one assignment at a time and start next assignment once the current assignment is completed. assuming you start on day 1, implement an efficient algorithm to find the maximum number of assignments you can complete., 用python按以下要求写出代码,suppose there are n assignments a= [a1, a2 …ai …an]that you need to complete before the deadline. an assignment ai= [durationi, deadlinei] need durationi days to complete and must be completed before or on deadlinei. you can only do one assignment at a time and start next assignment once the current assignment is completed. assuming you start on day 1, implement an efficient algorithm to find the maximum number of assignments you can complete., error (10048): verilog hdl error at wjy.v(84): values cannot be assigned directly to all or part of array "memory_out" - assignments must be made to individual elements only, error (10110): verilog hdl error at traffic_light.v(7): variable "ew_time" has mixed blocking and nonblocking procedural assignments -- must be all blocking or all nonblocking assignments, error (10110): verilog hdl error at ad_acq.v(21): variable "select_fifo" has mixed blocking and nonblocking procedural assignments -- must be all blocking or all nonblocking assignments, when we use kmeans for image segmentation, the color information of pixels is used for clustering, so each of our pixels can be regarded as a vector composed of r, g, and b, and rgb is our color feature. the specific process is similar to our example above, but the calculation object is changed from a scalar to a 3-dimensional vector. please implement the kmean_color function in segmentation.py and call it to complete the segmentation of color images. (very similar to kmeans function)### clustering methods for colorful image def kmeans_color(features, k, num_iters=500): n=none # 像素个数 assignments = np.zeros(n, dtype=np.uint32) #like the kmeans function above ### your code here ### end your code return assignments.
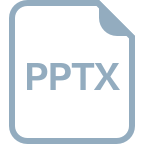
0 Reference Answer for Assignments.pptx
University-assignments-and-projects:大学课程作业.
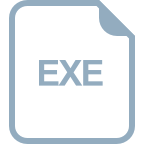
新北洋 BTP-R580II 驱动.exe
百达卡达晶控晶创延期.vmp.exe.
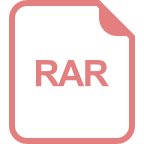
Java 开发--JSP毕业生招聘信息的发布与管理系统(论文+源代码+开题报告+外文翻译).rar
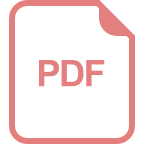
华大基因2023年9月生物信息分析水平初测试题
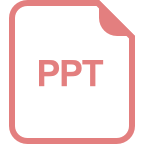
Matlab基本操作!!!!

IDEA 2023中如何导入和创建新项目

vs2022如何更新pip并安装pytext
Fast_algorithms_for_convolutional_neural_networks(快速神经网络入门资料).pdf.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- English (US)
SyntaxError: invalid assignment left-hand side
The JavaScript exception "invalid assignment left-hand side" occurs when there was an unexpected assignment somewhere. It may be triggered when a single = sign was used instead of == or === .
SyntaxError or ReferenceError , depending on the syntax.
What went wrong?
There was an unexpected assignment somewhere. This might be due to a mismatch of an assignment operator and an equality operator , for example. While a single = sign assigns a value to a variable, the == or === operators compare a value.
Typical invalid assignments
In the if statement, you want to use an equality operator ( === ), and for the string concatenation, the plus ( + ) operator is needed.
Assignments producing ReferenceErrors
Invalid assignments don't always produce syntax errors. Sometimes the syntax is almost correct, but at runtime, the left hand side expression evaluates to a value instead of a reference , so the assignment is still invalid. Such errors occur later in execution, when the statement is actually executed.
Function calls, new calls, super() , and this are all values instead of references. If you want to use them on the left hand side, the assignment target needs to be a property of their produced values instead.
Note: In Firefox and Safari, the first example produces a ReferenceError in non-strict mode, and a SyntaxError in strict mode . Chrome throws a runtime ReferenceError for both strict and non-strict modes.
Using optional chaining as assignment target
Optional chaining is not a valid target of assignment.
Instead, you have to first guard the nullish case.
- Assignment operators
- Equality operators

IMAGES
VIDEO
COMMENTS
The names None, False, True and __debug__ cannot be reassigned (assignments to them, even as an attribute name, raise SyntaxError), so they can be considered "true" constants. Constants added by the site module ¶
2. It's not really that True and False can be modified, so much as that True and False can be names, and if they are names, those names will shadow the names in builtin. That is True and False are not handled by the parser as special symbols, but appear to be looked up in the current local and global binding scope: >>> True = 'mumble'.
Three commonly used built-in constants: True : The true value of the bool type. Assignments to True raise a SyntaxError . False : The false value of the bool type ...
Note: The examples above are missing the repeated code line and caret (^) pointing to the problem in the traceback.The exception and traceback you see will be different when you're in the REPL vs trying to execute this code from a file. If this code were in a file, then you'd get the repeated code line and caret pointing to the problem, as you saw in other cases throughout this tutorial.
The names None, False, True and __debug__ cannot be reassigned (assignments to them, even as an attribute name, raise SyntaxError), so they can be considered "true" constants. Constants added by the site module ¶
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
False¶ The false value of the bool type. New in version 2.3. True¶ The true value of the bool type. New in version 2.3. None¶ The sole value of types.NoneType. None is frequently used to represent the absence of a value, as when default arguments are not passed to a function. Changed in version 2.4: Assignments to None are illegal and raise ...
Differentiating Exceptions From Syntax Errors. 00:00 In this lesson, you will learn how to differentiate between exceptions and syntax errors in Python. To show an example, I will head over to VS Code, and I have a script open that I called main.py. 00:13 I'm just going to write a bit of code in here, and you will keep using this script ...
Illegal characters in variable names; Incorrect indentation; Incorrect use of the assignment operator (=). The lack of experience with Python, copy-pasting errors, and typographical errors play a big role here. Especially the latter, as typos are easy to make and hard to spot, so it's a great practice to double-check your code during production.
PS C:\WINDOWS\system32> cd lpthw PS C:\WINDOWS\system32\lpthw> python ex6.py File "ex6.py", line 14 hilarious=False ^ SyntaxError: invalid syntax PS C:\WINDOWS\system32\lpthw> comments sorted by Best Top New Controversial Q&A Add a Comment
False¶ The false value of the bool type. New in version 2.3. True¶ The true value of the bool type. New in version 2.3. None¶ The sole value of types.NoneType. None is frequently used to represent the absence of a value, as when default arguments are not passed to a function. Changed in version 2.4: Assignments to None are illegal and raise ...
# SyntaxError: cannot assign to literal here (Python) The Python "SyntaxError: cannot assign to literal here. Maybe you meant '==' instead of '='?" occurs when we try to assign to a literal (e.g. a string or a number). To solve the error, specify the variable name on the left and the value on the right-hand side of the assignment.
The names None, False, True and __debug__ cannot be reassigned (assignments to them, even as an attribute name, raise SyntaxError), so they can be considered "true" constants. Constants added by the site module ¶
False¶ The false value of the bool type. New in version 2.3. True¶ The true value of the bool type. New in version 2.3. None¶ The sole value of types.NoneType. None is frequently used to represent the absence of a value, as when default arguments are not passed to a function. Changed in version 2.4: Assignments to None are illegal and raise ...
An assignment ai= [durationi, deadlinei] need durationi days to complete and must be completed before or on deadlinei. You can only do one assignment at a time and start next assignment once the current assignment is completed. Assuming you start on day 1, implement an efficient algorithm to find the maximum number of assignments you can complete.
Assignment Expression Syntax. For more information on concepts covered in this lesson, you can check out: Walrus operator syntax. One of the main reasons assignments were not expressions in Python from the beginning is the visual likeness of the assignment operator (=) and the equality comparison operator (==). This could potentially lead to bugs.
Invalid assignments don't always produce syntax errors. Sometimes the syntax is almost correct, but at runtime, the left hand side expression evaluates to a value instead of a reference, so the assignment is still invalid. Such errors occur later in execution, when the statement is actually executed. js. function foo() { return { a: 1 }; } foo ...
6. This x, y = (1, 2) is sequence assignment. It relies on the right hand side being an iterable object, and the left hand side being composed of the same number of variables as iterating the left hand side provides. This x, y -= (1, 2) is attempt to call the method __isub__ on the left hand operand (s). The nature of an in-place ("augmented ...
avId in self.disconnectedAvIds will return a boolean value.or is used to evaluate between two boolean values, so it's correct so far.. self.resultsStr += curStr is an assignment to a variable. An assignment to a variable is of the form lvalue = rvalue where lvalue is a variable you can assign values to and rvalue is some expression that evaluates to the data type of the lvalue.