- ▼Java Exercises
- ▼Java Basics
- Basic Part-I
- Basic Part-II
- ▼Java Data Types
- Java Enum Types
- ▼Java Control Flow
- Conditional Statement
- Recursive Methods
- ▼Java Math and Numbers
- ▼Object Oriented Programming
- Java Constructor
- Java Static Members
- Java Nested Classes
- Java Inheritance
- Java Abstract Classes
- Java Interface
- Java Encapsulation
- Java Polymorphism
- Object-Oriented Programming
- ▼Exception Handling
- Exception Handling Home
- ▼Functional Programming
- Java Lambda expression
- ▼Multithreading
- Java Thread
- Java Multithreading
- ▼Data Structures
- ▼Strings and I/O
- File Input-Output
- ▼Date and Time
- ▼Advanced Concepts
- Java Generic Method
- ▼Algorithms
- ▼Regular Expressions
- Regular Expression Home
- ▼JavaFx Exercises
- JavaFx Exercises Home
- ..More to come..

Java Programming Exercises, Practice, Solution
Java exercises.
Java is the foundation for virtually every type of networked application and is the global standard for developing and delivering embedded and mobile applications, games, Web-based content, and enterprise software. With more than 9 million developers worldwide, Java enables you to efficiently develop, deploy and use exciting applications and services.
The best way we learn anything is by practice and exercise questions. Here you have the opportunity to practice the Java programming language concepts by solving the exercises starting from basic to more complex exercises. A sample solution is provided for each exercise. It is recommended to do these exercises by yourself first before checking the solution.
Hope, these exercises help you to improve your Java programming coding skills. Currently, following sections are available, we are working hard to add more exercises .... Happy Coding!
List of Java Exercises:
- Basic Exercises Part-I [ 150 Exercises with Solution ]
- Basic Exercises Part-II [ 99 Exercises with Solution ]
- Methods [ 23 Exercises with Solution ]
- Data Types Exercises [ 15 Exercises with Solution ]
- Java Enum Types Exercises [ 5 Exercises with Solution ]
- Conditional Statement Exercises [ 32 Exercises with Solution ]
- Java recursive method Exercises [ 15 Exercises with Solution ]
- Math [ 27 Exercises with Solution ]
- Numbers [ 28 Exercises with Solution ]
- Java Constructor Exercises [ 10 exercises with solution ]
- Java Static Members Exercises [ 8 exercises with solution ]
- Java Nested Classes Exercises [ 10 exercises with solution ]
- Java Inheritance Exercises [ 9 exercises with solution ]
- Java Abstract Classes Exercises [ 12 exercises with solution ]
- Java Interface Exercises [ 11 exercises with solution ]
- Java Encapsulation Exercises [ 14 exercises with solution ]
- Java Polymorphism Exercises [ 12 exercises with solution ]
- Object-Oriented Programming [ 30 Exercises with Solution ]
- Exercises on handling and managing exceptions in Java [ 7 Exercises with Solution ]
- Java Lambda expression Exercises [ 25 exercises with solution ]
- Streams [ 8 Exercises with Solution ]
- Java Thread Exercises [ 7 exercises with solution ]
- Java Miltithreading Exercises [ 10 exercises with solution ]
- Array [ 77 Exercises with Solution ]
- Stack [ 29 Exercises with Solution ]
- Collection [ 126 Exercises with Solution ]
- String [ 107 Exercises with Solution ]
- Input-Output-File-System [ 18 Exercises with Solution ]
- Date Time [ 44 Exercises with Solution ]
- Java Generic Methods [ 7 exercises with solution ]
- Java Unit Test [ 10 Exercises with Solution ]
- Search [ 7 Exercises with Solution ]
- Sorting [ 19 Exercises with Solution ]
- Regular Expression [ 30 Exercises with Solution ]
- JavaFX [ 70 Exercises with Solution ]
Note: If you are not habituated with Java programming you can learn from the following :
- Java Programming Language
More to Come !
List of Exercises with Solutions :
- HTML CSS Exercises, Practice, Solution
- JavaScript Exercises, Practice, Solution
- jQuery Exercises, Practice, Solution
- jQuery-UI Exercises, Practice, Solution
- CoffeeScript Exercises, Practice, Solution
- Twitter Bootstrap Exercises, Practice, Solution
- C Programming Exercises, Practice, Solution
- C# Sharp Programming Exercises, Practice, Solution
- PHP Exercises, Practice, Solution
- Python Exercises, Practice, Solution
- R Programming Exercises, Practice, Solution
- Java Exercises, Practice, Solution
- SQL Exercises, Practice, Solution
- MySQL Exercises, Practice, Solution
- PostgreSQL Exercises, Practice, Solution
- SQLite Exercises, Practice, Solution
- MongoDB Exercises, Practice, Solution
[ Want to contribute to Java exercises? Send your code (attached with a .zip file) to us at w3resource[at]yahoo[dot]com. Please avoid copyrighted materials.]
Do not submit any solution of the above exercises at here, if you want to contribute go to the appropriate exercise page.
Follow us on Facebook and Twitter for latest update.
- Weekly Trends and Language Statistics
Methods in Java – Explained with Code Examples
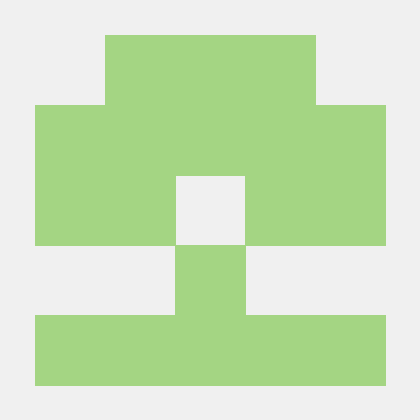
Methods are essential for organizing Java projects, encouraging code reuse, and improving overall code structure.
In this article, we will look at what Java methods are and how they work, including their syntax, types, and examples.
Here's what we'll cover:
What are java methods.
- Types of Access Specifiers in Java – Public ( public ) – Private ( private ) – Protected ( protected ) – Default ( Package-Private )
- Types of Methods – Pre-defined vs. User-defined – Based on functionality
In Java, a method is a set of statements that perform a certain action and are declared within a class.
Here's the fundamental syntax for a Java method:
Let's break down the components:
- accessSpecifier : defines the visibility or accessibility of classes, methods, and fields within a program.
- returnType : the data type of the value that the method returns. If the method does not return any value, the void keyword is used.
- methodName : the name of the method, following Java naming conventions.
- parameter : input value that the method accepts. These are optional, and a method can have zero or more parameters. Each parameter is declared with its data type and a name.
- method body : the set of statements enclosed in curly braces {} that define the task the method performs.
- return statement : if the method has a return type other than void , it must include a return statement followed by the value to be returned.
Here's an example of a simple Java method:
In this example, the addNumbers method takes two integers as parameters ( a and b ), calculates their sum, and returns the result. The main method then calls this method and prints the result.
Compile the Java code using the terminal, using the javac command:

Methods facilitate code reusability by encapsulating functionality in a single block. You can call that block from different parts of your program, avoiding code duplication and promoting maintainability.
Types of Access Specifiers in Java
Access specifiers control the visibility and accessibility of class members (fields, methods, and nested classes).
There are typically four main types of access specifiers: public, private, protected, and default. They dictate where and how these members can be accessed, promoting encapsulation and modularity.
Public ( public )
This grants access to the member from anywhere in your program, regardless of package or class. It's suitable for widely used components like utility functions or constants.
In this example:
- The MyClass class is declared with the public modifier, making it accessible from any other class.
- The publicField is a public field that can be accessed from outside the class.
- The publicMethod() is a public method that can be called from outside the class.
- The main method is the entry point of the program, where an object of MyClass is created, and the public field and method are accessed.
Private ( private )
This confines access to the member within the class where it's declared. It protects sensitive data and enforces encapsulation.
- The MyClass class has a privateField and a privateMethod , both marked with the private modifier.
- The accessPrivateMembers() method is a public method that can be called from outside the class. It provides access to the private field and calls the private method.
Protected ( protected )
The protected access specifier is used to make members (fields and methods) accessible within the same package or by subclasses, regardless of the package. They are not accessible from unrelated classes. It facilitates inheritance while controlling access to specific members in subclasses.
- The Animal class has a protected field ( species ) and a protected method ( makeSound ).
- The Dog class is a subclass of Animal , and it can access the protected members from the superclass.
- The displayInfo() method in the Dog class accesses the protected field and calls the protected method.

With the protected access specifier, members are accessible within the same package and by subclasses, promoting a certain level of visibility and inheritance while still maintaining encapsulation.
Default ( Package-Private )
If no access specifier is used, the default access level is package-private . Members with default access are accessible within the same package, but not outside it. It's often used for utility classes or helper methods within a specific module.
- The Animal class does not have any access modifier specified, making it default (package-private). It has a package-private field species and a package-private method makeSound .
- The Dog class is in the same package as Animal , so it can access the default (package-private) members of the Animal class.
- The Main class runs the program by creating an object of Dog and calling its displayInfo method.
When you run this program, it should output the species and the sound of the animal.
How to Choose the Right Access Specifier
- Public: Use for widely used components, interfaces, and base classes.
- Private: Use for internal implementation details and sensitive data protection.
- Default: Use for helper methods or components specific to a package.
- Protected: Use for shared functionality among subclasses, while restricting access from outside the inheritance hierarchy.
Types of Methods
In Java, methods can be categorized in two main ways:
1. Predefined vs. User-defined:
Predefined methods: These methods are already defined in the Java Class Library and can be used directly without any declaration.
Examples include System.out.println() for printing to the console and Math.max() for finding the maximum of two numbers.
User-defined methods: These are methods that you write yourself to perform specific tasks within your program. They are defined within classes and are typically used to encapsulate functionality and improve code reusability.
- add is a user-defined method because it's created by the user (programmer).
- The method takes two parameters ( num1 and num2 ) and returns their sum.
- The main method calls the add method with specific values, demonstrating the customized functionality provided by the user.
2. Based on functionality:
Within user-defined methods, there are several other classifications based on their characteristics:
Instance Methods:
Associated with an instance of a class. They can access instance variables and are called on an object of the class.
Here are some key characteristics of instance methods:
Access to Instance Variables:
- Instance methods have access to instance variables (also known as fields or properties) of the class.
- They can manipulate the state of the object they belong to.
Use of this Keyword:
- Inside an instance method, the this keyword refers to the current instance of the class. It's often used to differentiate between instance variables and parameters with the same name.
Non-static Context:
- Instance methods are called in the context of an object. They can't be called without creating an instance of the class.
Declaration and Invocation:
- Instance methods are declared without the static keyword.
- They are invoked on an instance of the class using the dot ( . ) notation.
Here's a simple example in Java to illustrate instance methods:
- bark and ageOneYear are instance methods of the Dog class.
- They are invoked on instances of the Dog class ( myDog and anotherDog ).
- These methods can access and manipulate the instance variables ( name and age ) of the respective objects.
Instance methods are powerful because they allow you to encapsulate behavior related to an object's state and provide a way to interact with and modify that state.
Static Methods:
A static method belongs to the class rather than an instance of the class. This means you can call a static method without creating an instance (object) of the class. It's declared using the static keyword.
Static methods are commonly used for utility functions that don't depend on the state of an object. For example, methods for mathematical calculations, string manipulations, and so on.
Abstract Methods:
These methods are declared but not implemented in a class. They are meant to be overridden by subclasses, providing a blueprint for specific functionality that must be implemented in each subclass.
Abstract methods are useful when you want to define a template in a base class or interface, leaving the specific implementation to the subclasses. Abstract methods define a contract that the subclasses must follow.
Other method types: Additionally, there are less common types like constructors used for object initialization, accessor methods (getters) for retrieving object data, and mutator methods (setters) for modifying object data.
In this article, we will look at Java methods, including their syntax, types, and recommended practices.
Happy Coding!
Read more posts .
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Welcome to Java! Easy Max Score: 3 Success Rate: 97.04%
Java stdin and stdout i easy java (basic) max score: 5 success rate: 96.83%, java if-else easy java (basic) max score: 10 success rate: 91.39%, java stdin and stdout ii easy java (basic) max score: 10 success rate: 92.81%, java output formatting easy java (basic) max score: 10 success rate: 96.54%, java loops i easy java (basic) max score: 10 success rate: 97.67%, java loops ii easy java (basic) max score: 10 success rate: 97.32%, java datatypes easy java (basic) max score: 10 success rate: 93.71%, java end-of-file easy java (basic) max score: 10 success rate: 97.91%, java static initializer block easy java (basic) max score: 10 success rate: 96.11%, cookie support is required to access hackerrank.
Seems like cookies are disabled on this browser, please enable them to open this website
Learn to code in Java — a robust programming language used to create software, web and mobile apps, and more.
- AI assistance for guided coding help
- Projects to apply new skills
- Quizzes to test your knowledge
- A certificate of completion
Skill level
Time to complete
Prerequisites
About this course
Popular for its versatility and ability to create a wide variety of applications, learning Java opens up your possibilities when coding. With it, you’ll be able to develop large systems, software, and mobile applications — and even create mobile apps for Android. Learn important Java coding fundamentals and practice your new skills with real-world projects.
Skills you'll gain
Build core programming concepts
Learn object-oriented concepts
Create Java projects
Hello World
Welcome to the world of Java programming! Java is a popular object-oriented programming language that is used in many different industries.
Learn about datatypes in Java and how we use them. Then, practice your skills with two projects where you create and manipulate variables.
Object-Oriented Java
Learn about object-oriented programming in Java. Explore syntax for defining classes and creating instances.
Conditionals and Control Flow
Conditionals and control flow in Java programs.
Arrays and ArrayLists
Build lists of data with Java arrays and ArrayLists.
Use loops to iterate through lists and repeat code.
String Methods
The Java String class provides a lot of useful methods for performing operations on strings and data manipulation.
Certificate of completion available with Plus or Pro
The platform
Hands-on learning

Projects in this course
Planting a tree, java variables: mad libs, earn a certificate of completion.
- Show proof Receive a certificate that demonstrates you've completed a course or path.
- Build a collection The more courses and paths you complete, the more certificates you collect.
- Share with your network Easily add certificates of completion to your LinkedIn profile to share your accomplishments.

Learn Java course ratings and reviews
- 5 stars 59%
- 4 stars 28%
Our learners work at
- Google Logo
- Amazon Logo
- Microsoft Logo
- Reddit Logo
- Spotify Logo
- YouTube Logo
- Instagram Logo
Frequently asked questions about Java
What is java.
Java is an open-source, general-purpose programming language known for its versatility and stability. It’s used for everything from building websites to operating systems and wearable devices. You can even find Java in outer space, running the Mars rover.
What does Java do?
What kind of jobs can java get me, are java and javascript the same, what do i need to know before learning java, join over 50 million learners and start learn java today, looking for something else, related resources, java and the command line, java program structure, java style guide, related courses and paths, study for the ap computer science a exam (java), intro to java, java for programmers, browse more topics.
- Cloud Computing 2,139,528 learners enrolled
- Mobile Development 1,273,387 learners enrolled
- Code Foundations 7,091,015 learners enrolled
- For Business 3,122,858 learners enrolled
- Computer Science 5,539,806 learners enrolled
- Java 1,133,005 learners enrolled
- Web Development 4,738,236 learners enrolled
- Data Science 4,257,826 learners enrolled
- Python 3,441,351 learners enrolled
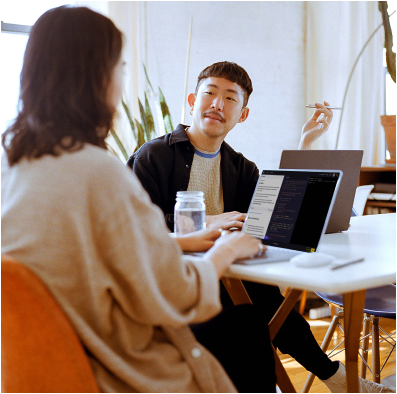
Unlock additional features with a paid plan
Practice projects, assessments, certificate of completion.
The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.
Defining Methods
Here is an example of a typical method declaration:
The only required elements of a method declaration are the method's return type, name, a pair of parentheses, () , and a body between braces, {} .
More generally, method declarations have six components, in order:
- Modifierssuch as public , private , and others you will learn about later.
- The return typethe data type of the value returned by the method, or void if the method does not return a value.
- The method namethe rules for field names apply to method names as well, but the convention is a little different.
- The parameter list in parenthesisa comma-delimited list of input parameters, preceded by their data types, enclosed by parentheses, () . If there are no parameters, you must use empty parentheses.
- An exception listto be discussed later.
- The method body, enclosed between bracesthe method's code, including the declaration of local variables, goes here.
Modifiers, return types, and parameters will be discussed later in this lesson. Exceptions are discussed in a later lesson.
The signature of the method declared above is:
Naming a Method
Although a method name can be any legal identifier, code conventions restrict method names. By convention, method names should be a verb in lowercase or a multi-word name that begins with a verb in lowercase, followed by adjectives, nouns, etc. In multi-word names, the first letter of each of the second and following words should be capitalized. Here are some examples:
Typically, a method has a unique name within its class. However, a method might have the same name as other methods due to method overloading .
Overloading Methods
The Java programming language supports overloading methods, and Java can distinguish between methods with different method signatures . This means that methods within a class can have the same name if they have different parameter lists (there are some qualifications to this that will be discussed in the lesson titled "Interfaces and Inheritance").
Suppose that you have a class that can use calligraphy to draw various types of data (strings, integers, and so on) and that contains a method for drawing each data type. It is cumbersome to use a new name for each methodfor example, drawString , drawInteger , drawFloat , and so on. In the Java programming language, you can use the same name for all the drawing methods but pass a different argument list to each method. Thus, the data drawing class might declare four methods named draw , each of which has a different parameter list.
Overloaded methods are differentiated by the number and the type of the arguments passed into the method. In the code sample, draw(String s) and draw(int i) are distinct and unique methods because they require different argument types.
You cannot declare more than one method with the same name and the same number and type of arguments, because the compiler cannot tell them apart.
The compiler does not consider return type when differentiating methods, so you cannot declare two methods with the same signature even if they have a different return type.
About Oracle | Contact Us | Legal Notices | Terms of Use | Your Privacy Rights
Copyright © 1995, 2022 Oracle and/or its affiliates. All rights reserved.
- Java Course
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
Java Tutorial
This Java Tutorial is designed for beginners as well as experienced professionals. Whether you’re starting your Java journey and looking to understand the basics of Java or its advanced concepts, this free Java tutorial is the perfect resource for you.

What is Java?
Developed by Sun Microsystems in 1995, Java is a highly popular, object-oriented programming language. This platform independent programming language is utilized for Android development, web development, artificial intelligence, cloud applications, and much more.
In this tutorial, we will cover everything from the basics of Java syntax to advanced topics like object-oriented programming and exception handling. So, by the end of this tutorial, you will have a strong understanding of Java and be ready to start writing your own Java applications. So let’s get started on this comprehensive Free Java programming course !
First Java Program
For full explanation of the above Program –
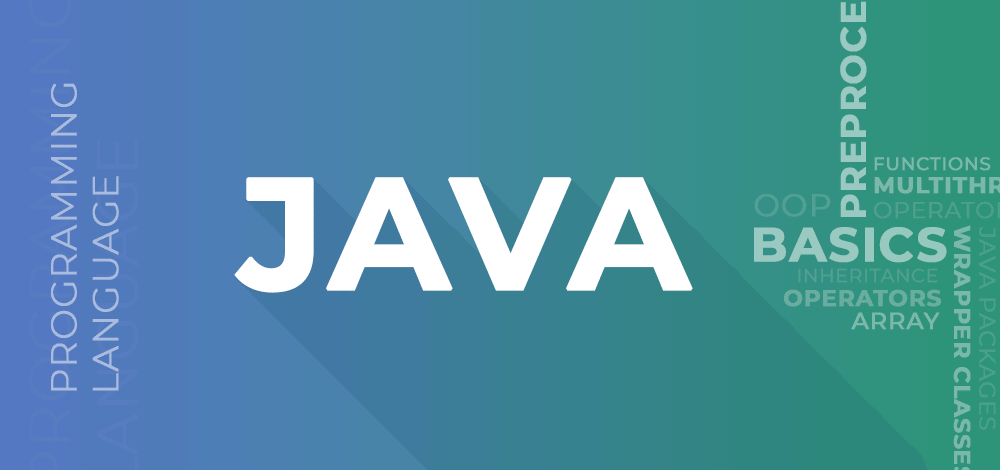
Table of Content
Overview of Java
Basics of java, input/output in java, flow control in java, operators in java, arrays in java, strings in java, oops in java, classes of java, interfaces in java, methods in java, packages in java, collection framework in java, collection classes in java, memory allocation in java, exception handling in java, multithreading in java, synchronization in java, file handling in java, java networking, java se 8 features, java date & time, java miscellaneous, interview questions on java, java practice problems, java jobs & opportunity, get started with free java tutorial.
Here in this section, you will find all the free resources that you need to become zero to mastery in the process of learning Java programming language .
- Introduction to Java
- History of Java
- Java vs C++ vs Python
- How to Download and Install Java?
- Setting Up the Environment in Java
- How to Download and Install Eclipse on Windows?
- Java Development Kit (JDK) in Java
- JVM and its architecture
- JDK Vs JRE Vs JVM
- Just In Time Compiler
- Byte Code Vs Machine Code
- Java Basic Syntax
- First Java Program (Hello World)
- Datatypes in Java
- Primitive Vs Non-Primitive Datatypes
- Java Identifiers
- Java Variables
- Java Keywords
- Scope of Variables
- Wrapper Classes in Java
- How to take Input from users in Java
- Scanner class in Java
- BufferedReader class in Java
- Scanner vs BufferedReader in Java
- Ways to Read Input from Console in Java
- Print Output in Java
- print() Vs println() in Java
- Formatted Outputs in Java
- Decision making in Java
- If Statement in Java
- If-Else Statement in java
- If-Else-If ladder in Java
- Loops in Java
- Do while loop
- For each loop
- Continue Statement in java
- Break Statement In Java
- Usage of Break in Java
- Return Statement in Java
- Arithmetic Operator
- Unary Operator
- Assignment Operator
- Relational Operator
- Logical Operator
- Ternary Operator
- Bitwise Operator
- Introduction to Arrays in Java
- Arrays class in Java
- Multi-Dimensional Array in Java
- How to declare and initialize 2D arrays in Java
- Jagged array in Java
- Final Arrays in Java
- Reflect Arrays in Java
- Java util.Arrays Vs reflect.Arrays
- Java Array Programs
- Introduction of Strings in Java
- String class in Java Set-1 | Set-2
- Why strings are immutable in Java?
- StringBuffer class in Java
- StringBuilder class in Java
- Strings vs StringBuffer vs StringBuilder in Java
- StringTokenizer class in Java Set-1 | Set-2
- StringJoiner in Java
- Java String Programs
OOPS in Java refers to Object-Oriented Programming concepts implemented in the Java programming language. Java’s OOP features include classes, objects, inheritance, polymorphism, and encapsulation, enabling modular and efficient code development. Understanding OOPS in Java is essential for building robust, scalable, and maintainable software applications.
- OOPS Concept in Java
- Why Java is not a purely Object-Oriented Language?
- Classes and Objects
- Naming Convention in Java
- Access Modifiers in Java
- Constructors in Java
- Four pillars of OOPS in Java
- Inheritance in Java
- Abstraction in Java
- Encapsulation in Java
- Polymorphism in Java
- This reference in Java
Classes in Java are the blueprint for creating objects and defining their properties and behaviors. They are the fundamental building blocks of Java programs, enabling the creation of reusable and modular code. Classes can contain fields, methods, constructors, and nested classes, providing a wide range of functionality and flexibility.
- Understanding classes and objects in Java
- Class vs interface
- Singleton class in java
- Object class in java
- Inner class in java
- Abstract classes in java
- Throwable class in java
Interfaces in Java are abstract types that define a set of methods that a class must implement. They provide a way to achieve abstraction, multiple inheritance, and loose coupling in Java programs. Interfaces are useful for defining common behaviors and functionalities that can be shared across different classes, leading to more modular and reusable code. Mastering interfaces in Java is crucial for building flexible and extensible software applications.
- Java Interfaces
- Interfaces and Inheritance in Java
- Class Vs Interface in Java
- Functional Interface
- Nested Interface
- Marker Interface
- Comparator Interface
- Introduction to methods in Java
- Different method calls in Java
- Static methods Vs Instance methods in Java
- Abstract methods in Java
- Method Overriding in Java
- Method Overloading in Java
- Method Overloading Vs Method Overriding
- Java Packages
- How to create a package in Java
- java.util package
- java.lang package
- java.io package
- Java Collection Framework
- Collections class in Java
- Collection Interface in Java
- List Interface in Java
- Queue Interface in Java
- Map Interface in Java
- Set Interface in Java
- SortedSet Interface in Java
- Deque Interface in Java
- Comparator in Java
- Comparator Vs Comparable in Java
- Iterator in Java
- ArrayList in Java
- Vector class in Java
- Stack class in Java
- LinkedList in Java
- Priority Queue in Java
- HashMap in Java
- LinkedHashMap in Java
- Dictionary in Java
- HashTable in Java
- HashSet in Java
- TreeSet in Java
- LinkedHashSet in Java
- Java Memory Management
- How are Java objects stored in memory
- Stack vs Heap memory allocation
- Types of memory areas allocated by JVM
- Garbage Collection in Java
- Heap and Stack memory allocation
- Types of JVM Garbage Collectors in Java
- Memory leaks in Java
- Java Virtual Machine(JVM) Stack Area
- Exceptions in java
- Types of Exceptions
- Checked Vs Unchecked Exceptions
- Try, Catch, Finally, throw, and throws
- Flow control in Try catch block
- Throw vs Throws
- Final vs Finally vs Finalize
- User-defined custom exception
- Chained Exceptions
- Null pointer Exceptions
- Exception handling with method Overriding
- Introduction to Multithreading in Java
- Lifecycle and Stages of a Thread
- Thread Priority in Java
- Main Thread in Java
- Thread class
- Runnable interface
- How to name a thread
- start() method in thread
- run() vs start() Method in Java
- sleep() method
- Daemon thread
- Thread Pool in Java
- Thread Group in Java
- Thread Safety in Java
- ShutdownHook
- Multithreading Tutorial
- Java Synchronization
- Importance of Thread synchronization in Java
- Method and Block Synchronization in Java
- Local frameworks vs thread synchronization
- Atomic Vs Volatile in Java
- Atomic Vs Synchronized in Java
- Deadlock in Multithreading
- Deadlock Prevention and Avoidance
- Lock Vs Monitor in Concurrency
- Reentrant Lock
- File Class in java
- How to create files in java
- How to read files in java
- How to write on files in java
- How to delete a file in java
- File Permissions
- File Writer
- FileDescriptor class
- RandomAccessFile class
- Introduction to Java Regex
- How to write Regex expressions
- Matcher class
- Pattern class
- Quantifiers
- Character class
- Introduction to Java IO
- Reader Class
- Writer Class
- FileInput stream
- File Output stream
- BufferedReader Input Stream
- BufferedReader Output stream
- BufferedReader vs Scanner
- Fast I/O in Java
- Introduction to Java Networking
- TCP architecture
- UDP architecture
- IPV4 Vs IPV6
- Connection-oriented Vs connectionless protocols
- Socket programming in Java
- Server Socket class
- URL class and methods
- Lambda Expressions
- Streams API
- New Date/Time API
- Default Methods
- Functional Interfaces
- Method references
- Optional class
- Stream Filter
- Type Annotations
- String Joiner
- Date Class in Java
- Methods of the Date class
- Java Current Date and time
- Compare dates in Java
- Introduction to Java JDBC
- JDBC Driver
- JDBC Connection
- Types of Statements in JDBC
- JDBC Tutorial
- Introduction to Reflection API
- Java IO Tutorial
- JavaFX Tutorial
- How to Run Java RMI application?
- Java 17 New Features
- Core Java Interview Questions
- Java Multiple Choice Questions
Features of Java
- Java has been one of the most popular programming languages for many years.
- Java is Object Oriented. However, it is not considered as pure object-oriented as it provides support for primitive data types (like int, char, etc)
- The Java codes are first compiled into byte code (machine-independent code). Then the byte code runs on Java Virtual Machine (JVM) regardless of the underlying architecture.
- Java syntax is similar to C/C++. But Java does not provide low-level programming functionalities like pointers. Also, Java codes are always written in the form of classes and objects.
- Java is used in all kinds of applications like Mobile Applications (Android is Java-based), desktop applications, web applications, client-server applications, enterprise applications, and many more.
- When compared with C++, Java codes are generally more maintainable because Java does not allow many things which may lead to bad/inefficient programming if used incorrectly. For example, non-primitives are always references in Java. So we cannot pass large objects (like we can do in C++) to functions, we always pass references in Java. One more example, since there are no pointers, bad memory access is also not possible.
- When compared with Python, Java kind of fits between C++ and Python. The programs are written in Java typically run faster than corresponding Python programs and slower than C++. Like C++, Java does static type checking, but Python does not.
Applications of Java
Here in this section, we have added some of the applications that were developed using the Java programming language.
- Mobile Applications
- Desktop GUI Applications
- Artificial intelligence
- Scientific Applications
- Cloud Applications
- Embedded Systems
- Gaming Applications
Keep up your pace and try hard till you excel in it. This will need some motivation and resources to Practice Java. So, there are few resources mentioned below this will help you in your journey:
Java Interview Questions and Answers Java Programming Examples Java Exercises – Basic to Advanced Java Practice Programs with Solutions Java Language MCQs with Answers Java Practice Quiz
According to report of Statista.com Java is most demanded programming languages after JavaScript by recruiters worldwide in 2024. Major MNC companies are recruiting Java Programmers.
- Deloitte India
- Many more…
Java Latest & Upcoming Features
Java SE 21 Released: September 2023 Java SE 21 is the latest stable release, featuring:
- Hidden Classes (Preview): Introduces a mechanism to restrict access to specific members of a class, enhancing modularity and code maintainability.
- Improved Garbage Collector Ergonomics (JEP 429): Simplifies configuration and monitoring of the garbage collection process.
- API Updates : Enhancements to existing APIs, including java.io.file for better file handling capabilities.
Java SE 22 Released: March 19, 2024 Java SE 22 introduces:
- Foreign Function & Memory API (JEP 454): Enhances interoperability with native code.
- Unnamed Variables & Patterns (JEP 456): Adds support for unnamed variables and patterns.
- Structured Concurrency (JEP 462, Second Preview): Simplifies multithreaded programming by structuring concurrency.
- String Templates (JEP 459, Second Preview): Provides a new syntax for creating and manipulating strings.
- Vector API (JEP 460, Seventh Incubator): Offers a portable and low-level abstraction for SIMD programming.
Upcoming Features (Expected in Java SE 23 – September 2024)
- Vector API (Incubator): A new API designed for high-performance vector computations, potentially beneficial for scientific computing and machine learning applications.
- Project Panama (Preview): Aims to improve interoperability between Java and native code, simplifying interactions with non-Java libraries and frameworks.
Trends in Java Development:
- Java continues to prioritize features that enhance application performance and developer experience.
- Java’s strong position in cloud environments is expected to hold as cloud computing remains a dominant trend.
- Java’s suitability for building scalable and modular systems aligns well with the popularity of microservices architectures.
- Expect to see Java evolving to integrate more seamlessly with technologies like artificial intelligence, big data, and the Internet of Things (IoT).
Java Programming Tutorial: FAQs
Why use java .
Java is simple to understand programming language because doesn’t contain concepts like : Pointers and operator overloading and it is secure and portable.
What are the major concepts in Java?
There are 4 major concept in Java that is abstraction, encapsulation, polymorphism, and inheritance. Along with this Java also works with three OOPs concept
How Java different to C++ ?
C++ | JAVA |
---|---|
C++ is platform dependent. | Java is platform independent. |
C++ uses compiler only. | Java uses compiler and interpreter both. |
C++ support pointers and operator overloading. | Java doesn’t support pointers and operator overloading concept. |
C++ not support multithreading concept. | Java supports multithreading concept. |
Why Java is so popular programming language?
Java is based on object model hence it is one the popular programming language.
What are the scope of Java Technologies for Web Applications?
Java Technologies for Web Applications are a set of Java-based technologies that are used to develop web applications. These technologies include: Java Servlet API JavaServer Pages JavaServer Faces Enterprise JavaBeans JDBC (Java Database Connectivity) Java Messaging Service (JMS): JavaMail API: JAX-WS
Check More Resources Related to Java Programming
Please login to comment..., similar reads.
- How to Delete Discord Servers: Step by Step Guide
- Google increases YouTube Premium price in India: Check our the latest plans
- California Lawmakers Pass Bill to Limit AI Replicas
- Best 10 IPTV Service Providers in Germany
- 15 Most Important Aptitude Topics For Placements [2024]
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Method within a method (homework assignment)
I have a homework assignment where I am asked to:
Write a method sort(int[] arr) that takes an integer array and uses a method from a previous exercise to determine if the array is sorted in increasing order or not. If it is already sorted, it should return the array, if it is not, the method should sort the array before returning it.
My problem is that I don't know how I should approach this assignment? Should I modify the previous method, call it within a new method or can I create a method within the method? This is the previous method that I wrote:
Thanx in advance
- 8 You can't declare methods within methods. Your assignment is telling you to use a method from a previous exercise to check if the array is sorted in increasing order or not. Use it. – proulxs Commented Sep 30, 2014 at 19:17
- public void bar() { foo(); } I call the foo() method from my bar() method. Same concept as your assignment. – Compass Commented Sep 30, 2014 at 19:19
3 Answers 3
In the interest of you actually learning something, here's my solution:
Here is the documentation for Arrays.sort
Not only is this more concise, it also does the sort in-place. This means that instead of copying the array you pass in, doing the work, and then returning the copy, it just does the work on the original array. This makes it slightly faster and means it takes up a minimal amount of extra space. Not that big a deal when your input size is 10, but it is when you get to 10 million. Also, note the wording of the assignment: If it is already sorted, it should return the array, if it is not, the method should sort the array before returning it. Technically, returning a copy of the original array is not what the assignment wants. It wants the original.
So it's short, clean, fast, and memory efficient. It must be good, right?
No, because it has one huge flaw. It breaks the implied contract of the method. A function that changes its arguments should not return anything, and a function that returns something should not modify its arguments. This is a basic principle of software engineering (and one that Arrays.sort demonstrates). Violating this can cause huge problems for those who assume you're competent and won't break this rule.
If we follow the rule, sort should be this:
So tell your teacher an armored hamster you met on the Internet said to stop teaching you poor interface design.

- Thank you very much! This seems like a better approach. I will definitely tell him that haha. – Morten Falk Commented Oct 1, 2014 at 7:14
I read "uses [the] method" to mean "calls the method". That said, testing for sortedness before sorting seems somewhat pointless, so it might be worth double-checking with whoever gave you that assignment.
One issue with your previous method is that it doesn't return anything. You'll need to do something about that to make it useful.
- 1 There are certain sorting algorithms that perform very poorly on sorted data, so doing a sanity check beforehand is reasonable. – Floegipoky Commented Sep 30, 2014 at 19:25
- True. In the actual assignment I am invoking the method and printing the result. – Morten Falk Commented Sep 30, 2014 at 19:26
- @Floegipoky: Point taken. And, again, it's only an exercise after all. – NPE Commented Sep 30, 2014 at 19:28
- I think the point is just learning how to do it regardless of it being pointless. So what should I do in practice? I know how to use the java.util.Arrays.sort method but my problem is combining the method that I already wrote with a new method that returns the array or sorts it... – Morten Falk Commented Sep 30, 2014 at 19:30
This is what you want to do:
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged java or ask your own question .
- The Overflow Blog
- At scale, anything that could fail definitely will
- Best practices for cost-efficient Kafka clusters
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Feedback requested: How do you use tag hover descriptions for curating and do...
- Staging Ground Reviewer Motivation
Hot Network Questions
- Applying for different jobs finding out it is for the same project between different companies
- Nearly stalled on takeoff after just 3 hours training on a PPL. Is this normal?
- Why doesn’t dust interfere with the adhesion of geckos’ feet?
- Can a British judge convict and sentence someone for contempt in their own court on the spot?
- Can you fix this error while creating alias for encryption and decryption by GPG in z-shell?
- Calculating area of intersection of two segmented polygons in QGIS
- How do I safely download and run an older version of software for testing without interfering with the currently installed version?
- How would humans actually colonize mars?
- Does an unseen creature with a burrow speed have advantage when attacking from underground?
- Not a cross, not a word (number crossword)
- In what instances are 3-D charts appropriate?
- What rules of legal ethics apply to information a lawyer learns during a consultation?
- Can a quadrilateral polygon have 3 obtuse angles?
- What is the difference between negation-eliminiation ¬E and contradiction-introduction ⊥I?
- Sylvester primes
- RS485 constant chip failure
- Why is there so much salt in cheese?
- Whats the safest way to store a password in database?
- Are all citizens of Saudi Arabia "considered Muslims by the state"?
- Asked to suggest referees 9 months after submission: what to do?
- Invest smaller lump sum vs investing (larger) monthly amount
- Second Derivative for Non standard Calculus
- Creating Layout of 2D Board game
- Geometry nodes: Curve caps horizontal
Java Tutorial
Java methods, java classes, java file handling, java how to's, java reference, java examples.
Java is a popular programming language.
Java is used to develop mobile apps, web apps, desktop apps, games and much more.
Examples in Each Chapter
Our "Try it Yourself" editor makes it easy to learn Java. You can edit Java code and view the result in your browser.
Try it Yourself »
Click on the "Run example" button to see how it works.
We recommend reading this tutorial, in the sequence listed in the left menu.
Java is an object oriented language and some concepts may be new. Take breaks when needed, and go over the examples as many times as needed.
Java Exercises
Test yourself with exercises.
Insert the missing part of the code below to output "Hello World".
Start the Exercise
Advertisement
Test your Java skills with a quiz.
Start Java Quiz
Learn by Examples
Learn by examples! This tutorial supplements all explanations with clarifying examples.
See All Java Examples
My Learning
Track your progress with the free "My Learning" program here at W3Schools.
Log in to your account, and start earning points!
This is an optional feature. You can study at W3Schools without using My Learning.

You will also find complete keyword and method references:
Java Keywords
Java String Methods
Java Math Methods
Java Output Methods
Java Arrays Methods
Java ArrayList Methods
Java LinkedList Methods
Java HashMap Methods
Java Errors and Exception Types
Download Java
Download Java from the official Java web site: https://www.oracle.com
Java Exam - Get Your Diploma!
Kickstart your career.
Get certified by completing the course

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Learn Java practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn java interactively.
Java Examples
The best way to learn Java programming is by practicing examples. The page contains examples on basic concepts of Java. You are advised to take the references from these examples and try them on your own.
All the programs on this page are tested and should work on all platforms.
Want to learn Java by writing code yourself? Enroll in our Interactive Java Course for FREE.
- Java Program to Check Prime Number
- Java Program to Display Fibonacci Series
- Java Program to Create Pyramids and Patterns
- Java Program to Reverse a Number
- Java Program to Print an Integer (Entered by the User)
- Java Program to Add Two Integers
- Java Program to Multiply two Floating Point Numbers
- Java Program to Find ASCII Value of a character
- Java Program to Compute Quotient and Remainder
- Java Program to Swap Two Numbers
- Java Program to Check Whether a Number is Even or Odd
- Java Program to Check Whether an Alphabet is Vowel or Consonant
- Java Program to Find the Largest Among Three Numbers
- Java Program to Find all Roots of a Quadratic Equation
- Java Program to Check Leap Year
- Java Program to Check Whether a Number is Positive or Negative
- Java Program to Check Whether a Character is Alphabet or Not
- Java Program to Calculate the Sum of Natural Numbers
- Java Program to Find Factorial of a Number
- Java Program to Generate Multiplication Table
- Java Program to Find GCD of two Numbers
- Java Program to Find LCM of two Numbers
- Java Program to Display Alphabets (A to Z) using loop
- Java Program to Count Number of Digits in an Integer
- Java Program to Calculate the Power of a Number
- Java Program to Check Palindrome
- Java Program to Check Whether a Number is Prime or Not
- Java Program to Display Prime Numbers Between Two Intervals
- Java Program to Check Armstrong Number
- Java Program to Display Armstrong Number Between Two Intervals
- Java Program to Display Prime Numbers Between Intervals Using Function
- Java Program to Display Armstrong Numbers Between Intervals Using Function
- Java Program to Display Factors of a Number
- Java Program to Make a Simple Calculator Using switch...case
- Java Program to Check Whether a Number can be Expressed as Sum of Two Prime Numbers
- Java Program to Find the Sum of Natural Numbers using Recursion
- Java Program to Find Factorial of a Number Using Recursion
- Java Program to Find G.C.D Using Recursion
- Java Program to Convert Binary Number to Decimal and vice-versa
- Java Program to Convert Octal Number to Decimal and vice-versa
- Java Program to Convert Binary Number to Octal and vice-versa
- Java Program to Reverse a Sentence Using Recursion
- Java Program to calculate the power using recursion
- Java Program to Calculate Average Using Arrays
- Java Program to Find Largest Element of an Array
- Java Program to Calculate Standard Deviation
- Java Program to Add Two Matrix Using Multi-dimensional Arrays
- Java Program to Multiply Two Matrix Using Multi-dimensional Arrays
- Java Program to Multiply two Matrices by Passing Matrix to a Function
- Java Program to Find Transpose of a Matrix
- Java Program to Find the Frequency of Character in a String
- Java Program to Count the Number of Vowels and Consonants in a Sentence
- Java Program to Sort Elements in Lexicographical Order (Dictionary Order)
- Java Program to Add Two Complex Numbers by Passing Class to a Function
- Java Program to Calculate Difference Between Two Time Periods
- Java Code To Create Pyramid and Pattern
- Java Program to Remove All Whitespaces from a String
- Java Program to Print an Array
- Java Program to Convert String to Date
- Java Program to Round a Number to n Decimal Places
- Java Program to Concatenate Two Arrays
- Java Program to Convert Character to String and Vice-Versa
- Java Program to Check if An Array Contains a Given Value
- Java Program to Check if a String is Empty or Null
- Java Program to Get Current Date/Time
- Java Program to Convert Milliseconds to Minutes and Seconds
- Java Program to Add Two Dates
- Java Program to Join Two Lists
- Java Program to Convert a List to Array and Vice Versa
- Java Program to Get Current Working Directory
- Java Program to Convert Map (HashMap) to List
- Java Program to Convert Array to Set (HashSet) and Vice-Versa
- Java Program to Convert Byte Array to Hexadecimal
- Java Program to Create String from Contents of a File
- Java Program to Append Text to an Existing File
- Java Program to Convert a Stack Trace to a String
- Java Program to Convert File to byte array and Vice-Versa
- Java Program to Convert InputStream to String
- Java Program to Convert OutputStream to String
- Java Program to Lookup enum by String value
- Java Program to Compare Strings
- Java Program to Sort a Map By Values
- Java Program to Sort ArrayList of Custom Objects By Property
- Java Program to Check if a String is Numeric
- Java Program to convert char type variables to int
- Java Program to convert int type variables to char
- Java Program to convert long type variables into int
- Java Program to convert int type variables to long
- Java Program to convert boolean variables into string
- Java Program to convert string type variables into boolean
- Java Program to convert string type variables into int
- Java Program to convert int type variables to String
- Java Program to convert int type variables to double
- Java Program to convert double type variables to int
- Java Program to convert string variables to double
- Java Program to convert double type variables to string
- Java Program to convert primitive types to objects and vice versa
- Java Program to Implement Bubble Sort algorithm
- Java Program to Implement Quick Sort Algorithm
- Java Program to Implement Merge Sort Algorithm
- Java Program to Implement Binary Search Algorithm
- Java Program to Call One Constructor from another
- Java Program to implement private constructors
- Java Program to pass lambda expression as a method argument
- Java Program to Pass the Result of One Method Call to Another Method
- Java Program to Calculate the Execution Time of Methods
- Java Program to Convert a String into the InputStream
- Java Program to Convert the InputStream into Byte Array
- Java Program to Load File as InputStream
- Java Program to Create File and Write to the File
- Java Program to Read the Content of a File Line by Line
- Java Program to Delete File in Java
- Java Program to Delete Empty and Non-empty Directory
- Java Program to Get the File Extension
- Java Program to Get the name of the file from the absolute path
- Java Program to Get the relative path from two absolute paths
- Java Program to Count number of lines present in the file
- Java Program to Determine the class of an object
- Java Program to Create an enum class
- Java Program to Print object of a class
- Java Program to Create custom exception
- Java Program to Create an Immutable Class
- Java Program to Check if two strings are anagram
- Java Program to Compute all the permutations of the string
- Java Program to Create random strings
- Java Program to Clear the StringBuffer
- Java Program to Capitalize the first character of each word in a String
- Java Program to Iterate through each characters of the string.
- Java Program to Differentiate String == operator and equals() method
- Java Program to Implement switch statement on strings
- Java Program to Calculate simple interest and compound interest
- Java Program to Implement multiple inheritance
- Java Program to Determine the name and version of the operating system
- Java Program to Check if two of three boolean variables are true
- Java Program to Iterate over enum
- Java Program to Check the birthday and print Happy Birthday message
- Java Program to Implement LinkedList
- Java Program to Implement stack data structure
- Java Program to Implement the queue data structure
- Java Program to Get the middle element of LinkedList in a single iteration
- Java Program to Convert the LinkedList into an Array and vice versa
- Java Program to Convert the ArrayList into a string and vice versa
- Java Program to Iterate over an ArrayList
- Java Program to Iterate over a HashMap
- Java Program to Iterate over a Set
- Java Program to Merge two lists
- Java Program to Update value of HashMap using key
- Java Program to Remove duplicate elements from ArrayList
- Java Program to Get key from HashMap using the value
- Java Program to Detect loop in a LinkedList
- Java Program to Calculate union of two sets
- Java Program to Calculate the intersection of two sets
- Java Program to Calculate the difference between two sets
- Java Program to Check if a set is the subset of another set
- Java Program to Sort map by keys
- Java Program to Pass ArrayList as the function argument
- Java Program to Iterate over ArrayList using Lambda Expression
- Java Program to Implement Binary Tree Data Structure
- Java Program to Perform the preorder tree traversal
- Java Program to Perform the postorder tree traversal
- Java Program to Perform the inorder tree traversal
- Java Program to Count number of leaf nodes in a tree
- Java Program to Check if a string contains a substring
- Java Program to Access private members of a class
- Java Program to Check if a string is a valid shuffle of two distinct strings
- Java Program to Implement the graph data structure
- Java Program to Remove elements from the LinkedList.
- Java Program to Add elements to a LinkedList
- Java Program to Access elements from a LinkedList.

IMAGES
VIDEO
COMMENTS
Write a Java method to display the factors of 3 in a given integer. Expected Output: Input an integer (positive/negative): 81 Factors of 3 of the said integer: 81 = 3 * 3 * 3 * 3 * 1. Click me to see the solution. 22. Write a Java method to check whether every digit of a given integer is even.
Java is a popular programming language that can be used to create various types of applications, such as desktop, web, enterprise, and mobile. Java is also an object-oriented language, which means that it organizes data and behaviour into reusable units called classes and objects. Java is known for its portability, performance, security, and robust
The best way we learn anything is by practice and exercise questions. Here you have the opportunity to practice the Java programming language concepts by solving the exercises starting from basic to more complex exercises. A sample solution is provided for each exercise. It is recommended to do these exercises by yourself first before checking ...
Create a method that takes two integers as arguments and returns their sum. Examples SumOfTwoNumbers(3, 2) 5 SumOfTwoNumbers(-3, -6) -9 SumOfTwoNumbers(7, 3) 10 Notes Don't forget to return the result. ... Practice Java coding with fun, bite-sized exercises. Earn XP, unlock achievements and level up. It's like Duolingo for learning to code.
Get certified by completing the JAVA course. Track your progress - it's free! Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Declaring a Java Method. The syntax to declare a method is: returnType methodName() { // method body } Here, returnType - It specifies what type of value a method returns For example if a method has an int return type then it returns an integer value. If the method does not return a value, its return type is void.; methodName - It is an identifier that is used to refer to the particular method ...
Java Methods. In Java, the word method refers to the same kind of thing that the word function is used for in other languages. Speci cally, a method is a function that belongs to a class. In Java, every function belongs to a class. A function is a reusable portion of a program, sometimes called a procedure or subroutine. It is like a mini ...
Types of Methods. In Java, methods can be categorized in two main ways: 1. Predefined vs. User-defined: Predefined methods: These methods are already defined in the Java Class Library and can be used directly without any declaration. Examples include System.out.println() for printing to the console and Math.max() for finding the maximum of two ...
Java Stdin and Stdout I. Easy Java (Basic) Max Score: 5 Success Rate: 96.83%. Solve Challenge. Java If-Else. Easy Java (Basic) Max Score: 10 Success Rate: 91.39%. Solve Challenge. Java Stdin and Stdout II. Easy Java (Basic) Max Score: 10 Success Rate: 92.81%. Solve Challenge. Java Output Formatting.
Learn Java Programming. Java is a platform-independent language that runs on 3 billion devices worldwide. It is widely used in enterprise applications, android development, big data, and legacy software, where reliability and security are crucial. You can often find universities teaching Java as a part of the curriculum as it focuses on writing ...
Popular for its versatility and ability to create a wide variety of applications, learning Java opens up your possibilities when coding. With it, you'll be able to develop large systems, software, and mobile applications — and even create mobile apps for Android. Learn important Java coding fundamentals and practice your new skills with ...
Defining Methods. Here is an example of a typical method declaration: double length, double grossTons) {. //do the calculation here. The only required elements of a method declaration are the method's return type, name, a pair of parentheses, (), and a body between braces, {}. More generally, method declarations have six components, in order:
The method in Java or Methods of Java is a collection of statements that perform some specific tasks and return the result to the caller. A Java method can perform some specific tasks without returning anything. Java Methods allows us to reuse the code without retyping the code. In Java, every method must be part of some class that is different from languages like C, C++, and Python.
Java Hello World Program. A "Hello, World!" is a simple program that outputs Hello, World! on the screen. Since it's a very simple program, it's often used to introduce a new programming language to a newbie. Let's explore how Java "Hello, World!" program works. Note: You can use our online Java compiler to run Java programs.
Now, with expert-verified solutions from Java Methods: Object-Oriented Programming and Data Structures 3rd Edition, you'll learn how to solve your toughest homework problems. Our resource for Java Methods: Object-Oriented Programming and Data Structures includes answers to chapter exercises, as well as detailed information to walk you through ...
java.lang.Long.highestOneBit() is a built-in method in Java which first convert the number to Binary, then it looks for the first set bit from the left, then it reset rest of the bits and then returns the value. In simple language, if the binary expression of a number contains a minimum of a single set bit, it returns 2^(last set bit position from
Understanding Java Methods 3rd Edition homework has never been easier than with Chegg Study. Why is Chegg Study better than downloaded Java Methods 3rd Edition PDF solution manuals? It's easier to figure out tough problems faster using Chegg Study. Unlike static PDF Java Methods 3rd Edition solution manuals or printed answer keys, our experts ...
I have a homework assignment where I am asked to: Write a method sort(int[] arr) that takes an integer array and uses a method from a previous exercise to determine if the array is sorted in increasing order or not. If it is already sorted, it should return the array, if it is not, the method should sort the array before returning it.
Example Explained. myMethod() is the name of the method static means that the method belongs to the Main class and not an object of the Main class. You will learn more about objects and how to access methods through objects later in this tutorial. void means that this method does not have a return value. You will learn more about return values later in this chapter
Step-by-step solution. Step 1 of 14. Variable. The term variable refers to the memory location of the value associated with it. The data is held by the variable. The variables also refer to the containers. The variable's names make the code more meaningful. • Each variable name has a data type. • If the variable is of the same data type ...
Java Methods Textbook Solutions. Select the Edition for Java Methods Below: Edition Name HW Solutions Java Methods 3rd Edition by Maria Litvin, Gary Litvin: 274: Join Chegg Study and get: Guided textbook solutions created by Chegg experts Learn from step-by-step solutions for over 34,000 ISBNs in Math, Science, Engineering, Business and more ...
Example Get your own Java Server. Click on the "Run example" button to see how it works. We recommend reading this tutorial, in the sequence listed in the left menu. Java is an object oriented language and some concepts may be new. Take breaks when needed, and go over the examples as many times as needed.
Java Program to Get the name of the file from the absolute path. Java Program to Get the relative path from two absolute paths. Java Program to Count number of lines present in the file. Java Program to Determine the class of an object. Java Program to Create an enum class. Java Program to Print object of a class.