- Skip to main content
- Select language
- Skip to search
- Destructuring assignment

Unpacking values from a regular expression match
Es2015 version, invalid javascript identifier as a property name.
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables.
Description
The object and array literal expressions provide an easy way to create ad hoc packages of data.
The destructuring assignment uses similar syntax, but on the left-hand side of the assignment to define what values to unpack from the sourced variable.
This capability is similar to features present in languages such as Perl and Python.
Array destructuring
Basic variable assignment, assignment separate from declaration.
A variable can be assigned its value via destructuring separate from the variable's declaration.
Default values
A variable can be assigned a default, in the case that the value unpacked from the array is undefined .
Swapping variables
Two variables values can be swapped in one destructuring expression.
Without destructuring assignment, swapping two values requires a temporary variable (or, in some low-level languages, the XOR-swap trick ).
Parsing an array returned from a function
It's always been possible to return an array from a function. Destructuring can make working with an array return value more concise.
In this example, f() returns the values [1, 2] as its output, which can be parsed in a single line with destructuring.
Ignoring some returned values
You can ignore return values that you're not interested in:
You can also ignore all returned values:
Assigning the rest of an array to a variable
When destructuring an array, you can unpack and assign the remaining part of it to a variable using the rest pattern:
Note that a SyntaxError will be thrown if a trailing comma is used on the left-hand side with a rest element:
When the regular expression exec() method finds a match, it returns an array containing first the entire matched portion of the string and then the portions of the string that matched each parenthesized group in the regular expression. Destructuring assignment allows you to unpack the parts out of this array easily, ignoring the full match if it is not needed.
Object destructuring
Basic assignment, assignment without declaration.
A variable can be assigned its value with destructuring separate from its declaration.
The ( .. ) around the assignment statement is required syntax when using object literal destructuring assignment without a declaration.
{a, b} = {a: 1, b: 2} is not valid stand-alone syntax, as the {a, b} on the left-hand side is considered a block and not an object literal.
However, ({a, b} = {a: 1, b: 2}) is valid, as is var {a, b} = {a: 1, b: 2}
NOTE: Your ( ..) expression needs to be preceded by a semicolon or it may be used to execute a function on the previous line.
Assigning to new variable names
A property can be unpacked from an object and assigned to a variable with a different name than the object property.
A variable can be assigned a default, in the case that the value unpacked from the object is undefined .
Setting a function parameter's default value
Es5 version, nested object and array destructuring, for of iteration and destructuring, unpacking fields from objects passed as function parameter.
This unpacks the id , displayName and firstName from the user object and prints them.
Computed object property names and destructuring
Computed property names, like on object literals , can be used with destructuring.
Rest in Object Destructuring
The Rest/Spread Properties for ECMAScript proposal (stage 3) adds the rest syntax to destructuring. Rest properties collect the remaining own enumerable property keys that are not already picked off by the destructuring pattern.
Destructuring can be used with property names that are not valid JavaScript identifiers by providing an alternative identifer that is valid.
Specifications
Browser compatibility.
[1] Requires "Enable experimental Javascript features" to be enabled under `about:flags`
Firefox-specific notes
- Firefox provided a non-standard language extension in JS1.7 for destructuring. This extension has been removed in Gecko 40 (Firefox 40 / Thunderbird 40 / SeaMonkey 2.37). See bug 1083498 .
- Starting with Gecko 41 (Firefox 41 / Thunderbird 41 / SeaMonkey 2.38) and to comply with the ES2015 specification, parenthesized destructuring patterns, like ([a, b]) = [1, 2] or ({a, b}) = { a: 1, b: 2 } , are now considered invalid and will throw a SyntaxError . See Jeff Walden's blog post and bug 1146136 for more details.
- Assignment operators
- "ES6 in Depth: Destructuring" on hacks.mozilla.org
Destructuring assignment
The two most used data structures in JavaScript are Object and Array .
- Objects allow us to create a single entity that stores data items by key.
- Arrays allow us to gather data items into an ordered list.
However, when we pass these to a function, we may not need all of it. The function might only require certain elements or properties.
Destructuring assignment is a special syntax that allows us to “unpack” arrays or objects into a bunch of variables, as sometimes that’s more convenient.
Destructuring also works well with complex functions that have a lot of parameters, default values, and so on. Soon we’ll see that.
Array destructuring
Here’s an example of how an array is destructured into variables:
Now we can work with variables instead of array members.
It looks great when combined with split or other array-returning methods:
As you can see, the syntax is simple. There are several peculiar details though. Let’s see more examples to understand it better.
It’s called “destructuring assignment,” because it “destructurizes” by copying items into variables. However, the array itself is not modified.
It’s just a shorter way to write:
Unwanted elements of the array can also be thrown away via an extra comma:
In the code above, the second element of the array is skipped, the third one is assigned to title , and the rest of the array items are also skipped (as there are no variables for them).
…Actually, we can use it with any iterable, not only arrays:
That works, because internally a destructuring assignment works by iterating over the right value. It’s a kind of syntax sugar for calling for..of over the value to the right of = and assigning the values.
We can use any “assignables” on the left side.
For instance, an object property:
In the previous chapter, we saw the Object.entries(obj) method.
We can use it with destructuring to loop over the keys-and-values of an object:
The similar code for a Map is simpler, as it’s iterable:
There’s a well-known trick for swapping values of two variables using a destructuring assignment:
Here we create a temporary array of two variables and immediately destructure it in swapped order.
We can swap more than two variables this way.
The rest ‘…’
Usually, if the array is longer than the list at the left, the “extra” items are omitted.
For example, here only two items are taken, and the rest is just ignored:
If we’d like also to gather all that follows – we can add one more parameter that gets “the rest” using three dots "..." :
The value of rest is the array of the remaining array elements.
We can use any other variable name in place of rest , just make sure it has three dots before it and goes last in the destructuring assignment.
Default values
If the array is shorter than the list of variables on the left, there will be no errors. Absent values are considered undefined:
If we want a “default” value to replace the missing one, we can provide it using = :
Default values can be more complex expressions or even function calls. They are evaluated only if the value is not provided.
For instance, here we use the prompt function for two defaults:
Please note: the prompt will run only for the missing value ( surname ).
Object destructuring
The destructuring assignment also works with objects.
The basic syntax is:
We should have an existing object on the right side, that we want to split into variables. The left side contains an object-like “pattern” for corresponding properties. In the simplest case, that’s a list of variable names in {...} .
For instance:
Properties options.title , options.width and options.height are assigned to the corresponding variables.
The order does not matter. This works too:
The pattern on the left side may be more complex and specify the mapping between properties and variables.
If we want to assign a property to a variable with another name, for instance, make options.width go into the variable named w , then we can set the variable name using a colon:
The colon shows “what : goes where”. In the example above the property width goes to w , property height goes to h , and title is assigned to the same name.
For potentially missing properties we can set default values using "=" , like this:
Just like with arrays or function parameters, default values can be any expressions or even function calls. They will be evaluated if the value is not provided.
In the code below prompt asks for width , but not for title :
We also can combine both the colon and equality:
If we have a complex object with many properties, we can extract only what we need:
The rest pattern “…”
What if the object has more properties than we have variables? Can we take some and then assign the “rest” somewhere?
We can use the rest pattern, just like we did with arrays. It’s not supported by some older browsers (IE, use Babel to polyfill it), but works in modern ones.
It looks like this:
In the examples above variables were declared right in the assignment: let {…} = {…} . Of course, we could use existing variables too, without let . But there’s a catch.
This won’t work:
The problem is that JavaScript treats {...} in the main code flow (not inside another expression) as a code block. Such code blocks can be used to group statements, like this:
So here JavaScript assumes that we have a code block, that’s why there’s an error. We want destructuring instead.
To show JavaScript that it’s not a code block, we can wrap the expression in parentheses (...) :
Nested destructuring
If an object or an array contains other nested objects and arrays, we can use more complex left-side patterns to extract deeper portions.
In the code below options has another object in the property size and an array in the property items . The pattern on the left side of the assignment has the same structure to extract values from them:
All properties of options object except extra that is absent in the left part, are assigned to corresponding variables:
Finally, we have width , height , item1 , item2 and title from the default value.
Note that there are no variables for size and items , as we take their content instead.
Smart function parameters
There are times when a function has many parameters, most of which are optional. That’s especially true for user interfaces. Imagine a function that creates a menu. It may have a width, a height, a title, items list and so on.
Here’s a bad way to write such a function:
In real-life, the problem is how to remember the order of arguments. Usually IDEs try to help us, especially if the code is well-documented, but still… Another problem is how to call a function when most parameters are ok by default.
That’s ugly. And becomes unreadable when we deal with more parameters.
Destructuring comes to the rescue!
We can pass parameters as an object, and the function immediately destructurizes them into variables:
We can also use more complex destructuring with nested objects and colon mappings:
The full syntax is the same as for a destructuring assignment:
Then, for an object of parameters, there will be a variable varName for property incomingProperty , with defaultValue by default.
Please note that such destructuring assumes that showMenu() does have an argument. If we want all values by default, then we should specify an empty object:
We can fix this by making {} the default value for the whole object of parameters:
In the code above, the whole arguments object is {} by default, so there’s always something to destructurize.
Destructuring assignment allows for instantly mapping an object or array onto many variables.
The full object syntax:
This means that property prop should go into the variable varName and, if no such property exists, then the default value should be used.
Object properties that have no mapping are copied to the rest object.
The full array syntax:
The first item goes to item1 ; the second goes into item2 , all the rest makes the array rest .
It’s possible to extract data from nested arrays/objects, for that the left side must have the same structure as the right one.
We have an object:
Write the destructuring assignment that reads:
- name property into the variable name .
- years property into the variable age .
- isAdmin property into the variable isAdmin (false, if no such property)
Here’s an example of the values after your assignment:
The maximal salary
There is a salaries object:
Create the function topSalary(salaries) that returns the name of the top-paid person.
- If salaries is empty, it should return null .
- If there are multiple top-paid persons, return any of them.
P.S. Use Object.entries and destructuring to iterate over key/value pairs.
Open a sandbox with tests.
Open the solution with tests in a sandbox.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
SyntaxError: Invalid destructuring assignment target in JS
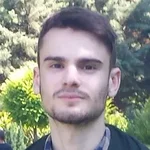
Last updated: Mar 2, 2024 Reading time · 2 min
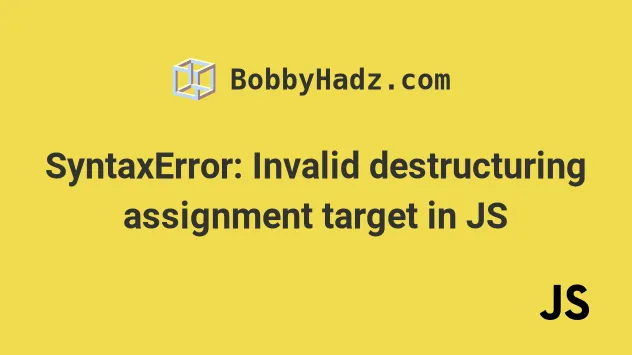
# SyntaxError: Invalid destructuring assignment target in JS
The "SyntaxError: Invalid destructuring assignment target" error occurs when we make a syntax error when declaring or destructuring an object, often in the arguments list of a function.
To solve the error make sure to correct any syntax errors in your code.

Here are some examples of how the error occurs:
# Setting a variable to be equal to multiple objects
The first example throws the error because we set a variable to be equal to multiple objects without the objects being contained in an array.
Instead, you should place the objects in an array or only assign a single value to the variable.
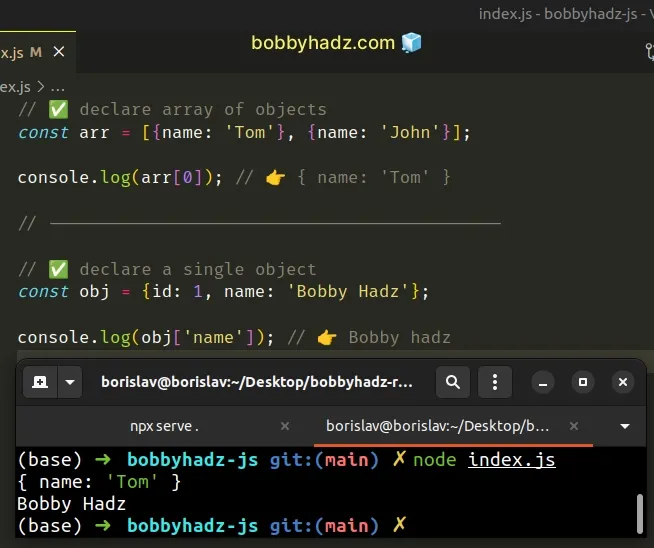
The square brackets [] syntax is used to declare an array and the curly braces {} syntax is used to declare an object.
Arrays are a collection of elements and objects are a mapping of key-value pairs.
# Incorrectly destructuring in a function's arguments
The second example throws the error because we incorrectly destructure an object in a function's arguments.
If you're trying to provide a default value for a function, use the equal sign instead.
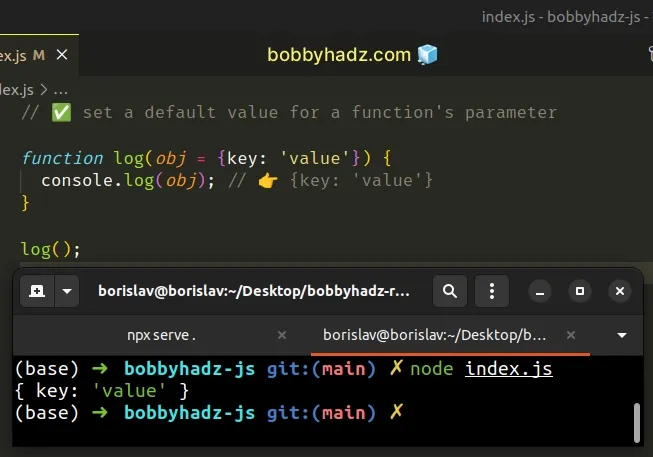
And you can destructure a value from an object argument as follows.
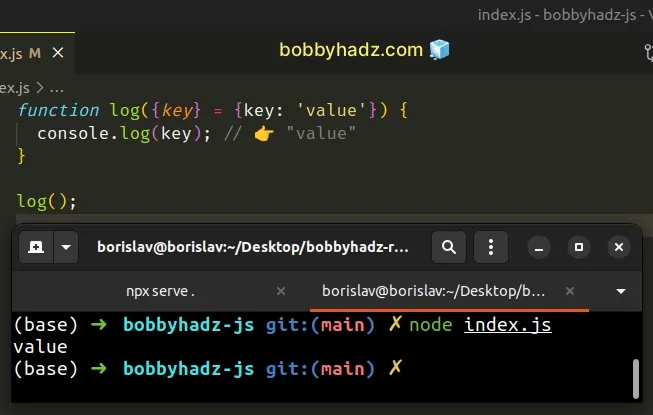
Here's the same example but with arrays.
If you can't figure out where exactly the error occurs, look at the error message in your browser's console or your terminal (if using Node.js).
The screenshot above shows that the error occurs on line 11 in the index.js file.
You can also hover over the squiggly red line to get additional information.
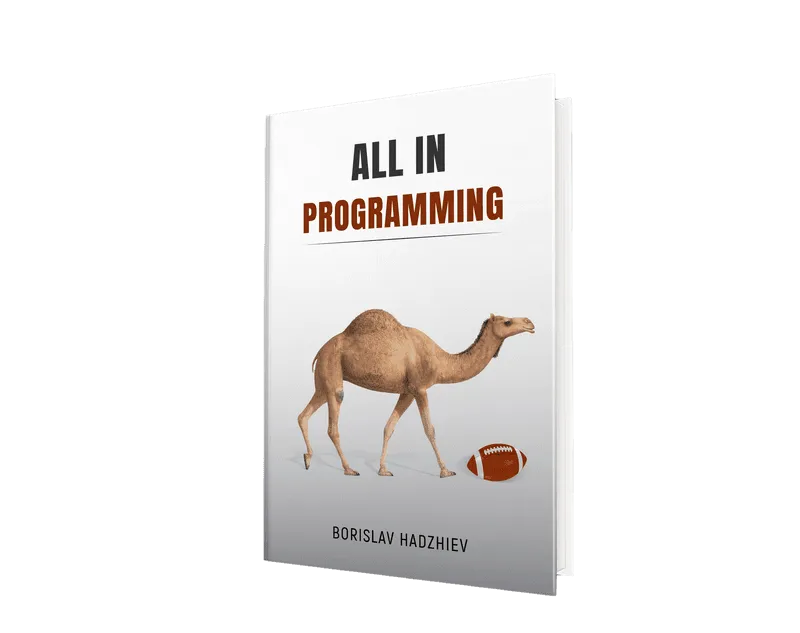
Borislav Hadzhiev
Web Developer
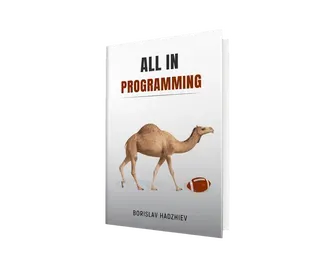
Copyright © 2024 Borislav Hadzhiev
37 Destructuring
- 37.1 A first taste of destructuring
- 37.2 Constructing vs. extracting
- 37.3 Where can we destructure?
- 37.4.1 Property value shorthands
- 37.4.2 Rest properties
- 37.4.3 Syntax pitfall: assigning via object destructuring
- 37.5.1 Array-destructuring works with any iterable
- 37.5.2 Rest elements
- 37.6.1 Array-destructuring: swapping variable values
- 37.6.2 Array-destructuring: operations that return Arrays
- 37.6.3 Object-destructuring: multiple return values
- 37.7.1 Object-destructuring and missing properties
- 37.7.2 Array-destructuring and missing elements
- 37.8.1 You can’t object-destructure undefined and null
- 37.8.2 You can’t Array-destructure non-iterable values
- 37.9 (Advanced)
- 37.10.1 Default values in Array-destructuring
- 37.10.2 Default values in object-destructuring
- 37.11 Parameter definitions are similar to destructuring
- 37.12 Nested destructuring
37.1 A first taste of destructuring
With normal assignment, you extract one piece of data at a time – for example:
With destructuring, you can extract multiple pieces of data at the same time via patterns in locations that receive data. The left-hand side of = in the previous code is one such location. In the following code, the square brackets in line A are a destructuring pattern:
This code does the same as the previous code.
Note that the pattern is “smaller” than the data: we are only extracting what we need.
37.2 Constructing vs. extracting
In order to understand what destructuring is, consider that JavaScript has two kinds of operations that are opposites:
- You can construct compound data, for example, by setting properties and via object literals.
- You can extract data out of compound data, for example, by getting properties.
Constructing data looks as follows:
Extracting data looks as follows:
The operation in line A is new: we declare two variables f2 and l2 and initialize them via destructuring (multivalue extraction).
The following part of line A is a destructuring pattern :
Destructuring patterns are syntactically similar to the literals that are used for multivalue construction. But they appear where data is received (e.g., at the left-hand sides of assignments), not where data is created (e.g., at the right-hand sides of assignments).
37.3 Where can we destructure?
Destructuring patterns can be used at “data sink locations” such as:
Variable declarations:
Assignments:
Parameter definitions:
Note that variable declarations include const and let declarations in for-of loops:
In the next two sections , we’ll look deeper into the two kinds of destructuring: object-destructuring and Array-destructuring.
37.4 Object-destructuring
Object-destructuring lets you batch-extract values of properties via patterns that look like object literals:
You can think of the pattern as a transparent sheet that you place over the data: the pattern key 'street' has a match in the data. Therefore, the data value 'Evergreen Terrace' is assigned to the pattern variable s .
You can also object-destructure primitive values:
And you can object-destructure Arrays:
Why does that work? Array indices are also properties .
37.4.1 Property value shorthands
Object literals support property value shorthands and so do object patterns:
exercises/destructuring/object_destructuring_exrc.mjs
37.4.2 Rest properties
In object literals, you can have spread properties. In object patterns, you can have rest properties (which must come last):
A rest property variable, such as remaining (line A), is assigned an object with all data properties whose keys are not mentioned in the pattern.
remaining can also be viewed as the result of non-destructively removing property a from obj .
37.4.3 Syntax pitfall: assigning via object destructuring
If we object-destructure in an assignment, we are facing a pitfall caused by syntactic ambiguity – you can’t start a statement with a curly brace because then JavaScript thinks you are starting a block:
eval() delays parsing (and therefore the SyntaxError ) until the callback of assert.throws() is executed. If we didn’t use it, we’d already get an error when this code is parsed and assert.throws() wouldn’t even be executed.
The workaround is to put the whole assignment in parentheses:
37.5 Array-destructuring
Array-destructuring lets you batch-extract values of Array elements via patterns that look like Array literals:
You can skip elements by mentioning holes inside Array patterns:
The first element of the Array pattern in line A is a hole, which is why the Array element at index 0 is ignored.
37.5.1 Array-destructuring works with any iterable
Array-destructuring can be applied to any value that is iterable, not just to Arrays:
37.5.2 Rest elements
In Array literals, you can have spread elements. In Array patterns, you can have rest elements (which must come last):
A rest element variable, such as remaining (line A), is assigned an Array with all elements of the destructured value that were not mentioned yet.
37.6 Examples of destructuring
37.6.1 array-destructuring: swapping variable values.
You can use Array-destructuring to swap the values of two variables without needing a temporary variable:
37.6.2 Array-destructuring: operations that return Arrays
Array-destructuring is useful when operations return Arrays, as does, for example, the regular expression method .exec() :
37.6.3 Object-destructuring: multiple return values
Destructuring is very useful if a function returns multiple values – either packaged as an Array or packaged as an object.
Consider a function findElement() that finds elements in an Array:
Its second parameter is a function that receives the value and index of an element and returns a boolean indicating if this is the element the caller is looking for.
We are now faced with a dilemma: Should findElement() return the value of the element it found or the index? One solution would be to create two separate functions, but that would result in duplicated code because both functions would be very similar.
The following implementation avoids duplication by returning an object that contains both index and value of the element that is found:
Destructuring helps us with processing the result of findElement() :
As we are working with property keys, the order in which we mention value and index doesn’t matter:
The kicker is that destructuring also serves us well if we are only interested in one of the two results:
All of these conveniences combined make this way of handling multiple return values quite versatile.
37.7 What happens if a pattern part does not match anything?
What happens if there is no match for part of a pattern? The same thing that happens if you use non-batch operators: you get undefined .
37.7.1 Object-destructuring and missing properties
If a property in an object pattern has no match on the right-hand side, you get undefined :
37.7.2 Array-destructuring and missing elements
If an element in an Array pattern has no match on the right-hand side, you get undefined :
37.8 What values can’t be destructured?
37.8.1 you can’t object-destructure undefined and null.
Object-destructuring only fails if the value to be destructured is either undefined or null . That is, it fails whenever accessing a property via the dot operator would fail too.
37.8.2 You can’t Array-destructure non-iterable values
Array-destructuring demands that the destructured value be iterable. Therefore, you can’t Array-destructure undefined and null . But you can’t Array-destructure non-iterable objects either:
See quiz app .
37.9 (Advanced)
All of the remaining sections are advanced.
37.10 Default values
Normally, if a pattern has no match, the corresponding variable is set to undefined :
If you want a different value to be used, you need to specify a default value (via = ):
In line A, we specify the default value for p to be 123 . That default is used because the data that we are destructuring has no property named prop .
37.10.1 Default values in Array-destructuring
Here, we have two default values that are assigned to the variables x and y because the corresponding elements don’t exist in the Array that is destructured.
The default value for the first element of the Array pattern is 1 ; the default value for the second element is 2 .
37.10.2 Default values in object-destructuring
You can also specify default values for object-destructuring:
Neither property key first nor property key last exist in the object that is destructured. Therefore, the default values are used.
With property value shorthands, this code becomes simpler:
37.11 Parameter definitions are similar to destructuring
Considering what we have learned in this chapter, parameter definitions have much in common with an Array pattern (rest elements, default values, etc.). In fact, the following two function declarations are equivalent:
37.12 Nested destructuring
Until now, we have only used variables as assignment targets (data sinks) inside destructuring patterns. But you can also use patterns as assignment targets, which enables you to nest patterns to arbitrary depths:
Inside the Array pattern in line A, there is a nested object pattern at index 1.
Nested patterns can become difficult to understand, so they are best used in moderation.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Incorrectly parsed object destructuring assignment with short-hand properties #5035
zhuravlikjb commented Sep 30, 2015
mhegazy commented Sep 30, 2015
Sorry, something went wrong.
vladima commented Sep 30, 2015
ahejlsberg commented Sep 30, 2015
Zhuravlikjb commented oct 1, 2015.
Description
Array destructuring, object destructuring, specifications, browser compatibility.
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables.
The source for this interactive example is stored in a GitHub repository. If you'd like to contribute to the interactive examples project, please clone https://github.com/mdn/interactive-examples and send us a pull request.
The object and array literal expressions provide an easy way to create ad hoc packages of data.
The destructuring assignment uses similar syntax, but on the left-hand side of the assignment to define what values to unpack from the sourced variable.
This capability is similar to features present in languages such as Perl and Python.
Basic variable assignment
Assignment separate from declaration.
A variable can be assigned its value via destructuring separate from the variable's declaration.

Default values
A variable can be assigned a default, in the case that the value unpacked from the array is undefined .
Swapping variables
Two variables values can be swapped in one destructuring expression.
Without destructuring assignment, swapping two values requires a temporary variable (or, in some low-level languages, the XOR-swap trick ).
Parsing an array returned from a function
It's always been possible to return an array from a function. Destructuring can make working with an array return value more concise.
In this example, f() returns the values [1, 2] as its output, which can be parsed in a single line with destructuring.
Ignoring some returned values
You can ignore return values that you're not interested in:
You can also ignore all returned values:
Assigning the rest of an array to a variable
When destructuring an array, you can unpack and assign the remaining part of it to a variable using the rest pattern:
Be aware that a SyntaxError will be thrown if a trailing comma is used on the left-hand side with a rest element:
Unpacking values from a regular expression match
When the regular expression exec() method finds a match, it returns an array containing first the entire matched portion of the string and then the portions of the string that matched each parenthesized group in the regular expression. Destructuring assignment allows you to unpack the parts out of this array easily, ignoring the full match if it is not needed.
Basic assignment
Assignment without declaration.
A variable can be assigned its value with destructuring separate from its declaration.
Notes : The parentheses ( ... ) around the assignment statement are required when using object literal destructuring assignment without a declaration.
{a, b} = {a: 1, b: 2} is not valid stand-alone syntax, as the {a, b} on the left-hand side is considered a block and not an object literal.
However, ({a, b} = {a: 1, b: 2}) is valid, as is var {a, b} = {a: 1, b: 2}
Your ( ... ) expression needs to be preceded by a semicolon or it may be used to execute a function on the previous line.
Assigning to new variable names
A property can be unpacked from an object and assigned to a variable with a different name than the object property.
Here, for example, var {p: foo} = o takes from the object o the property named p and assigns it to a local variable named foo .
A variable can be assigned a default, in the case that the value unpacked from the object is undefined .
Assigning to new variables names and providing default values
A property can be both 1) unpacked from an object and assigned to a variable with a different name and 2) assigned a default value in case the unpacked value is undefined .
Setting a function parameter's default value
Es5 version, es2015 version.
In the function signature for drawES2015Chart above, the destructured left-hand side is assigned to an empty object literal on the right-hand side: {size = 'big', coords = {x: 0, y: 0}, radius = 25} = {} . You could have also written the function without the right-hand side assignment. However, if you leave out the right-hand side assignment, the function will look for at least one argument to be supplied when invoked, whereas in its current form, you can simply call drawES2015Chart() without supplying any parameters. The current design is useful if you want to be able to call the function without supplying any parameters, the other can be useful when you want to ensure an object is passed to the function.
- Nested object and array destructuring
For of iteration and destructuring
Unpacking fields from objects passed as function parameter.
This unpacks the id , displayName and firstName from the user object and prints them.
Computed object property names and destructuring
Computed property names, like on object literals , can be used with destructuring.
Rest in Object Destructuring
The Rest/Spread Properties for ECMAScript proposal (stage 3) adds the rest syntax to destructuring. Rest properties collect the remaining own enumerable property keys that are not already picked off by the destructuring pattern.
Invalid JavaScript identifier as a property name
Destructuring can be used with property names that are not valid JavaScript identifiers by providing an alternative identifer that is valid.
Combined Array and Object Destructuring
Array and Object destructuring can be combined. Say you want the third element in the array props below, and then you want the name property in the object, you can do the following:
- Assignment operators
- "ES6 in Depth: Destructuring" on hacks.mozilla.org
Document Tags and Contributors
- Destructuring
- Destructuring_assignment
- ECMAScript 2015
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Using promises
- Iterators and generators
- Meta programming
- JavaScript modules
- Client-side web APIs
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.ListFormat
- Intl.Locale
- Intl.NumberFormat
- Intl.PluralRules
- Intl.RelativeTimeFormat
- ReferenceError
- SharedArrayBuffer
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Arithmetic operators
- Array comprehensions
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) operator
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical operators
- Object initializer
- Operator precedence
- (currently at stage 1) pipes the value of an expression into a function. This allows the creation of chained function calls in a readable manner. The result is syntactic sugar in which a function call with a single argument can be written like this:">Pipeline operator
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for await...of
- for each...in
- function declaration
- import.meta
- try...catch
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- The arguments object
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration`X' before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: 'x' is not iterable
- TypeError: More arguments needed
- TypeError: Reduce of empty array with no initial value
- TypeError: can't access dead object
- TypeError: can't access property "x" of "y"
- TypeError: can't assign to property "x" on "y": not an object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cannot use 'in' operator to search for 'x' in 'y'
- TypeError: cyclic object value
- TypeError: invalid 'instanceof' operand 'x'
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- X.prototype.y called on incompatible type
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
Learn the best of web development
Get the latest and greatest from MDN delivered straight to your inbox.
Thanks! Please check your inbox to confirm your subscription.
If you haven’t previously confirmed a subscription to a Mozilla-related newsletter you may have to do so. Please check your inbox or your spam filter for an email from us.

- Performance
- Announcements
- cfexchangemail
- cfhtmltopdf
- CFML Reference
- cfprocparam
- cfspreadsheet
- ColdFusion 10
- ColdFusion 11
- ColdFusion 2016
- ColdFusion 2018
- ColdFusion 20121
- ColdFusion Archives(or CAR)
- ColdFusion Mobile
- Enhancement
- Iconography
- Installation
- JDBC Connection
- JSON Backward Compatibility
- Language Enhancements
- Member Functions
- New feature
- New features
- QueryExecute
- serializeJSON
- session - less site
- SOLR Search
- Spreadsheet
- Usability Issues
- Application Datasource
- Application Monitoring
- Application Performance
- Distributed
- Performance Monitoring Toolset
- ColdFusion Summit Survey
- ColdFusion Webinar
- Developer week
- Govt Summit
- Adobe Developer Connection
- Amazon AWS Webinar
- Builder Plugin
- ColdFusion Community Portal
- ColdFusion seminar
- End of Sale
- Learn CF in a Week
- Linux Download
- Marketing Manager
- Migration Guide
- Online ColdFusion Meetup
- Windows 2016 Support
- Windows 2019 Support
- Adobe Certification
- Getting Started
- Discussions

This blog contains details about DeStructuring Assignment.
The Destructuring Assignment syntax is a way to assign the values from Arrays and Structs( Complex Objects ), into distinct variables.
Conventional way of doing it is to store the object and then retrieve it using the JSON Object API calls. However using DeStructuring , it becomes very simple, where just by writing a line of code, one can extract the relevant attributes and choose to ignore the other ones.
Note: The above defined syntax of [variable1, variable2, …………, variableN] = [value1, value2, ……., valueN] , is written for the sake of simplicity. This syntax can be as complex as possible.
For example here the LVAL i.e [variable1, variable2, …………, variableN] , can be substituted with complex values like Array/Struct elements or even a complex Object.
Similarly the RVAL i.e [value1, value2, ……., valueN] , can be substituted by Array/Struct elements as well as Array/Struct References or even a complex Object.
Lets get into more details and try to simulate the different scenarios through examples and see how we can write some short, concise and more powerful syntax using this feature.
- Multi-Initializer Statements with same #LVAL and #RVAL
- Multi-Initializer Statements with RVAL as an Array Reference
- Multi-Initializer Statements by ignoring extra RVALs
- Multi-Initializer Statements to ignore some values from RVAL
- Multi-Initializer Statements using Rest Operator(…)
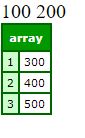
- Complex Multi-Initializer Statements
- Swapping Values
Object Destructuring : Up till now, we have mostly seen the examples of ArrayInitializers as well as complex DeStructuring. DeStructuring is also supported for Structs as well as Complex Objects. Lets take some examples to simulate Object Destructuring and how we can leverage this functionality for Structs.
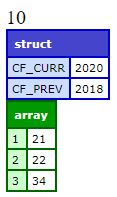
- Multi-Initializer Statements using Object Reference
- Multi-Initializer Statements with LVAL Object having default Values
- Nested Object Destructuring: Using this one can initialize attributeValues from childObjects within ParentObject.

- Rest Operator Support in Objects Destructuring Multi-Initializer Statements
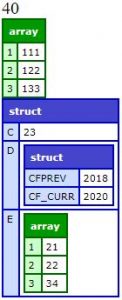
- Ignoring RVAL in Objects Destructuring
- Destructuring Support in local variables using var keyword
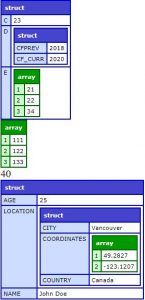
- Destructuring Support using Final keyword in Global as well as Local Scope

- Object Destructuring static keyword support : Object Destructuring can also be leveraged in components using the static keyword. A sample code-snippet is as shown below.

This is a great feature, love using it in JavaScript, but the CF implementation misses it’s most powerful implementation and that is using it with function parameters. One should be able to call a function and pass a struct of named params with destructuring notation and have the inputs mapped. Example: var bar = {opt1: 1, opt2: 2, opt3:3}; function foo(required numeric opt1, any …opts){ writedump(arguments); }
foo({bar});
Expected output: { opt1: 1, opts: {opt2: 2, opt3: 3} }
I hope this makes sense. It is how I use this functionality in JS.

Thanks for sharing the observation. And while someone at Adobe MAY see this and respond, you would do better to file this also as a feature request at https://tracker.adobe.com . Adobe really does pay attention to that (and sometimes to here).
Also, if you do that, you may want to help those not familiar with the implementation in JS (or the feature in general) by showing a working example in JS, such as could be could run at jsfiddle.net. That could help some better connect the dots of how what you propose does indeed work in JS.
Thanks Charlie, MDN already has examples of object destructuring in function parameters in their docs. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Destructuring_assignment#object_destructuring
I thought you are someone at Adobe At least that’s the impression I have had from the last couple of developer conferences. I could be wrong. Anyway, I will submit it in the tracker.
Looks like someone made this suggestion in 2019 and they marked it as “fixed”.
https://tracker.adobe.com/#/view/CF-4205217
Nope, I don’t work for Adobe. I do work with them, as an ACP (Adobe Community Professional), and I may evangelize (and even do apologetics) on their behalf, but nope, not an employee. As always, I’m just trying to help.
BTW, whenever I speak, I clearly indicate that I am an independent consultant, on the front slide, then later when I introduce myself after the intro to the talk, and then also on the bottom of all my slides. I’d never intend (at all) to convey or leave open the impression that I work for Adobe (or anyone, but myself).
About that MSDN page, I wonder if to meant to share another. That one does certainly discuss destructuring assignment, but I didn’t see any that showed its use in passing function arguments as you proposed. If there is another, that would be a help for many, I’m sure.
Look for ‘Unpacking fields from objects passed as a function parameter’ as a simple example.
Using spread in function calls: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Spread_syntax#spread_in_function_calls
Interesting about the existing tracker ticket. And you added your comment asking about it being fixed there, and I offered a possible explanation. But in case people here don’t go there, let me offer it (and a bit more) here:
The info from Adobe also indicated “For2022Triage”, which is certainly interesting to see. It suggests perhaps maybe the “fix” is coming in a CF2022. I agree they could be more clear, as that’s in the “component” field rather than the update/build. Perhaps they have to do this because there is not technically yet a cf2022.
And I’d heard some other rumblings that the next CF version would be called that (or would come out this year), so we shall see, on both fronts. 🙂
Also, you offered there a link to a cffiddle example you created. I’m sharing that also for readers here, who may not have gone there. Also, about that example, it does not “fail”. You may want to revise it or clarify better what output yo unexpected it to produce.
Finally, of course, the folks there wouldn’t know about our discussions here, so I added a link there pointing to this. Such cross-posting of discussions can get messy, but I’m just trying to help given what you’ve offered.
Thanks for the additional feedback.
https://codepen.io/Anthony-Kolka/pen/WNZMZZg
Ah, ok (on that “unpacking” example). I was focusing more on the rest (“…”) aspect in your first example here and didn’t see that there.
But I see now how you’d hope to be able to name a struct key as the arg of a function, and pass the function the struct and have it figure it out/unpack it. I understand now, and hopefully this example (on top of yours and Abram’s in the tracker ticket) may help Adobe see what you want and deliver that in that seemingly upcoming enhancement.

So pretty interesting and I’ll admit that some of this went over my head and made me think hard about what was going on. I’d be interested in seeing a real world example of how you would use this if you were able to put one together. This is pretty abstract and I think it’d help me grasp it more firmly seeing it in a real world scenario. That being said I think I can see how I may could use this so will start trying to play.
Also I am assuming this is CF2021 specific? On CFFiddle the code would only run with 2021 selected so may be worthy of noting in the description unless I am incorrect that is.
Grae, yep, this is new to cf2021. And I can relate to your sentiment, having felt similarly when I first saw the feature and similar examples in the pre-release.
Fwiw, I will say that the feature is a generic one, and if you Google on it you’ll find it’s supported by other languages like Javascript, Python, etc.
And if you find resources on using the feature with those, the examples are similar, showing “how it works” but not so much “why would one bother”. I suspect they figure people can be left to connect the dots for the various ways one might leverage the capabilities.
But fwiw, as I kept looking for anything more, I did find this which you may appreciate :
https://www.smashingmagazine.com/2019/09/reintroduction-destructuring-assignment/
No, it’s not cf-specific, but again neither is the feature. Hope it may help.
For real world examples check out React’s state implementation. Especially Redux.
You must be logged in to post a comment.

No credit card required.

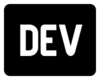
DEV Community

Posted on Feb 1, 2023
Understanding the Destructuring assignment in JavaScript
I needed a refresher on a few JavaScript features and concepts, so I went to do some reading on a couple of them. In today's article, we are going to be looking at the Destructuring Assignment. If you are not already aware, it is one of the many features for working with arrays and objects that was introduced to JavaScript in ES6(the 2015 edition of JavaScript). This article is a succinct collection of the knowledge I have gathered from various resources on the topic of interest. Without any further long talk, let's get to business!
What is Destructuring?
The Destructuring assignment syntax is a JavaScript expression that allows you to unpack values from arrays, or properties from objects, into distinct variables. Destructuring does not mean “destructive” or "destroyed". It’s called “destructuring assignment” because it “destructurizes” by copying items into variables. The original array or object itself remains unmodified. Destructuring can greatly reduce the lines of code necessary to manipulate data in these structures. There are two types of destructuring: Object destructuring and Array destructuring:
Object Destructuring
Object destructuring allows you to create new variables using an object property as their values. Consider the following example; an object representing a student with an id , name and dateOfBirth .
Traditionally, if you were to create a new variable for each property, you would have to assign each variable individually which is a lot of repetition if you ask me. Take a lot at what I'm talking about:
With object destructuring, this can all be done in a single line of code. By enclosing each variable in curly brackets {} , JavaScript will create new variables from each property with the same name:
Log these value to a console and you'll see that the values of the variables are the values of the properties of the student object.
It is important to note that destructuring an object does not effect a change on the original object(i.e the original object is not modified). If you call the original student object you'll see that it's properties are unchanged.
The default assignment for object destructuring creates new variables with the same name as the object property. If you do not want the new variable to have the same name as the object property, you have the option of giving the new variable a different name by using a colon ( : ) to decide the name, as seen in the following example:
We can also assign default values to variables whose property may not exist in the object we want to destructure. This will prevent the variable from having an undefined value assigned to it. The code below demonstrates this:
Pros of Object Destructuring
It allows us to write code that is more succint, since it allows us to bundle variables inside one object and then access the individual elements in another function without needing to use the dot notation.
It makes sure the code doesn't break in case a value is missing since it allows us to set default values for specific variables
When working with large frameworks that pass objects to functions with a lot of values, if we only need one or two values, we can destructure it. This helps make the code easier to work with.
Using object destructuring in JavaScript has some cons too. Some of them are:
If an object has a lot of variables, it becomes very tedious to destructure it. In this case, use the dot notation.
In case an object is different from what was expected and we don't account for that, it might result in bugs in the code.
Array Destructing
Array destructuring allows you to create new variables using an array item as a value. The syntax of Array Destructuring is as follows:
As you can see, the syntax is pretty simple. It’s just a shorter way to write the following:
In array destructuring, unwanted items of the array can also be thrown away by adding an extra comma. See the example below:
In the code above, the second item of the array is skipped, the third one is assigned to title , and the rest of the array items are also skipped as there are no variables for them.
If the array we want to destructure is shorter than the list of variables on the left, there’ll be no errors. Absent values are assigned undefined :
If we want a default value to replace the missing one, we can provide it using = :
Default values can be more complex expressions or even function calls. They are evaluated only if the value is not provided.
The fact that many other programming languages do not have a corresponding syntax for destructuring might give this feature a learning curve both for new JavaScript developers and those coming from another language. Be persistent and as time goes by, you'll get the hang of it. Hope to meet you soon in another article.
Top comments (2)
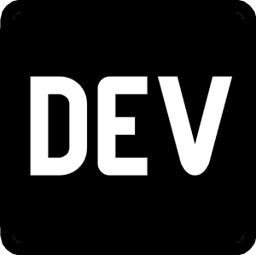
Templates let you quickly answer FAQs or store snippets for re-use.

- Email [email protected]
- Joined Jul 21, 2021
Great article, you got my follow, keep writing!

- Location Hamilton, Ontario, Canada
- Education Mohawk College Of Technology Hamilton, Ontario, CA
- Joined Jun 27, 2020
Thank you Al. Your comment is highly appreciated.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
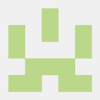
Mastering Next.js 13/14 : App Router and API Routes
Fabrikapp - Apr 19
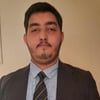
SVM and Kernels: The Math that Makes Classification Magic
HarshTiwari1710 - Apr 5
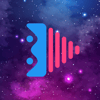
Getting credentials for your Discord Activity
WavePlay Staff - Apr 18
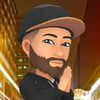
🔍 Searching the web
Suneeh - Apr 5
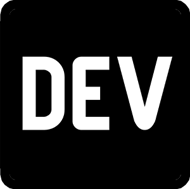
We're a place where coders share, stay up-to-date and grow their careers.
Destructuring assignment
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables.
Description
The object and array literal expressions provide an easy way to create ad hoc packages of data.
The destructuring assignment uses similar syntax but uses it on the left-hand side of the assignment instead. It defines which values to unpack from the sourced variable.
Similarly, you can destructure objects on the left-hand side of the assignment.
This capability is similar to features present in languages such as Perl and Python.
For features specific to array or object destructuring, refer to the individual examples below.
Binding and assignment
For both object and array destructuring, there are two kinds of destructuring patterns: binding pattern and assignment pattern , with slightly different syntaxes.
In binding patterns, the pattern starts with a declaration keyword ( var , let , or const ). Then, each individual property must either be bound to a variable or further destructured.
All variables share the same declaration, so if you want some variables to be re-assignable but others to be read-only, you may have to destructure twice — once with let , once with const .
In many other syntaxes where the language binds a variable for you, you can use a binding destructuring pattern. These include:
- The looping variable of for...in for...of , and for await...of loops;
- Function parameters;
- The catch binding variable.
In assignment patterns, the pattern does not start with a keyword. Each destructured property is assigned to a target of assignment — which may either be declared beforehand with var or let , or is a property of another object — in general, anything that can appear on the left-hand side of an assignment expression.
Note: The parentheses ( ... ) around the assignment statement are required when using object literal destructuring assignment without a declaration.
{ a, b } = { a: 1, b: 2 } is not valid stand-alone syntax, as the { a, b } on the left-hand side is considered a block and not an object literal according to the rules of expression statements . However, ({ a, b } = { a: 1, b: 2 }) is valid, as is const { a, b } = { a: 1, b: 2 } .
If your coding style does not include trailing semicolons, the ( ... ) expression needs to be preceded by a semicolon, or it may be used to execute a function on the previous line.
Note that the equivalent binding pattern of the code above is not valid syntax:
You can only use assignment patterns as the left-hand side of the assignment operator. You cannot use them with compound assignment operators such as += or *= .
Default value
Each destructured property can have a default value . The default value is used when the property is not present, or has value undefined . It is not used if the property has value null .
The default value can be any expression. It will only be evaluated when necessary.
Rest property
You can end a destructuring pattern with a rest property ...rest . This pattern will store all remaining properties of the object or array into a new object or array.
The rest property must be the last in the pattern, and must not have a trailing comma.
Array destructuring
Basic variable assignment, destructuring with more elements than the source.
In an array destructuring from an array of length N specified on the right-hand side of the assignment, if the number of variables specified on the left-hand side of the assignment is greater than N , only the first N variables are assigned values. The values of the remaining variables will be undefined.
Swapping variables
Two variables values can be swapped in one destructuring expression.
Without destructuring assignment, swapping two values requires a temporary variable (or, in some low-level languages, the XOR-swap trick ).
Parsing an array returned from a function
It's always been possible to return an array from a function. Destructuring can make working with an array return value more concise.
In this example, f() returns the values [1, 2] as its output, which can be parsed in a single line with destructuring.
Ignoring some returned values
You can ignore return values that you're not interested in:
You can also ignore all returned values:
Using a binding pattern as the rest property
The rest property of array destructuring assignment can be another array or object binding pattern. The inner destructuring destructures from the array created after collecting the rest elements, so you cannot access any properties present on the original iterable in this way.
These binding patterns can even be nested, as long as each rest property is the last in the list.
On the other hand, object destructuring can only have an identifier as the rest property.
Unpacking values from a regular expression match
When the regular expression exec() method finds a match, it returns an array containing first the entire matched portion of the string and then the portions of the string that matched each parenthesized group in the regular expression. Destructuring assignment allows you to unpack the parts out of this array easily, ignoring the full match if it is not needed.
Using array destructuring on any iterable
Array destructuring calls the iterable protocol of the right-hand side. Therefore, any iterable, not necessarily arrays, can be destructured.
Non-iterables cannot be destructured as arrays.
Iterables are only iterated until all bindings are assigned.
The rest binding is eagerly evaluated and creates a new array, instead of using the old iterable.
Object destructuring
Basic assignment, assigning to new variable names.
A property can be unpacked from an object and assigned to a variable with a different name than the object property.
Here, for example, const { p: foo } = o takes from the object o the property named p and assigns it to a local variable named foo .
Assigning to new variable names and providing default values
A property can be both
- Unpacked from an object and assigned to a variable with a different name.
- Assigned a default value in case the unpacked value is undefined .
Unpacking properties from objects passed as a function parameter
Objects passed into function parameters can also be unpacked into variables, which may then be accessed within the function body. As for object assignment, the destructuring syntax allows for the new variable to have the same name or a different name than the original property, and to assign default values for the case when the original object does not define the property.
Consider this object, which contains information about a user.
Here we show how to unpack a property of the passed object into a variable with the same name. The parameter value { id } indicates that the id property of the object passed to the function should be unpacked into a variable with the same name, which can then be used within the function.
You can define the name of the unpacked variable. Here we unpack the property named displayName , and rename it to dname for use within the function body.
Nested objects can also be unpacked. The example below shows the property fullname.firstName being unpacked into a variable called name .
Setting a function parameter's default value
Default values can be specified using = , and will be used as variable values if a specified property does not exist in the passed object.
Below we show a function where the default size is 'big' , default co-ordinates are x: 0, y: 0 and default radius is 25.
In the function signature for drawChart above, the destructured left-hand side has a default value of an empty object = {} .
You could have also written the function without that default. However, if you leave out that default value, the function will look for at least one argument to be supplied when invoked, whereas in its current form, you can call drawChart() without supplying any parameters. Otherwise, you need to at least supply an empty object literal.
For more information, see Default parameters > Destructured parameter with default value assignment .
Nested object and array destructuring
For of iteration and destructuring, computed object property names and destructuring.
Computed property names, like on object literals , can be used with destructuring.
Invalid JavaScript identifier as a property name
Destructuring can be used with property names that are not valid JavaScript identifiers by providing an alternative identifier that is valid.
Destructuring primitive values
Object destructuring is almost equivalent to property accessing . This means if you try to destruct a primitive value, the value will get wrapped into the corresponding wrapper object and the property is accessed on the wrapper object.
Same as accessing properties, destructuring null or undefined throws a TypeError .
This happens even when the pattern is empty.
Combined array and object destructuring
Array and object destructuring can be combined. Say you want the third element in the array props below, and then you want the name property in the object, you can do the following:
The prototype chain is looked up when the object is deconstructed
When deconstructing an object, if a property is not accessed in itself, it will continue to look up along the prototype chain.
Specifications
Browser compatibility.
- Assignment operators
- ES6 in Depth: Destructuring on hacks.mozilla.org (2015)
© 2005–2023 MDN contributors. Licensed under the Creative Commons Attribution-ShareAlike License v2.5 or later. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Destructuring_assignment
ES6 In Depth: Destructuring
ES6 In Depth is a series on new features being added to the JavaScript programming language in the 6th Edition of the ECMAScript standard, ES6 for short.
Editor’s note: An earlier version of today’s post, by Firefox Developer Tools engineer Nick Fitzgerald , originally appeared on Nick’s blog as Destructuring Assignment in ES6 .
What is destructuring assignment?
Destructuring assignment allows you to assign the properties of an array or object to variables using syntax that looks similar to array or object literals. This syntax can be extremely terse, while still exhibiting more clarity than the traditional property access.
Without destructuring assignment, you might access the first three items in an array like this:
With destructuring assignment, the equivalent code becomes more concise and readable:
SpiderMonkey (Firefox’s JavaScript engine) already has support for most of destructuring, but not quite all of it. Track SpiderMonkey’s destructuring (and general ES6) support in bug 694100 .
Destructuring arrays and iterables
We already saw one example of destructuring assignment on an array above. The general form of the syntax is:
This will just assign variable1 through variableN to the corresponding item in the array. If you want to declare your variables at the same time, you can add a var , let , or const in front of the assignment:
In fact, variable is a misnomer since you can nest patterns as deep as you would like:
And you can capture all trailing items in an array with a “rest” pattern:
When you access items in the array that are out of bounds or don’t exist, you get the same result you would by indexing: undefined .
Note that destructuring assignment with an array assignment pattern also works for any iterable:
Destructuring objects
Destructuring on objects lets you bind variables to different properties of an object. You specify the property being bound, followed by the variable you are binding its value to.
There is a helpful syntactical shortcut for when the property and variable names are the same:
And just like destructuring on arrays, you can nest and combine further destructuring:
When you destructure on properties that are not defined, you get undefined :
One potential gotcha you should be aware of is when you are using destructuring on an object to assign variables, but not to declare them (when there is no let , const , or var ):
This happens because the JavaScript grammar tells the engine to parse any statement starting with { as a block statement (for example, { console } is a valid block statement). The solution is to either wrap the whole expression in parentheses:
Destructuring values that are not an object, array, or iterable
When you try to use destructuring on null or undefined , you get a type error:
However, you can destructure on other primitive types such as booleans, numbers, and strings, and get undefined :
This may come unexpected, but upon further examination the reason turns out to be simple. When using an object assignment pattern, the value being destructured is required to be coercible to an Object . Most types can be converted to an object, but null and undefined may not be converted. When using an array assignment pattern, the value must have an iterator .
Default values
You can also provide default values for when the property you are destructuring is not defined:
(Editor’s note: This feature is currently implemented in Firefox only for the first two cases, not the third. See bug 932080 .)
Practical applications of destructuring
Function parameter definitions.
As developers, we can often expose more ergonomic APIs by accepting a single object with multiple properties as a parameter instead of forcing our API consumers to remember the order of many individual parameters. We can use destructuring to avoid repeating this single parameter object whenever we want to reference one of its properties:
This is a simplified snippet of real world code from the Firefox DevTools JavaScript debugger (which is also implemented in JavaScript—yo dawg). We have found this pattern particularly pleasing.
Configuration object parameters
Expanding on the previous example, we can also give default values to the properties of the objects we are destructuring. This is particularly helpful when we have an object that is meant to provide configuration and many of the object’s properties already have sensible defaults. For example, jQuery’s ajax function takes a configuration object as its second parameter, and could be rewritten like this:
This avoids repeating var foo = config.foo || theDefaultFoo; for each property of the configuration object.
(Editor’s note: Unfortunately, default values within object shorthand syntax still aren’t implemented in Firefox. I know, we’ve had several paragraphs to work on it since that earlier note. See bug 932080 for the latest updates.)
With the ES6 iteration protocol
ECMAScript 6 also defines an iteration protocol , which we talked about earlier in this series. When you iterate over Map s (an ES6 addition to the standard library) , you get a series of [key, value] pairs. We can destructure this pair to get easy access to both the key and the value:
Iterate over only the keys:
Or iterate over only the values:
Multiple return values
Although multiple return values aren’t baked into the language proper, they don’t need to be because you can return an array and destructure the result:
Alternatively, you can use an object as the container and name the returned values:
Both of these patterns end up much better than holding onto the temporary container:
Or using continuation passing style:
Importing names from a CommonJS module
Not using ES6 modules yet? Still using CommonJS modules? No problem! When importing some CommonJS module X, it is fairly common that module X exports more functions than you actually intend to use. With destructuring, you can be explicit about which parts of a given module you’d like to use and avoid cluttering your namespace:
(And if you do use ES6 modules, you know that a similar syntax is available in import declarations.)
So, as you can see, destructuring is useful in many individually small cases. At Mozilla we’ve had a lot of experience with it. Lars Hansen introduced JS destructuring in Opera ten years ago, and Brendan Eich added support to Firefox a bit later. It shipped in Firefox 2. So we know that destructuring sneaks into your everyday use of the language, quietly making your code a bit shorter and cleaner all over the place.
Five weeks ago, we said that ES6 would change the way you write JavaScript. It is this sort of feature we had particularly in mind: simple improvements that can be learned one at a time. Taken together, they will end up affecting every project you work on. Revolution by way of evolution.
Updating destructuring to comply with ES6 has been a team effort. Special thanks to Tooru Fujisawa (arai) and Arpad Borsos (Swatinem) for their excellent contributions.
Support for destructuring is under development for Chrome, and other browsers will undoubtedly add support in time. For now, you’ll need to use Babel or Traceur if you want to use destructuring on the Web.
Thanks again to Nick Fitzgerald for this week’s post.
Next week, we’ll cover a feature that is nothing more or less than a slightly shorter way to write something JS already has—something that has been one of the fundamental building blocks of the language all along. Will you care? Is slightly shorter syntax something you can get excited about? I confidently predict the answer is yes, but don’t take my word for it. Join us next week and find out, as we look at ES6 arrow functions in depth.
Jason Orendorff
ES6 In Depth editor
I like computing, bicycles, hiphop, books, and pen plotters. My pronouns are he/him.
- fitzgeraldnick.com
More articles by Nick Fitzgerald…
- https://blog.mozilla.org/jorendorff/
More articles by Jason Orendorff…
Discover great resources for web development
Sign up for the Mozilla Developer Newsletter:
Thanks! Please check your inbox to confirm your subscription.
If you haven’t previously confirmed a subscription to a Mozilla-related newsletter you may have to do so. Please check your inbox or your spam filter for an email from us.
12 comments
This is not allowed anymore by ES6: “`js ({ safe }) = {}; “` It has to be: “`js ({ safe } = {}); “`
Oddly enough they only show the right example on their website: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Destructuring_assignment
That’s because I updated the MDN page awhile back. ;-)
Woops! Updated. Thanks!
Is there a canonical source where can I keep up to date with these kinds of changes?
Hope someday we can have a cool pattern matching feature :)
Feels a bit like Erlang’s pattern-matching. Neat.
Adding to the transpilers list, you can also use Typescript 1.5 (still in beta) to have destructuring support :) issue [here]( https://github.com/Microsoft/TypeScript/issues/240 )
Will this be something that can be used in the other browsers as well? I just see this working for Firefox?
Yup, but it might be a little while until support exists across all the browsers. See the end of the article for your options for working around this, eg Traceur, Babel, TypeScript, etc.
var first = someArray[0], second = someArray[1], third = someArray[2]; would kind of align nicer with the comparison to var [first, second, third] = someArray;
Destructuring would be 1000% easier to read if it just used reverse fat arrow (<=) instead of equals. let { x, y } <= somObj; I know EXACTLY what that's doing, intuitively, as opposed to let { x, y } = somObj; which I look at and go "wtf"
Comments are closed for this article.

IMAGES
VIDEO
COMMENTS
For your 2nd issue, it's a typo, you're passing an object and y is a property, so it should be in:fly={{y: -200, .. No clue about your default export issue however, I've never had to explicitly export components.
For both object and array destructuring, there are two kinds of destructuring patterns: binding pattern and assignment pattern, with slightly different syntaxes. In binding patterns, the pattern starts with a declaration keyword (var, let, or const). Then, each individual property must either be bound to a variable or further destructured.
bug: Module parse failed: Shorthand property assignments are valid only in destructuring patterns #452. Closed DiegoCantu opened this issue Jun 23, ... Module parse failed: Shorthand property assignments are valid only in destructuring patterns #452. DiegoCantu opened this issue Jun 23, 2023 · 15 comments Assignees. Labels.
The ( ..) around the assignment statement is required syntax when using object literal destructuring assignment without a declaration. {a, b} = {a: 1, b: 2} is not valid stand-alone syntax, as the {a, b} on the left-hand side is considered a block and not an object literal. However, ({a, b} = {a: 1, b: 2}) is valid, as is var {a, b} = {a: 1, b: 2} NOTE: Your ( ..) expression needs to be ...
📌 Shorthand Properties Shorthand Properties released in ES6 makes the construction of the object simpler because when we have it with a property and a value and both have the same value we can omit the "value" and just declare the property, see the example below: Old mode:
If an object or an array contains other nested objects and arrays, we can use more complex left-side patterns to extract deeper portions. In the code below options has another object in the property size and an array in the property items. The pattern on the left side of the assignment has the same structure to extract values from them:
// ⛔️ Uncaught SyntaxError: Invalid destructuring assignment target const obj = {name: 'Tom'}, {name: 'John'} Instead, you should place the objects in an array or only assign a single value to the variable.
Destructuring patterns are syntactically similar to the literals that are used for multivalue construction. But they appear where data is received (e.g., at the left-hand sides of assignments), not where data is created (e.g., at the right-hand sides of assignments). ... If a property in an object pattern has no match on the right-hand side ...
However in case of shorthand property assignments: ... Also note that the same problem exists with the destructuring pattern of for-of loop (perhaps, due to the same reasons): var s; for ({s = 5} of q) {} ... parse/check/emit shorthand property assignment in destructuring microsoft/TypeScript 5 participants Footer
In the function signature for drawES2015Chart above, the destructured left-hand side is assigned to an empty object literal on the right-hand side: {size = 'big', coords = {x: 0, y: 0}, radius = 25} = {}.You could have also written the function without the right-hand side assignment. However, if you leave out the right-hand side assignment, the function will look for at least one argument to ...
The destructuring syntax is used for assigning object and array entries to their own variables. For instance, we can write the following code to assign array entries to individual variables: const [a, b] = [1, 2]; Then, the destructuring assignment syntax assigns the entries to the variables located in the same position.
Object Destructuring. We can use the destructuring assignment syntax for objects as well. For example, we can write: const {a,b} = {a:1, b:2}; In the code above, a is set to 1 and b is set to 2 because the key is matched to the name of the variable when assigning the values to variables. As we have a as the key and 1 as the corresponding value, the variable a is set to 1 because the key name ...
Property ShortHand : ColdFusion now provided a clean and concise way for defining Object Literals Properties. If a developer wants to define an Object where keys have the same name as the variables passed in, they can use the Property ShortHand Syntax. Syntax: myObject = ${.
The default assignment for object destructuring creates new variables with the same name as the object property. If you do not want the new variable to have the same name as the object property, you have the option of giving the new variable a different name by using a colon (:) to decide the name, as seen in the following example:
For both object and array destructuring, there are two kinds of destructuring patterns: binding pattern and assignment pattern, with slightly different syntaxes. In binding patterns, the pattern starts with a declaration keyword (var, let, or const). Then, each individual property must either be bound to a variable or further destructured.
What is destructuring assignment? Destructuring assignment allows you to assign the properties of an array or object to variables using syntax that looks similar to array or object literals. This syntax can be extremely terse, while still exhibiting more clarity than the traditional property access. Without destructuring assignment, you might ...
You will notice that instead of square brackets, object destructuring uses curly brackets to surround the variable declaration. In order to deconstruct more complex objects, such as the address data in the person object, you can nest the declaration of variables in line with the structure of the object. You prefix each block of variable destructuring with the name of the parent property and ...
Shorthand property assignments are valid only in destructuring patterns. vuejs2; destructuring; Share. Improve this question. ... How to destructure an Array while assigning the value to a Vue instance data property. Related questions. 4 ... Cannot Use Destructuring Assignment In Vuex Mutation.
The ({a}) you have as a LeftHandSideExpression is a ParenthesizedExpression, not an ObjectLiteral, and the parenthesis don't contain a simple assignment target. You probably are looking for a parenthesised statement to allow the destructuring pattern: var a = 10; ({a} = 0); 1: Surprisingly, (a) = 0; is a valid statement though.