- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
- How to Fix - UnboundLocalError: Local variable Referenced Before Assignment in Python
- How to use Pickle to save and load Variables in Python?
- How to fix Unresolved reference issue in PyCharm
- Unused variable in for loop in Python
- How to Access Dictionary Values in Python Using For Loop
- Python | Accessing variable value from code scope
- How to fix "SyntaxError: invalid character" in Python
- Assign Function to a Variable in Python
- How to Reference Elements in an Array in Python
- How to Store Values in Dictionary in Python Using For Loop
- How to Fix: SyntaxError: positional argument follows keyword argument in Python
- How To Fix Valueerror Exceptions In Python
- How To Fix - Python RuntimeWarning: overflow encountered in scalar
- Different Forms of Assignment Statements in Python
- Unused local variable in Python
- Access environment variable values in Python
- How to import variables from another file in Python?
- Python Program to Find and Print Address of Variable
- How To Fix Recursionerror In Python
- JavaScript ReferenceError - Can't access lexical declaration`variable' before initialization

How to Fix – UnboundLocalError: Local variable Referenced Before Assignment in Python
Developers often encounter the UnboundLocalError Local Variable Referenced Before Assignment error in Python. In this article, we will see what is local variable referenced before assignment error in Python and how to fix it by using different approaches.
What is UnboundLocalError: Local variable Referenced Before Assignment?
This error occurs when a local variable is referenced before it has been assigned a value within a function or method. This error typically surfaces when utilizing try-except blocks to handle exceptions, creating a puzzle for developers trying to comprehend its origins and find a solution.
Below, are the reasons by which UnboundLocalError: Local variable Referenced Before Assignment error occurs in Python :
Nested Function Variable Access
Global variable modification.
In this code, the outer_function defines a variable ‘x’ and a nested inner_function attempts to access it, but encounters an UnboundLocalError due to a local ‘x’ being defined later in the inner_function.
In this code, the function example_function tries to increment the global variable ‘x’, but encounters an UnboundLocalError since it’s treated as a local variable due to the assignment operation within the function.
Solution for Local variable Referenced Before Assignment in Python
Below, are the approaches to solve “Local variable Referenced Before Assignment”.
In this code, example_function successfully modifies the global variable ‘x’ by declaring it as global within the function, incrementing its value by 1, and then printing the updated value.
In this code, the outer_function defines a local variable ‘x’, and the inner_function accesses and modifies it as a nonlocal variable, allowing changes to the outer function’s scope from within the inner function.
Please Login to comment...
Similar reads.
- Python Errors
- Python How-to-fix
- Python Programs
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
[SOLVED] Local Variable Referenced Before Assignment
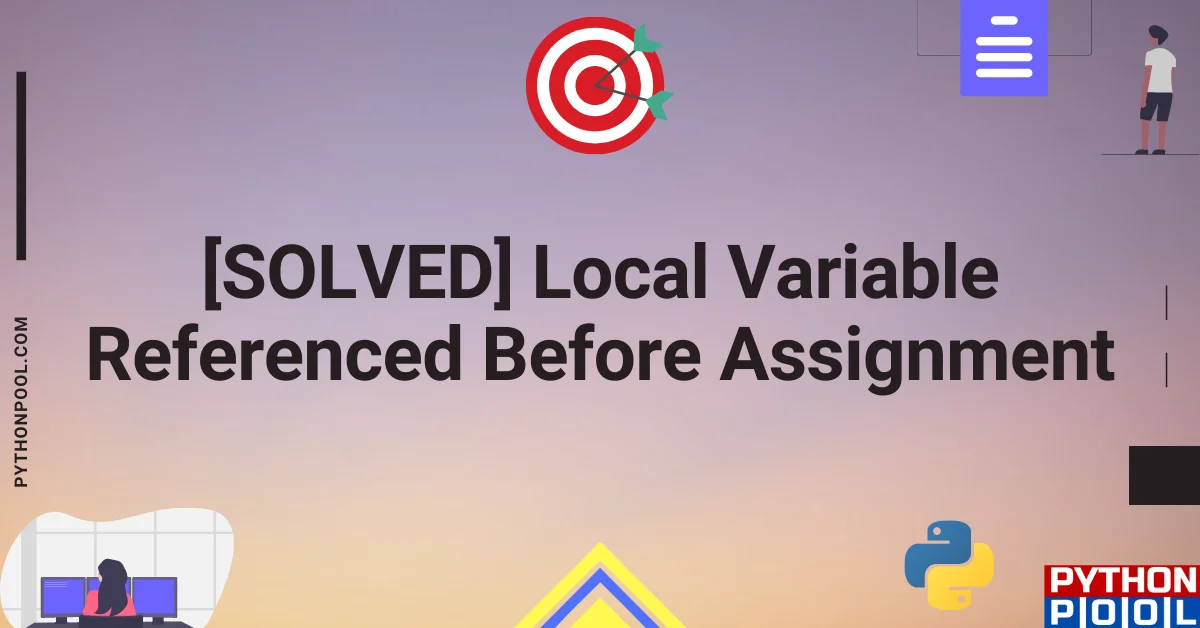
Python treats variables referenced only inside a function as global variables. Any variable assigned to a function’s body is assumed to be a local variable unless explicitly declared as global.
Why Does This Error Occur?
Unboundlocalerror: local variable referenced before assignment occurs when a variable is used before its created. Python does not have the concept of variable declarations. Hence it searches for the variable whenever used. When not found, it throws the error.
Before we hop into the solutions, let’s have a look at what is the global and local variables.
Local Variable Declarations vs. Global Variable Declarations
![unboundlocalerror local variable 'workbook' referenced before assignment [Fixed] typeerror can’t compare datetime.datetime to datetime.date](https://www.pythonpool.com/wp-content/uploads/2024/01/typeerror-cant-compare-datetime.datetime-to-datetime.date_-300x157.webp)
Local Variable Referenced Before Assignment Error with Explanation
Try these examples yourself using our Online Compiler.
Let’s look at the following function:

Explanation
The variable myVar has been assigned a value twice. Once before the declaration of myFunction and within myFunction itself.
Using Global Variables
Passing the variable as global allows the function to recognize the variable outside the function.
Create Functions that Take in Parameters
Instead of initializing myVar as a global or local variable, it can be passed to the function as a parameter. This removes the need to create a variable in memory.
UnboundLocalError: local variable ‘DISTRO_NAME’
This error may occur when trying to launch the Anaconda Navigator in Linux Systems.
Upon launching Anaconda Navigator, the opening screen freezes and doesn’t proceed to load.
Try and update your Anaconda Navigator with the following command.
If solution one doesn’t work, you have to edit a file located at
After finding and opening the Python file, make the following changes:
In the function on line 159, simply add the line:
DISTRO_NAME = None
Save the file and re-launch Anaconda Navigator.
DJANGO – Local Variable Referenced Before Assignment [Form]
The program takes information from a form filled out by a user. Accordingly, an email is sent using the information.
Upon running you get the following error:
We have created a class myForm that creates instances of Django forms. It extracts the user’s name, email, and message to be sent.
A function GetContact is created to use the information from the Django form and produce an email. It takes one request parameter. Prior to sending the email, the function verifies the validity of the form. Upon True , .get() function is passed to fetch the name, email, and message. Finally, the email sent via the send_mail function
Why does the error occur?
We are initializing form under the if request.method == “POST” condition statement. Using the GET request, our variable form doesn’t get defined.
Local variable Referenced before assignment but it is global
This is a common error that happens when we don’t provide a value to a variable and reference it. This can happen with local variables. Global variables can’t be assigned.
This error message is raised when a variable is referenced before it has been assigned a value within the local scope of a function, even though it is a global variable.
Here’s an example to help illustrate the problem:
In this example, x is a global variable that is defined outside of the function my_func(). However, when we try to print the value of x inside the function, we get a UnboundLocalError with the message “local variable ‘x’ referenced before assignment”.
This is because the += operator implicitly creates a local variable within the function’s scope, which shadows the global variable of the same name. Since we’re trying to access the value of x before it’s been assigned a value within the local scope, the interpreter raises an error.
To fix this, you can use the global keyword to explicitly refer to the global variable within the function’s scope:
However, in the above example, the global keyword tells Python that we want to modify the value of the global variable x, rather than creating a new local variable. This allows us to access and modify the global variable within the function’s scope, without causing any errors.
Local variable ‘version’ referenced before assignment ubuntu-drivers
This error occurs with Ubuntu version drivers. To solve this error, you can re-specify the version information and give a split as 2 –
Here, p_name means package name.
With the help of the threading module, you can avoid using global variables in multi-threading. Make sure you lock and release your threads correctly to avoid the race condition.
When a variable that is created locally is called before assigning, it results in Unbound Local Error in Python. The interpreter can’t track the variable.
Therefore, we have examined the local variable referenced before the assignment Exception in Python. The differences between a local and global variable declaration have been explained, and multiple solutions regarding the issue have been provided.
Trending Python Articles
![unboundlocalerror local variable 'workbook' referenced before assignment [Fixed] nameerror: name Unicode is not defined](https://www.pythonpool.com/wp-content/uploads/2024/01/Fixed-nameerror-name-Unicode-is-not-defined-300x157.webp)
Fixing Python UnboundLocalError: Local Variable ‘x’ Accessed Before Assignment
Understanding unboundlocalerror.
The UnboundLocalError in Python occurs when a function tries to access a local variable before it has been assigned a value. Variables in Python have scope that defines their level of visibility throughout the code: global scope, local scope, and nonlocal (in nested functions) scope. This error typically surfaces when using a variable that has not been initialized in the current function’s scope or when an attempt is made to modify a global variable without proper declaration.
Solutions for the Problem
To fix an UnboundLocalError, you need to identify the scope of the problematic variable and ensure it is correctly used within that scope.

Method 1: Initializing the Variable
Make sure to initialize the variable within the function before using it. This is often the simplest fix.
Method 2: Using Global Variables
If you intend to use a global variable and modify its value within a function, you must declare it as global before you use it.
Method 3: Using Nonlocal Variables
If the variable is defined in an outer function and you want to modify it within a nested function, use the nonlocal keyword.
That’s it. Happy coding!
Next Article: Fixing Python TypeError: Descriptor 'lower' for 'str' Objects Doesn't Apply to 'dict' Object
Previous Article: Python TypeError: write() argument must be str, not bytes
Series: Common Errors in Python and How to Fix Them
Related Articles
- Python Warning: Secure coding is not enabled for restorable state
- 4 ways to install Python modules on Windows without admin rights
- Python TypeError: object of type ‘NoneType’ has no len()
- Python: How to access command-line arguments (3 approaches)
- Understanding ‘Never’ type in Python 3.11+ (5 examples)
- Python: 3 Ways to Retrieve City/Country from IP Address
- Using Type Aliases in Python: A Practical Guide (with Examples)
- Python: Defining distinct types using NewType class
- Using Optional Type in Python (explained with examples)
- Python: How to Override Methods in Classes
- Python: Define Generic Types for Lists of Nested Dictionaries
- Python: Defining type for a list that can contain both numbers and strings
Search tutorials, examples, and resources
- PHP programming
- Symfony & Doctrine
- Laravel & Eloquent
- Tailwind CSS
- Sequelize.js
- Mongoose.js
Explore your training options in 10 minutes Get Started
- Graduate Stories
- Partner Spotlights
- Bootcamp Prep
- Bootcamp Admissions
- University Bootcamps
- Coding Tools
- Software Engineering
- Web Development
- Data Science
- Tech Guides
- Tech Resources
- Career Advice
- Online Learning
- Internships
- Apprenticeships
- Tech Salaries
- Associate Degree
- Bachelor's Degree
- Master's Degree
- University Admissions
- Best Schools
- Certifications
- Bootcamp Financing
- Higher Ed Financing
- Scholarships
- Financial Aid
- Best Coding Bootcamps
- Best Online Bootcamps
- Best Web Design Bootcamps
- Best Data Science Bootcamps
- Best Technology Sales Bootcamps
- Best Data Analytics Bootcamps
- Best Cybersecurity Bootcamps
- Best Digital Marketing Bootcamps
- Los Angeles
- San Francisco
- Browse All Locations
- Digital Marketing
- Machine Learning
- See All Subjects
- Bootcamps 101
- Full-Stack Development
- Career Changes
- View all Career Discussions
- Mobile App Development
- Cybersecurity
- Product Management
- UX/UI Design
- What is a Coding Bootcamp?
- Are Coding Bootcamps Worth It?
- How to Choose a Coding Bootcamp
- Best Online Coding Bootcamps and Courses
- Best Free Bootcamps and Coding Training
- Coding Bootcamp vs. Community College
- Coding Bootcamp vs. Self-Learning
- Bootcamps vs. Certifications: Compared
- What Is a Coding Bootcamp Job Guarantee?
- How to Pay for Coding Bootcamp
- Ultimate Guide to Coding Bootcamp Loans
- Best Coding Bootcamp Scholarships and Grants
- Education Stipends for Coding Bootcamps
- Get Your Coding Bootcamp Sponsored by Your Employer
- GI Bill and Coding Bootcamps
- Tech Intevriews
- Our Enterprise Solution
- Connect With Us
- Publication
- Reskill America
- Partner With Us
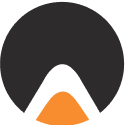
- Resource Center
- Bachelor’s Degree
- Master’s Degree
Python local variable referenced before assignment Solution
When you start introducing functions into your code, you’re bound to encounter an UnboundLocalError at some point. This error is raised when you try to use a variable before it has been assigned in the local context .
In this guide, we talk about what this error means and why it is raised. We walk through an example of this error in action to help you understand how you can solve it.
Find your bootcamp match
What is unboundlocalerror: local variable referenced before assignment.
Trying to assign a value to a variable that does not have local scope can result in this error:
Python has a simple rule to determine the scope of a variable. If a variable is assigned in a function , that variable is local. This is because it is assumed that when you define a variable inside a function you only need to access it inside that function.
There are two variable scopes in Python: local and global. Global variables are accessible throughout an entire program; local variables are only accessible within the function in which they are originally defined.
Let’s take a look at how to solve this error.
An Example Scenario
We’re going to write a program that calculates the grade a student has earned in class.
We start by declaring two variables:
These variables store the numerical and letter grades a student has earned, respectively. By default, the value of “letter” is “F”. Next, we write a function that calculates a student’s letter grade based on their numerical grade using an “if” statement :
Finally, we call our function:
This line of code prints out the value returned by the calculate_grade() function to the console. We pass through one parameter into our function: numerical. This is the numerical value of the grade a student has earned.
Let’s run our code and see what happens:
An error has been raised.
The Solution
Our code returns an error because we reference “letter” before we assign it.
We have set the value of “numerical” to 42. Our if statement does not set a value for any grade over 50. This means that when we call our calculate_grade() function, our return statement does not know the value to which we are referring.
We do define “letter” at the start of our program. However, we define it in the global context. Python treats “return letter” as trying to return a local variable called “letter”, not a global variable.
We solve this problem in two ways. First, we can add an else statement to our code. This ensures we declare “letter” before we try to return it:
Let’s try to run our code again:
Our code successfully prints out the student’s grade.
If you are using an “if” statement where you declare a variable, you should make sure there is an “else” statement in place. This will make sure that even if none of your if statements evaluate to True, you can still set a value for the variable with which you are going to work.
Alternatively, we could use the “global” keyword to make our global keyword available in the local context in our calculate_grade() function. However, this approach is likely to lead to more confusing code and other issues. In general, variables should not be declared using “global” unless absolutely necessary . Your first, and main, port of call should always be to make sure that a variable is correctly defined.
In the example above, for instance, we did not check that the variable “letter” was defined in all use cases.
That’s it! We have fixed the local variable error in our code.
The UnboundLocalError: local variable referenced before assignment error is raised when you try to assign a value to a local variable before it has been declared. You can solve this error by ensuring that a local variable is declared before you assign it a value.
Now you’re ready to solve UnboundLocalError Python errors like a professional developer !
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication .
What's Next?

Get matched with top bootcamps
Ask a question to our community, take our careers quiz.
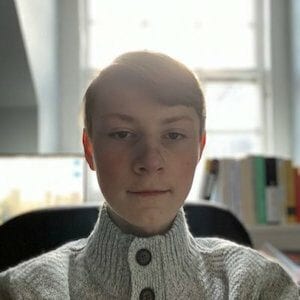
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
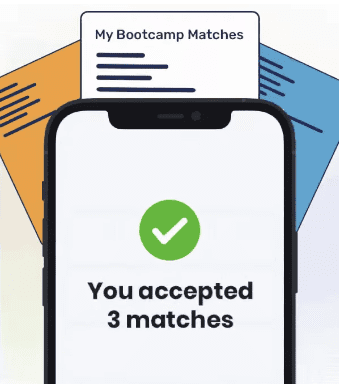
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
UnboundLocalError: local variable 'classes' referenced before assignment #705
baditaflorin commented Jan 9, 2019 • edited by tomchadwin
tomchadwin commented Jan 9, 2019
Sorry, something went wrong.
tomchadwin commented Feb 12, 2019
- 👍 1 reaction
HueyNemud commented Apr 12, 2019 • edited
kaplanlior commented Apr 15, 2019
Tomchadwin commented jun 26, 2019.
DanTimmermann commented Jul 7, 2019
Tomchadwin commented jul 8, 2019, dantimmermann commented jul 8, 2019.
UnderJollyRoger commented Apr 26, 2020 • edited
mypitaya commented Jul 26, 2021
Tomchadwin commented jul 26, 2021, mypitaya commented jul 26, 2021 via email.
No branches or pull requests

IMAGES
VIDEO
COMMENTS
Output. Hangup (SIGHUP) Traceback (most recent call last): File "Solution.py", line 7, in <module> example_function() File "Solution.py", line 4, in example_function x += 1 # Trying to modify global variable 'x' without declaring it as global UnboundLocalError: local variable 'x' referenced before assignment Solution for Local variable Referenced Before Assignment in Python
File "weird.py", line 5, in main. print f(3) UnboundLocalError: local variable 'f' referenced before assignment. Python sees the f is used as a local variable in [f for f in [1, 2, 3]], and decides that it is also a local variable in f(3). You could add a global f statement: def f(x): return x. def main():
Local Variables Global Variables; A local variable is declared primarily within a Python function.: Global variables are in the global scope, outside a function. A local variable is created when the function is called and destroyed when the execution is finished.
2 Solutions for the Problem. 2.1 Method 1: Initializing the Variable. 2.2 Method 2: Using Global Variables. 2.3 Method 3: Using Nonlocal Variables.
Trying to assign a value to a variable that does not have local scope can result in this error: UnboundLocalError: local variable referenced before assignment. Python has a simple rule to determine the scope of a variable. If a variable is assigned in a function, that variable is local. This is because it is assumed that when you define a ...
To prevent UnboundLocalError, the secret is in scope declaration. Declare a variable as global within a function if you're modifying a global variable. Alternatively, use nonlocal for variables in nested functions. python Global variable fix def func(): global var Hello, Global! var = 1 Changed it, see? Nested function fix def outer(): var = 0 def inner(): nonlocal var Outer! Lemme borrow this ...
UnboundLocalError: local variable '_' referenced before assignment #423. ChristophSchranz opened this issue Feb 22 ... self.log.warning(_('No web browser found: %s.') % e) gpu-jupyter_1 | UnboundLocalError: local variable '_' referenced before assignment Should this line work? The text was updated successfully, but these errors were encountered
@catskillsresearch: The external file you link to has too many dependencies to try to run it here.And even then, we don't know which input has been used. It would help if you'd inspect the values and datatypes of is_returns, is_weekly, is_monthly and kwargs, and based on those try to create a stand-alone example.Can you verify whether my tests below coincide with your case?
Another UnboundLocalError: local variable referenced before assignment Issue 2 global var becomes local --UnboundLocalError: local variable referenced before assignment
UnboundLocalError: local variable 'a' referenced before assignment The text was updated successfully, but these errors were encountered: 👍 17 callensm, bendesign55, nulladdict, rohanbanerjee, balovbohdan, drewszurko, hellotuitu, rribani, jeromesteve202, egmaziero, and 7 more reacted with thumbs up emoji
I have following simple function to get percent values for different cover types from a raster. It gives me following error: UnboundLocalError: local variable 'a' referenced before assignment whic...
How often? 33 Traceback (most recent call last): File "main.py", line 20, in <module> main() File "main.py", line 17, in main won += 1 UnboundLocalError: local variable 'won' referenced before assignment when I try to run the following code:
UnboundLocalError: local variable 'torch' referenced before assignment The text was updated successfully, but these errors were encountered: All reactions
When trying to 'Geocode' a CSV file I receive this answer: UnboundLocalError: local variable 'attributes' referenced before assignment Traceback (most recent call last): File "/home/user/.local/ ... UnboundLocalError: local variable 'attributes' referenced before assignment. Traceback (most recent call last):
I was advised to make a separate python library and then import it. After reading a bit more on Stackoverflow, I realized that the best way is to write methods and I've taken up that path. def USB(port): activateme = serial.Serial(port,115200) #print "starting to monitor". for line in activateme: #print line.
UnboundLocalError: local variable packed referenced before assignment #32506. Closed gehring opened this issue Sep 13, 2019 · 4 comments Closed ... packed) UnboundLocalError: local variable 'packed' referenced before assignment ...
UnboundLocalError: local variable 'item' referenced before assignment (python, flask) 0. UnboundLocalError: local variable 'filename' referenced before assignment ... UnboundLocalError: local variable referenced before assignment # Hot Network Questions Looking for a Star Wars Legends novel where Gamorreans were used as guinea pigs
@arunmallya I opened this new issue since you closed the last one as far as I have checked I haven't made and any changes to the directory structure it's as same as the one mentioned in the readme file and I haven't made any changes to t...
Saved searches Use saved searches to filter your results more quickly
Dear Tom, I hope you are doing well. I just followed the instruction from "YOUTUBE Tutorial of jdvkwast as an instructor of QGIS in IHE-UNESCO, Using the PCRaster within QGIS, there is a unique processing tool that is not available in GRASS nor in GDAL The problem is the tool does not come out in PCRaster Processing Tool, But it is in the Folders.