
Python Data Structures
Week 1 - assignment 6.5.
Write code using find() and string slicing to extract the number at the end of the line below. Convert the extracted value to a floating point number and print it out.
text = "X-DSPAM-Confidence: 0.8475";
text = "X-DSPAM-Confidence: 0.8475"
Colpos = text.find(':') # Colon Position
text_a_Colpos = text[Colpos+1 : ] # Text after colon position
number = text_a_Colpos.strip()
print(float(number))
ans = float(text_a_Colpos)
# Using Split and join functions
num_str = text_a_Colpos.split() # string format of number in list
d = ""
num = d.join(num_str) # converts list into string
num_f = float(num)
print(num_f)
=============================================================================================
Week 3 - Assignment 7.1
Write a program that prompts for a file name, then opens that file and reads through the file, and print the contents of the file in upper case. Use the file words.txt to produce the output below.
when you are testing below enter words.txt as the file name.
file = input('Enter the file name: ')
fhandle = open(file)
for line in fhandle:
line_strip = line.strip()
line = line_strip.upper()
print(line)
Assignment 7.2
Write a program that prompts for a file name, then opens that file and reads through the file, looking for lines of the form:
X-DSPAM-Confidence: 0.8475
Count these lines and extract the floating point values from each of the lines and compute the average of those values and produce an output as shown below. Do not use the sum() function or a variable named sum in your solution.
when you are testing below enter mbox-short.txt as the file name.
fname = input('Enter the file name: ')
fhandle = open(fname)
for line in fhandle :
if 'X-DSPAM-Confidence:' in line :
Colpos = line.find(':')
num_string = line[Colpos + 1 : ]
num = float(num_string)
count = count + 1
Total = Total + num
avg = Total / count
print('Average spam confidence:',avg)
===============================================================================================
Week 4 - Assignment 8.4
Open the file romeo.txt and read it line by line. For each line, split the line into a list of words using the split() method. The program should build a list of words. For each word on each line check to see if the word is already in the list and if not append it to the list. When the program completes, sort and print the resulting words in alphabetical order.
fhandle = open('romeo.txt')
lst = list()
words = line.split()
print(words)
for word in words:
if lst is None:
lst.append(word)
elif word in lst:
Assignment 8.5
Open the file mbox-short.txt and read it line by line. When you find a line that starts with 'From ' like the following line:
From [email protected] Sat Jan 5 09:14:16 2008
You will parse the From line using split() and print out the second word in the line (i.e. the entire address of the person who sent the message). Then print out a count at the end.
Hint: make sure not to include the lines that start with 'From:'.
if line.startswith('From') :
if line[4] is ':' :
req_line = line.split()
print(req_line[1])
print('There were',count, 'lines in the file with From as the first word')
==============================================================================================
Week 5 - Assignment 9.4
Write a program to read through the mbox-short.txt and figure out who has sent the greatest number of mail messages. The program looks for 'From ' lines and takes the second word of those lines as the person who sent the mail. The program creates a Python dictionary that maps the sender's mail address to a count of the number of times they appear in the file. After the dictionary is produced, the program reads through the dictionary using a maximum loop to find the most prolific committer.
reg_mailer = dict() # regular mailer
mail = words[1]
# reg_mailer[mail] = reg_mailer.get(mail,0) + 1
if reg_mailer is None or mail not in reg_mailer :
reg_mailer[mail] = 1
reg_mailer[mail] = reg_mailer[mail] + 1
a = max(reg_mailer.values())
for key, value in reg_mailer.items() :
if value == a :
print(key,a)
Week 6 - Assignment 10.2
Write a program to read through the mbox-short.txt and figure out the distribution by hour of the day for each of the messages.
You can pull the hour out from the 'From ' line by finding the time and then splitting the string a second time using a colon.
Once you have accumulated the counts for each hour, print out the counts, sorted by hour as shown below.
time_mail = dict()
time = words[5]
time_tup = time.split(':')
time_tuple = time_tup[0]
time_mail[time_tuple] = time_mail.get(time_tuple,0) + 1
# if reg_mailer is None or mail not in reg_mailer :
# reg_mailer[mail] = 1
# reg_mailer[mail] = reg_mailer[mail] + 1
ans = sorted(time_mail.items())
for k,v in ans:
Search This Blog
Coursera python data structures assignment answers.
This course will introduce the core data structure of the Python programming ... And, the answer is only for hint . it is helpful for those student who haven't submit Assignment and who confused ... These five lines are the lines to do this particular assignment where we are ...
chapter 7 week 3 Assignment 7.1

Post a Comment
if you have any doubt , please write comment

Popular posts from this blog
Chapter 9 week 5 assignment 9.4.

chapter 10 week 6 Assignment 10.2

chapter 6 week 1 Assignment 6.5


assignment 7.1 python data structures
- python repetition structures
- mac big sur and python3 problems
- python 0-1 kanpsack
- logical operators python
- Python For Data Science And Machine Learning Bootcamp
- python practice problems
- class item assignment python
- assignment 4.6 python for everybody
- assignment 6.5 python for everybody
- cors assignment 4.6 python
- python data structures
- advanced python course
- python fundamentals assignment solutions pdf
- python data structures 9.4
- python beginner practice problems
- learn python the hard way pdf
- data structures and algorithms in python
- python for data science
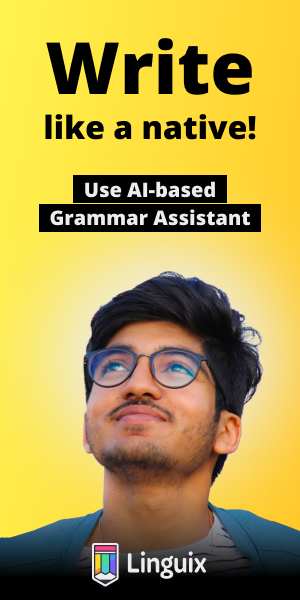
New to Communities?
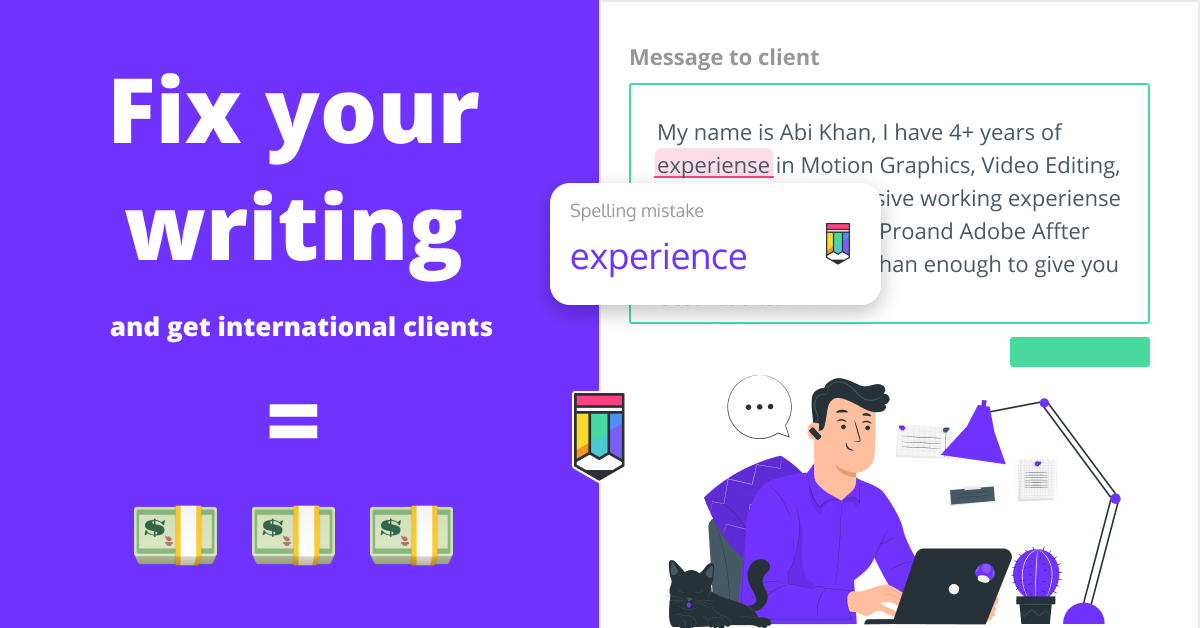
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Current repository contains all assignments, notes, quizzes and course materials from the "Python for Everybody Specialization" provided by Coursera and University of Michigan.
sersavn/coursera-python-for-everybody-specialization
Folders and files, repository files navigation, python for everybody specialization.
Coursera Python for Everybody specialization is offered by University of Michigan.
Specialization includes following courses:
- Getting started with python
- Python Data Structures
- Using python to Access Web Data
- Using Databases with Python
- Capstone: Retrieving Processing and Visualizing Data with Python
Each course described above contains assignments answers, course material and might contain some notes. Some course slides seemed to awesome and entartaining for me, so I could not resist adding all of them here 😃.
Additional materials
All additional course materials also can be found here . Also here is the link to the HTML version of Charles R. Severance book.
Incredible Lecturer
Without him existing on a planet I probably would never quit civil engineering and got interest in programming at all. Professor Charles Severance, University of Michigan.

- Python 93.6%
- JavaScript 5.3%

IMAGES
VIDEO
COMMENTS
My solutions to all quizzes and assignments for Python Data Structures on coursera by the University of Michigan - Coursera-Python-Data-Structures/Week 3 Assignment 7.1-solution.py at master · pavanayila/Coursera-Python-Data-Structures
Python Data Structures Assignment 7.1 Solution [Coursera] | Assignment 7.1 Python Data StructuresCoursera: Programming For Everybody Assignment 7.1 program s...
Contribute to riya2905/Coursera-PY4E-Python-Data-Structures development by creating an account on GitHub.
I'm working on assignment 7.1 on the Python for Everybody specialisation from coursera. The assignment is the following: "Write a program that prompts for a file name, then opens that file and reads through the file, and print the contents of the file in upper case. Use the file words.txt to produce the output below.
This course will introduce the core data structures of the Python programming language. We will move past the basics of procedural programming and explore how we can use the Python built-in data structures such as lists, dictionaries, and tuples to perform increasingly complex data analysis. This course will cover Chapters 6-10 of the textbook ...
Python Data Structures Assignment 7.1 Solution [Coursera] | Assignment 7.1 Python Data StructuresPython Data Structures Assignment 7.1 Solution [Coursera] | ...
About Press Copyright Contact us Creators Advertise Developers Terms Privacy Policy & Safety How YouTube works Test new features NFL Sunday Ticket Press Copyright ...
Contribute to Ritik2703/Coursera---Python-Data-Structure-Answers development by creating an account on GitHub.
python-for-everybody. /. wk7 - assignment 7.1.py. Cannot retrieve latest commit at this time. History. Code. Blame. 12 lines (9 loc) · 448 Bytes. 7.1 Write a program that prompts for a file name, then opens that file and reads through the file, and print the contents of the file in upper case.
Assignment 7.2. Write a program that prompts for a file name, then opens that file and reads through the file, looking for lines of the form: X-DSPAM-Confidence: 0.8475. Count these lines and extract the floating point values from each of the lines and compute the average of those values and produce an output as shown below.
This course will introduce the core data structures of the Python programming language. We will move past the basics of procedural programming and explore how we can use the Python built-in data structures such as lists, dictionaries, and tuples to perform increasingly complex data analysis. This course will cover Chapters 6-10 of the textbook ...
..Welcome to #mythoughtsPYTHON DATA STRUCTURES Assignment 7.1 [coursera] | Assignment 7.1 in PYHTON DATA STRUCTURES |Assignment 7.1 in PY4E |
chapter 6 week 1 Assignment 6.5 Tuesday, June 16, 2020 6.5 Write code using find() and string slicing (see section 6.10) to extract the number at the end of the line below.
This class provides an introduction to the Python programming language and the iPython notebook. This is the third course in the Genomic Big Data Science Specialization from Johns Hopkins University. Week One. Module 1 • 2 hours to complete. This week we will have an overview of Python and take the first steps towards programming.
{"payload":{"allShortcutsEnabled":false,"fileTree":{"Week-3":{"items":[{"name":"Assignment7.1.py","path":"Week-3/Assignment7.1.py","contentType":"file"},{"name ...
The above video consists of the images of the Assignment 7.1 and 7.2 of the course Python for everybody, the code was programmed on the auto-grader whose scr...
There are 9 modules in this course. This course focuses on developing Python skills for assembling business data. It will cover some of the same material from Introduction to Accounting Data Analytics and Visualization, but in a more general purpose programming environment (Jupyter Notebook for Python), rather than in Excel and the Visual Basic ...
Get code examples like"assignment 7.1 python data structures". Write more code and save time using our ready-made code examples.
{"payload":{"allShortcutsEnabled":false,"fileTree":{"":{"items":[{"name":".gitignore","path":".gitignore","contentType":"file"},{"name":"README.md","path":"README.md ...
# Coursera :- #python data structures# Python👇👇program run code 👇👇https://1drv.ms/w/s!AspSlroH33QifAdPaMMP5CG9bwECHAPTER :- PYTHON DATA STRUCTURESASSIGN...
Current repository contains all assignments, notes, quizzes and course materials from the "Python for Everybody Specialization" provided by Coursera and University of Michigan. - sersavn/coursera-python-for-everybody-specialization
There are 9 modules in this course. This course focuses on developing Python skills for assembling business data. It will cover some of the same material from Introduction to Accounting Data Analytics and Visualization, but in a more general purpose programming environment (Jupyter Notebook for Python), rather than in Excel and the Visual Basic ...
Hi everyone,This video is for education purpose onlylike share and subscribe for more videoPlease visit my Blog to see more contenthttps://priyadigitalworld....
There are 3 modules in this course. Our "Programming with Generative AI" course takes you on a practical journey, exploring how generative AI tools can transform your coding workflow. Whether you're a software developer, tech lead, or AI enthusiast, this hands-on program is designed for you. Learn by doing: - Dive deep into GitHub Copilot, the ...