Java default Initialization of Instance Variables and Initialization Blocks
1. default initialization of instance variables in java.
- Integer numbers have default value: 0
- for int type: 0
- for byte type: (byte) 0
- for short type: (short) 0
- for long type: 0L
- Floating point numbers have default value: 0.0
- for float type: 0.0f
- for double type: 0.0d
- Boolean variables have default value: false
- Character variables have default value: ‘\u0000’
- Object references have default value: null

2. Default Initialization of Arrays in Java
3. instance initialization blocks in java, 4. static initialization blocks in java, other recommended tutorials:.
- 9 Rules about Constructors in Java
- 12 Rules and Examples About Inheritance in Java
- 12 Rules of Overriding in Java You Should Know
- 10 Java Core Best Practices Every Java Programmer Should Know
- Understand Interfaces in Java
- Understand how variables are passed in Java
- Understand access modifiers in Java
- Understand encapsulation in Java
About the Author:

Add comment
Notify me of follow-up comments
Comments
Java, JavaScript & Web tutorials
Does Java have default parameters?
Short answer: No. Fortunately, you can simulate them. Many programming languages like C++ or modern JavaScript have a simple option to call a function without providing values for its arguments. In Java, default method parameters require a bit more typing to achieve this effect in comparison to other languages. From this article, you will learn how the default method parameters work in Java .
1. Java default parameters in practice
The syntax of Java language doesn’t allow you to declare a method with a predefined value for a parameter. Fortunately, you can achieve the same effect with simple code constructions.
There are several options that you may use to simulate behavior known from other programming languages. You can:
- use method overloading
- allow nulls as an input
- declare a method with Java Varargs
Let’s take a closer look at these options.
1.1. Java default parameters with method overloading
Probably the best option to achieve default method parameters in Java is by using the method overloading. Method overloading allows you to declare several methods with the same name but with a different number of parameters.
How to use method overloading to simulate default method parameters?
By calling a more complex method by a simpler one.
Here is a practical example of a method which allows you to query for an optional number of some documents:
The only required parameter is a query string. As you can see, you only have to implement the method with the highest number of parameters. To clarify, t he simple method is just a proxy that passes its parameter to the more complex method and provides default parameter values for the optional arguments.
In comparison to other options, the main advantage of method overloading is the simplicity for the client code. You can easily read what set of parameters is allowed for a given method.
Although method overloading is strongly recommended, it’s worth knowing about other possibilities.
1.2. Allowing Nulls as method parameters
Another option is to declare a single method that accepts all possible parameters. Next, in the body of the method, you can check which parameters are nulls and assign them default values.
In this approach, our previous example looks as follows:
There are two main disadvantages to this approach. First, you still need to pass all arguments in client code. Even if some of them are nulls. Second, you need to know which parameters are nullable as you can’t tell that just by looking at the method declaration.
What is more, many developers consider reassinging method parameters as bad practice as it makes the code harder to follow. With this in mind, many static code analysis tools allow you to check for this kind of code smell.
Some people suggest wrapping parameters which aren’t required inside Java 8 Optional class. However, the Optional type was designed for method outputs and not inputs. If you are interested why, check out my other article about Java Optional use cases .
1.3. Varargs parameter
The Varargs option is limited only to arguments of the same type and meaning. Therefore, it doesn’t actually solve the problem of default parameters in Java methods. But you may consider it in some cases.
Technically, it’s even possible to use Varargs as a single optional parameter. In this case, you don’t have to pass null in the client code to use the default value. Yet, it rather feels like an awful hack. I recommend sticking to method overloading.
2. Java default parameters in long parameter list
The main problem with method overloading as a solution for default parameter values reveals itself when a method accepts multiple parameters. Creating an overloaded method for each possible combination of parameters might be cumbersome.
To deal with this issue, you should introduce the Parameter Object pattern .
What is it?
Simply put, the Parameter Object is a wrapper object for all parameters of a method.
But wait! Aren’t we just moving the problem of the long parameter list from a method to the constructor of the new class?
Indead, we are. But class constructors give us one additional solution for the problem which is the Builder pattern.
2.1. Solving Java default parameters with Parameter Object
As mentioned before, the first thing you need is a parameter object class that wraps the list of method parameters. Let’s see how it can look like for a method from the previous example:
As you can see, the implemented parameter object is nothing more than just a regular POJO. The advantage of the Parameter Object over a regular method parameter list is the fact that class fields can have default values . Just like in the above example.
That’s it. Now you have all default parameters in a single place.
2.2. … and Builder patterns
Once you create a wrapper class for the method parameter list you should also create a corresponding builder class. Usually, you’ll do it as an inner static class.
But don’t type builders alone, automate this work!
Popular Java IDEs provide plugins that generate builder classes.
In our example the builder will look as follows:
The final step is to use the builder to construct a new parameter object. In this case, you assign only selected parameters. For those parameters you skip, their default values are going to be used. Here’s an example:
Our example is really simple as the method has only three parameters so it might look like overengineering. In fact, the solution for default parameters using Parameter Object and Builder shines when the list of method parameters is much longer.
Let’s sum up.
Java doesn’t have a simple solution for default method parameters as available in other common programming languages. Yet, you can simulate it using other Java constructions and patterns. In addition, you learn a simple approach with method overloading which works for methods with a short parameter list. For complex methods, the Parameter Object pattern is more flexible.
If you find the article useful, please share it with your friends. Don’t forget to subscribe so I can notify you about other similar posts.
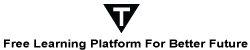
Java Tutorial
Control statements, java object class, java inheritance, java polymorphism, java abstraction, java encapsulation, java oops misc.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.
Primitive Data Types
The Java programming language is statically-typed, which means that all variables must first be declared before they can be used. This involves stating the variable's type and name, as you've already seen:
Doing so tells your program that a field named "gear" exists, holds numerical data, and has an initial value of "1". A variable's data type determines the values it may contain, plus the operations that may be performed on it. In addition to int , the Java programming language supports seven other primitive data types . A primitive type is predefined by the language and is named by a reserved keyword. Primitive values do not share state with other primitive values. The eight primitive data types supported by the Java programming language are:
byte : The byte data type is an 8-bit signed two's complement integer. It has a minimum value of -128 and a maximum value of 127 (inclusive). The byte data type can be useful for saving memory in large arrays , where the memory savings actually matters. They can also be used in place of int where their limits help to clarify your code; the fact that a variable's range is limited can serve as a form of documentation.
short : The short data type is a 16-bit signed two's complement integer. It has a minimum value of -32,768 and a maximum value of 32,767 (inclusive). As with byte , the same guidelines apply: you can use a short to save memory in large arrays, in situations where the memory savings actually matters.
int : By default, the int data type is a 32-bit signed two's complement integer, which has a minimum value of -2 31 and a maximum value of 2 31 -1. In Java SE 8 and later, you can use the int data type to represent an unsigned 32-bit integer, which has a minimum value of 0 and a maximum value of 2 32 -1. Use the Integer class to use int data type as an unsigned integer. See the section The Number Classes for more information. Static methods like compareUnsigned , divideUnsigned etc have been added to the Integer class to support the arithmetic operations for unsigned integers.
long : The long data type is a 64-bit two's complement integer. The signed long has a minimum value of -2 63 and a maximum value of 2 63 -1. In Java SE 8 and later, you can use the long data type to represent an unsigned 64-bit long, which has a minimum value of 0 and a maximum value of 2 64 -1. Use this data type when you need a range of values wider than those provided by int . The Long class also contains methods like compareUnsigned , divideUnsigned etc to support arithmetic operations for unsigned long.
float : The float data type is a single-precision 32-bit IEEE 754 floating point. Its range of values is beyond the scope of this discussion, but is specified in the Floating-Point Types, Formats, and Values section of the Java Language Specification. As with the recommendations for byte and short , use a float (instead of double ) if you need to save memory in large arrays of floating point numbers. This data type should never be used for precise values, such as currency. For that, you will need to use the java.math.BigDecimal class instead. Numbers and Strings covers BigDecimal and other useful classes provided by the Java platform.
double : The double data type is a double-precision 64-bit IEEE 754 floating point. Its range of values is beyond the scope of this discussion, but is specified in the Floating-Point Types, Formats, and Values section of the Java Language Specification. For decimal values, this data type is generally the default choice. As mentioned above, this data type should never be used for precise values, such as currency.
boolean : The boolean data type has only two possible values: true and false . Use this data type for simple flags that track true/false conditions. This data type represents one bit of information, but its "size" isn't something that's precisely defined.
char : The char data type is a single 16-bit Unicode character. It has a minimum value of '\u0000' (or 0) and a maximum value of '\uffff' (or 65,535 inclusive).
In addition to the eight primitive data types listed above, the Java programming language also provides special support for character strings via the java.lang.String class. Enclosing your character string within double quotes will automatically create a new String object; for example, String s = "this is a string"; . String objects are immutable , which means that once created, their values cannot be changed. The String class is not technically a primitive data type, but considering the special support given to it by the language, you'll probably tend to think of it as such. You'll learn more about the String class in Simple Data Objects
Default Values
It's not always necessary to assign a value when a field is declared. Fields that are declared but not initialized will be set to a reasonable default by the compiler. Generally speaking, this default will be zero or null , depending on the data type. Relying on such default values, however, is generally considered bad programming style.
The following chart summarizes the default values for the above data types.
Local variables are slightly different; the compiler never assigns a default value to an uninitialized local variable. If you cannot initialize your local variable where it is declared, make sure to assign it a value before you attempt to use it. Accessing an uninitialized local variable will result in a compile-time error.
You may have noticed that the new keyword isn't used when initializing a variable of a primitive type. Primitive types are special data types built into the language; they are not objects created from a class. A literal is the source code representation of a fixed value; literals are represented directly in your code without requiring computation. As shown below, it's possible to assign a literal to a variable of a primitive type:
Integer Literals
An integer literal is of type long if it ends with the letter L or l ; otherwise it is of type int . It is recommended that you use the upper case letter L because the lower case letter l is hard to distinguish from the digit 1 .
Values of the integral types byte , short , int , and long can be created from int literals. Values of type long that exceed the range of int can be created from long literals. Integer literals can be expressed by these number systems:
- Decimal: Base 10, whose digits consists of the numbers 0 through 9; this is the number system you use every day
- Hexadecimal: Base 16, whose digits consist of the numbers 0 through 9 and the letters A through F
- Binary: Base 2, whose digits consists of the numbers 0 and 1 (you can create binary literals in Java SE 7 and later)
For general-purpose programming, the decimal system is likely to be the only number system you'll ever use. However, if you need to use another number system, the following example shows the correct syntax. The prefix 0x indicates hexadecimal and 0b indicates binary:
Floating-Point Literals
A floating-point literal is of type float if it ends with the letter F or f ; otherwise its type is double and it can optionally end with the letter D or d .
The floating point types ( float and double ) can also be expressed using E or e (for scientific notation), F or f (32-bit float literal) and D or d (64-bit double literal; this is the default and by convention is omitted).
Character and String Literals
Literals of types char and String may contain any Unicode (UTF-16) characters. If your editor and file system allow it, you can use such characters directly in your code. If not, you can use a "Unicode escape" such as '\u0108' (capital C with circumflex), or "S\u00ED Se\u00F1or" (Sí Señor in Spanish). Always use 'single quotes' for char literals and "double quotes" for String literals. Unicode escape sequences may be used elsewhere in a program (such as in field names, for example), not just in char or String literals.
The Java programming language also supports a few special escape sequences for char and String literals: \b (backspace), \t (tab), \n (line feed), \f (form feed), \r (carriage return), \" (double quote), \' (single quote), and \\ (backslash).
There's also a special null literal that can be used as a value for any reference type. null may be assigned to any variable, except variables of primitive types. There's little you can do with a null value beyond testing for its presence. Therefore, null is often used in programs as a marker to indicate that some object is unavailable.
Finally, there's also a special kind of literal called a class literal , formed by taking a type name and appending " .class" ; for example, String.class . This refers to the object (of type Class ) that represents the type itself.
Using Underscore Characters in Numeric Literals
In Java SE 7 and later, any number of underscore characters ( _ ) can appear anywhere between digits in a numerical literal. This feature enables you, for example. to separate groups of digits in numeric literals, which can improve the readability of your code.
For instance, if your code contains numbers with many digits, you can use an underscore character to separate digits in groups of three, similar to how you would use a punctuation mark like a comma, or a space, as a separator.
The following example shows other ways you can use the underscore in numeric literals:
You can place underscores only between digits; you cannot place underscores in the following places:
- At the beginning or end of a number
- Adjacent to a decimal point in a floating point literal
- Prior to an F or L suffix
- In positions where a string of digits is expected
The following examples demonstrate valid and invalid underscore placements (which are highlighted) in numeric literals:
About Oracle | Contact Us | Legal Notices | Terms of Use | Your Privacy Rights
Copyright © 1995, 2022 Oracle and/or its affiliates. All rights reserved.
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
Default Values Assigned to Primitive Data Types in Java
- How to Get Size, Minimum, and Maximum Value of Data Types in Java?
- Primitive data type vs. Object data type in Java with Examples
- Assigning values to static final variables in Java
- Default Array Values in Java
- Java Program to Convert a Float value to String
- Why Java Collections Cannot Directly Store Primitives Types?
- Can We Override Default Method in Java?
- Java Program to Convert String to Float Value
- Is an array a primitive type or an object in Java?
- Default Methods In Java 8
- Static and non static blank final variables in Java
- Program to convert Primitive Array to Stream in Java
- TextStyle values() method in Java with Examples
- What are Primitive Data Types in JavaScript ?
- Primitive and Non-primitive data-types in JavaScript
- Uninitialized primitive data types in C/C++
- Golang Program that Uses Named Return Values and Defaults
- Is String a Primitive or a Derived Type?
- How to Assign Default Value for Struct Field in Golang?
In Java, when a variable is declared but not initialized, it is assigned a default value based on its data type. The default values for the primitive data types in Java are as follows:
byte: 0 short: 0 int: 0 long: 0L float: 0.0f double: 0.0d char: ‘\u0000’ (null character) boolean: false
It is important to note that these default values are only assigned if the variable is not explicitly initialized with a value. If a variable is initialized with a value, that value will be used instead of the default.
Primitive data types are built-in data types in java and can be used directly without using any new keyword. As we know primitive data types are treated differently by java cause of which the wrapper class concept also comes into play. But here we will be entirely focussing on data types. So, in java, there are 8 primitive data types as shown in the table below with their corresponding sizes.
Now, here default values are values assigned by the compiler to the variables which are declared but not initialized or given a value. They are different according to the return type of data type which is shown below where default values assigned to variables of different primitive data types are given in the table. However, relying on such default values is not considered a good programming style.
Now as we know Initializing a variable means to give an initial value to a variable before using it. So, in order to use the default values first, declare the variable with data type and name (eg, int x , here int is the data type and x is the name of the variable), if you don’t declare the variable before using it, it would result in a compile-time error. Now to use the default value of the variable do not initialize it, i.e. do not assign a value to it.
Output explanation:
Here ‘ a ‘ is a class member variable or you can say an instance variable and it will be initialized to its default value by the compiler.
Note: There would have been a problem if variable (‘a’) was not a class member as the compiler never assigns default values to an uninitialized local variable.
In this scenario, there will be an error pointing to variable ‘ a ‘ that variable ‘ a ‘ might not have been initialized yet. This is because here ‘ a ‘ is the main() method local variable and has to be initialized before being used. The compiler never assigns default values to an uninitialized local variable. If you haven’t initialized the variable where you have declared it, assign the variable a value before using it, or else it will result in a compile-time error.
It is as shown in the next example as shown below for a better understanding of the class variable.

Please Login to comment...
Similar reads.
- java-basics
- Java-Data Types
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Default Constructor in Java – Class Constructor Example
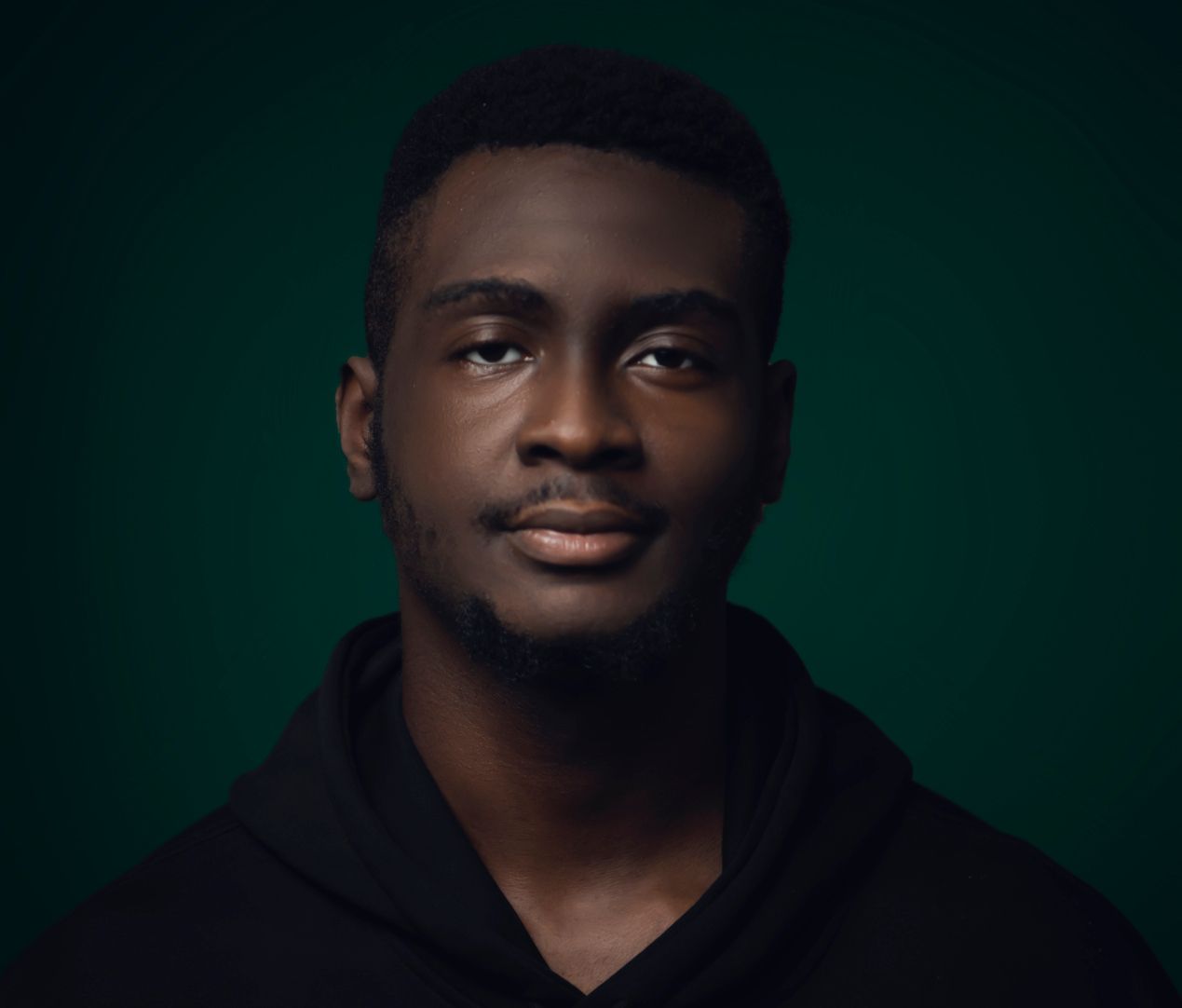
In this article, we will talk about constructors, how to create our own constructors, and what default constructors are in Java.
What is a constructor?
As a class-based object-oriented programming term, a constructor is a unique method used to initialize a newly created object (class). There are a few rules you must follow when creating constructors. These rules include:
- The name of the constructor must be the same as the class name.
- The constructor must have no return type.
Before we proceed, let's see what a class looks like in Java:
The code above shows a class called Student with three attributes – firstName , lastName , and age . We will assume that the class is supposed to be a sample for registering students. Recall that the three attributes do not have any values so none of the information is hard coded.
Now we will use constructors to create a new instance of our Student object. That is:
We have created a constructor which we used to initialize the attributes defined in the Student object. The code above is an example of a no-argument constructor. Let's see an example of a different kind now:
Now we have created a parameterized constructor . A parameterized constructor is a constructor created with arguments/parameters. Let's break it down.
We created a new constructor that takes in three arguments – two strings and an integer.
We then linked these arguments to the attributes we defined when we created our class. Now we have initialized the Student object using a constructor.
Lastly, we created a new instance of the Student object and passed in our arguments. We were able to pass in these arguments because we had already defined them in a constructor.
I created one constructor with three arguments, but you can also create separate constructors for initializing each attribute.
Now that you know what a constructor is in Java and how to use it, let's now look into default constructors.
What is a default constructor?
A default constructor is a constructor created by the compiler if we do not define any constructor(s) for a class. Here is an example:
Can you spot the difference between this and the two previous examples? Notice that we did not define any constructor before creating myStudent to initialize the attributes created in the class.
This will not throw an error our way. Rather, the compiler will create an empty constructor but you will not see this constructor anywhere in the code – this happens under the hood.
This is what the code above will look like when the compiler starts doing its job:
A lot of people mix up the default constructor for the no-argument constructor, but they are not the same in Java. Any constructor created by the programmer is not considered a default constructor in Java.
In this article, we learned what constructors are and how we can create and use them to initialize our objects.
We also talked about default constructors and what makes them different from no-argument constructors.
Happy Coding!
ihechikara.com
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
7 Best Java Homework Help Websites: How to Choose Your Perfect Match?
J ava programming is not a field that could be comprehended that easily; thus, it is no surprise that young learners are in search of programming experts to get help with Java homework and handle their assignments. But how to choose the best alternative when the number of proposals is enormous?
In this article, we are going to talk about the top ‘do my Java assignment’ services that offer Java assignment assistance and dwell upon their features. In the end, based on the results, you will be able to choose the one that meets your demands to the fullest and answer your needs. Here is the list of services that are available today, as well as those that are on everyone's lips:
TOP Java Assignment Help Services: What Makes Them Special?
No need to say that every person is an individual and the thing that suits a particular person could not meet the requirements of another. So, how can we name the best Java assignment help services on the web? - We have collected the top issues students face when searching for Java homework help and found the companies that promise to meet these requirements.
What are these issues, though?
- Pricing . Students are always pressed for budget, and finding services that suit their pockets is vital. Thus, we tried to provide services that are relatively affordable on the market. Of course, professional services can’t be offered too cheaply, so we have chosen the ones that balance professionalism and affordability.
- Programming languages . Not all companies have experts in all possible programming languages. Thus, we tried to choose the ones that offer as many different languages as possible.
- Expert staff . In most cases, students come to a company when they need to place their ‘do my Java homework’ orders ASAP. Thus, a large expert staff is a real benefit for young learners. They want to come to a service, place their order and get a professional to start working on their project in no time.
- Reviews . Of course, everyone wants to get professional help with Java homework from a reputable company that has already completed hundreds of Java assignments for their clients. Thus, we have mentioned only those companies that have earned enough positive feedback from their clients.
- Deadline options. Flexible deadline options are also a benefit for those who are placing their last-minute Java homework help assignments. Well, we also provide services with the most extended deadlines for those who want to save some money and place their projects beforehand.
- Guarantees . This is the must-feature if you want to get quality assistance and stay assured you are totally safe with the company you have chosen. In our list, we have only named companies that provide client-oriented guarantees and always keep their word, as well as offer only professional Java assignment experts.
- Customization . Every service from the list offers Java assistance tailored to clients’ personal needs. There, you won’t find companies that offer pre-completed projects and sell them at half-price.
So, let’s have a closer look at each option so you can choose the one that totally meets your needs.
DoMyAssignments.com
At company service, you can get assistance with academic writing as well as STEM projects. The languages you can get help with are C#, C++, Computer science, Java, Javascript, HTML, PHP, Python, Ruby, and SQL.
The company’s prices start at $30/page for a project that needs to be done in 14+ days.
Guarantees and extra services
The company offers a list of guarantees to make your cooperation as comfortable as possible. So, what can you expect from the service?
- Free revisions . When you get your order, you can ask your expert for revisions if needed. It means that if you see that any of your demands were missed, you can get revisions absolutely for free.
- Money-back guarantee. The company offers professional help, and they are sure about their experts and the quality of their assistance. Still, if you receive a project that does not meet your needs, you can ask for a full refund.
- Confidentiality guarantee . Stay assured that all your personal information is safe and secure, as the company scripts all the information you share with them.
- 100% customized assistance . At this service, you won’t find pre-written codes, all the projects are completed from scratch.

Expert staff
If you want to hire one of the top Java homework experts at DoMyAssignments , you can have a look at their profile, see the latest orders they have completed, and make sure they are the best match for your needs. Also, you can have a look at the samples presented on their website and see how professional their experts are. If you want to hire a professional who completed a particular sample project, you can also turn to a support team and ask if you can fire this expert.
CodingHomeworkHelp.org
CodingHomeworkHelp is rated at 9.61/10 and has 10+ years of experience in the programming assisting field. Here, you can get help with the following coding assignments: MatLab, Computer Science, Java, HTML, C++, Python, R Studio, PHP, JavaScript, and C#.
Free options all clients get
Ordering your project with CodingHomeworkHelp.org, you are to enjoy some other options that will definitely satisfy you.
- Partial payments . If you order a large project, you can pay for it in two parts. Order the first one, get it done, and only then pay for the second one.
- Revisions . As soon as you get your order, you can ask for endless revisions unless your project meets your initial requirements.
- Chat with your expert . When you place your order, you get an opportunity to chat directly with your coding helper. If you have any questions or demands, there is no need to first contact the support team and ask them to contact you to your assistant.
- Code comments . If you have questions concerning your code, you can ask your helper to provide you with the comments that will help you better understand it and be ready to discuss your project with your professor.
The prices start at $20/page if you set a 10+ days deadline. But, with CodingHomeworkHelp.org, you can get a special discount; you can take 20% off your project when registering on the website. That is a really beneficial option that everyone can use.
CWAssignments.com
CWAssignments.com is an assignment helper where you can get professional help with programming and calculations starting at $30/page. Moreover, you can get 20% off your first order.
Working with the company, you are in the right hands and can stay assured that the final draft will definitely be tailored to your needs. How do CWAssignments guarantee their proficiency?
- Money-back guarantee . If you are not satisfied with the final work, if it does not meet your expectations, you can request a refund.
- Privacy policy . The service collects only the data essential to complete your order to make your cooperation effective and legal.
- Security payment system . All the transactions are safe and encrypted to make your personal information secure.
- No AI-generated content . The company does not use any AI tools to complete their orders. When you get your order, you can even ask for the AI detection report to see that your assignment is pure.
With CWAssignments , you can regulate the final cost of your project. As it was mentioned earlier, the prices start at $30/page, but if you set a long-term deadline or ask for help with a Java assignment or with a part of your task, you can save a tidy sum.
DoMyCoding.com
This company has been offering its services on the market for 18+ years and provides assistance with 30+ programming languages, among which are Python, Java, C / C++ / C#, JavaScript, HTML, SQL, etc. Moreover, here, you can get assistance not only with programming but also with calculations.
Pricing and deadlines
With DoMyCoding , you can get help with Java assignments in 8 hours, and their prices start at $30/page with a 14-day deadline.
Guarantees and extra benefits
The service offers a number of guarantees that protect you from getting assistance that does not meet your requirements. Among the guarantees, you can find:
- The money-back guarantee . If your order does not meet your requirements, you will get a full refund of your order.
- Free edits within 7 days . After you get your project, you can request any changes within the 7-day term.
- Payments in parts . If you have a large order, you can pay for it in installments. In this case, you get a part of your order, check if it suits your needs, and then pay for the other part.
- 24/7 support . The service operates 24/7 to answer your questions as well as start working on your projects. Do not hesitate to use this option if you need to place an ASAP order.
- Confidentiality guarantee . The company uses the most secure means to get your payments and protects the personal information you share on the website to the fullest.
More benefits
Here, we also want to pay your attention to the ‘Samples’ section on the website. If you are wondering if a company can handle your assignment or you simply want to make sure they are professionals, have a look at their samples and get answers to your questions.
AssignCode.com
AssignCode is one of the best Java assignment help services that you can entrust with programming, mathematics, biology, engineering, physics, and chemistry. A large professional staff makes this service available to everyone who needs help with one of these disciplines. As with some of the previous companies, AssignCode.com has reviews on different platforms (Reviews.io and Sitejabber) that can help you make your choice.
As with all the reputed services, AssignCode offers guarantees that make their cooperation with clients trustworthy and comfortable. Thus, the company guarantees your satisfaction, confidentiality, client-oriented attitude, and authenticity.
Special offers
Although the company does not offer special prices on an ongoing basis, regular clients can benefit from coupons the service sends them via email. Thus, if you have already worked with the company, make sure to check your email before placing a new one; maybe you have received a special offer that will help you save some cash.
AssignmentShark.com
Reviews about this company you can see on different platforms. Among them are Reviews.io (4.9 out of 5), Sitejabber (4.5 points), and, of course, their own website (9.6 out of 10). The rate of the website speaks for itself.
Pricing
When you place your ‘do my Java homework’ request with AssignmentShark , you are to pay $20/page for the project that needs to be done in at least ten days. Of course, if the due date is closer, the cost will differ. All the prices are presented on the website so that you can come, input all the needed information, and get an approximate calculation.
Professional staff
On the ‘Our experts’ page, you can see the full list of experts. Or, you can use filters to see the professional in the required field.
The company has a quick form on its website for those who want to join their professional staff, which means that they are always in search of new experts to make sure they can provide clients with assistance as soon as the need arises.
Moreover, if one wants to make sure the company offers professional assistance, one can have a look at the latest orders and see how experts provide solutions to clients’ orders.
What do clients get?
Placing orders with the company, one gets a list of inclusive services:
- Free revisions. You can ask for endless revisions until your order fully meets your demands.
- Code comments . Ask your professional to provide comments on the codes in order to understand your project perfectly.
- Source files . If you need the list of references and source files your helper turned to, just ask them to add these to the project.
- Chat with the professional. All the issues can be solved directly with your coding assistant.
- Payment in parts. Large projects can be paid for in parts. When placing your order, let your manager know that you want to pay in parts.
ProgrammingDoer.com
ProgrammingDoer is one more service that offers Java programming help to young learners and has earned a good reputation among previous clients.
The company cherishes its reputation and does its best to let everyone know about their proficiency. Thus, you, as a client, can read what people think about the company on several platforms - on their website as well as at Reviews.io.
What do you get with the company?
Let’s have a look at the list of services the company offers in order to make your cooperation with them as comfortable as possible.
- Free revisions . If you have any comments concerning the final draft, you can ask your professional to revise it for free as many times as needed unless it meets your requirements to the fullest.
- 24/7 assistance . No matter when you realize that you have a programming assignment that should be done in a few days. With ProgrammingDoer, you can place your order 24/7 and get a professional helper as soon as there is an available one.
- Chat with the experts . When you place your order with the company, you get an opportunity to communicate with your coding helper directly to solve all the problems ASAP.
Extra benefits
If you are not sure if the company can handle your assignment the right way, if they have already worked on similar tasks, or if they have an expert in the needed field, you can check this information on your own. First, you can browse the latest orders and see if there is something close to the issue you have. Then, you can have a look at experts’ profiles and see if there is anyone capable of solving similar issues.
Can I hire someone to do my Java homework?
If you are not sure about your Java programming skills, you can always ask a professional coder to help you out. All you need is to find the service that meets your expectations and place your ‘do my Java assignment’ order with them.
What is the typical turnaround time for completing a Java homework assignment?
It depends on the service that offers such assistance as well as on your requirements. Some companies can deliver your project in a few hours, but some may need more time. But, you should mind that fast delivery is more likely to cost you some extra money.
What is the average pricing structure for Java assignment help?
The cost of the help with Java homework basically depends on the following factors: the deadline you set, the complexity level of the assignment, the expert you choose, and the requirements you provide.
How will we communicate and collaborate on my Java homework?
Nowadays, Java assignment help companies provide several ways of communication. In most cases, you can contact your expert via live chat on a company’s website, via email, or a messenger. To see the options, just visit the chosen company’s website and see what they offer.
Regarding the Author:
Nayeli Ellen, a dynamic editor at AcademicHelp, combines her zeal for writing with keen analytical skills. In her comprehensive review titled " Programming Assignment Help: 41 Coding Homework Help Websites ," Nayeli offers an in-depth analysis of numerous online coding homework assistance platforms.
What’s New for C++ Developers in Visual Studio 2022 17.10
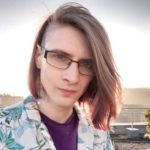
May 22nd, 2024 2 2
We are happy to announce that Visual Studio 2022 version 17.10 is now generally available! This post summarizes the new features you can find in this release for C++. You can download Visual Studio 2022 from the Visual Studio downloads page or upgrade your existing installation by following the Update Visual Studio Learn page .
Standard Library and Compiler
We have incremented the minor version number of the MSVC toolset from 19.39 (VS 2022 v17.9) to 19.40 (VS 2022 v17.10). For more details, and some ways in which this will affect projects that assume that MSVC versions are all 19.3X for Visual Studio 2022 releases, see the MSVC Toolset Minor Version Number 14.40 in VS 2022 v17.10 blog post .
We’ve made a host of changes to our standard library implementation, with the help of the community. As always, you can see the STL Changelog for full details. We implemented C++26’s P2510R3 Formatting Pointers , which brings the set of format specifiers for pointers when using std::format more in line with those that already exist for integers. We also implemented a few smaller features from C++26 and C++23, such as P2836R1 , which makes std::basic_const_iterator act in a more natural way with respect to implicit conversions. On the performance side, we improved the vectorized implementations of std::min_element , std::ranges::min and friends, made the copy/move assignment operators of std::expected trivial when expected, and more.
C++ Productivity and Game Development
You can now use Build Insights to view your template instantiation information. Template instantiation collection must be activated in Tools > Options > Build Insights.
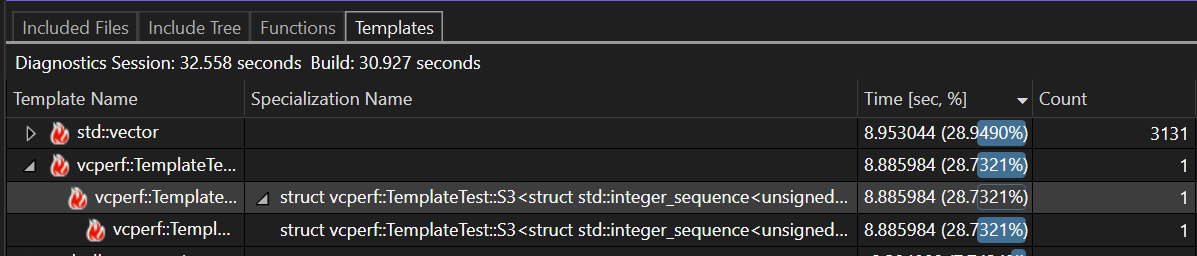
Check out our blog post on Templates View or our recording from Pure Virtual C++ for more details:
You can now keep our Unreal Engine plugin needed for Unreal Engine Test Adapter running in the background, greatly reducing startup costs. This is an opt-in feature that can be activated via Tools > Options > Unreal Engine. Furthermore, we have added additional Unreal Engine Macros to be indented in accordance with the UE Code Style.
Cross-Platform
If you’re targeting Linux, be sure to check out our video on our most recent development features for Linux from Pure Virtual C++:
CMake Targets View
We have added support for pinning CMake targets in the CMake Targets View. There is a top-level folder now for Pinned Targets. You can pin any targets by right-clicking and selecting the Pin option in the context menu.

You can also unpin any target in the Pinned Targets folder by selecting Unpin.
Connection Manager
We introduced some UX updates and usability improvements to the Connection Manager. With these updates we provide a more seamless experience when connecting to remote systems and/or debugging failed connections. Check out our blog post for more details.
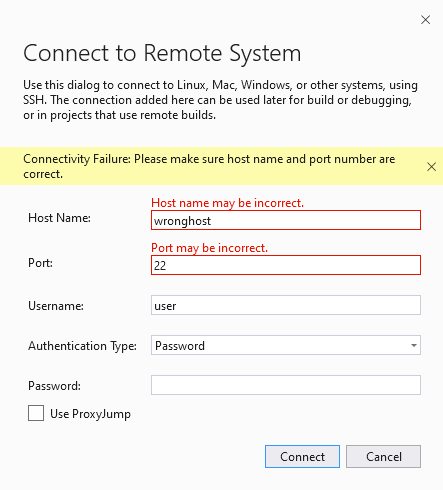
Core Editor
View and address pull request comments.
You can now view your GitHub and Azure DevOps pull request comments directly in your working file in Visual Studio. Enable the feature flag, “Pull Request Comments,” in Options > Environment > Preview Features and checkout the pull request branch to get started.
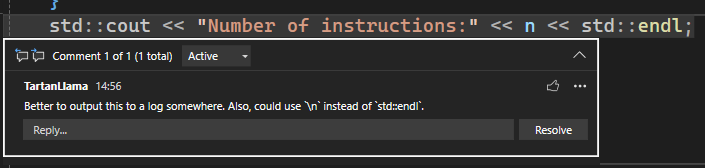
Generated Pull Request Descriptions
Similar to our generated Git commit message feature , you can now get a first draft for your pull request description created by GitHub Copilot. You’ll need to verify you have an active GitHub Copilot subscription. Try it out by clicking the ‘Add AI Generated Pull Request Description’ sparkle pen icon within the Create a Pull Request window. Please share your feedback on this feature here .
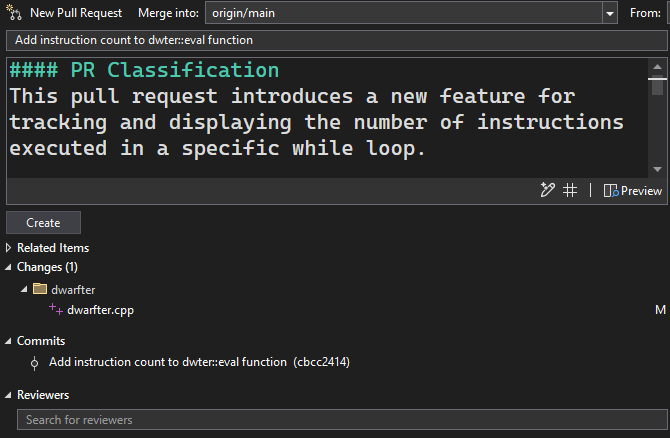
Image Hover Preview
If you hover over the path to an image, Visual Studio will now give you a small preview of the image itself, along with the size of the image in pixels and bytes. The size is capped to 500px wide and high.
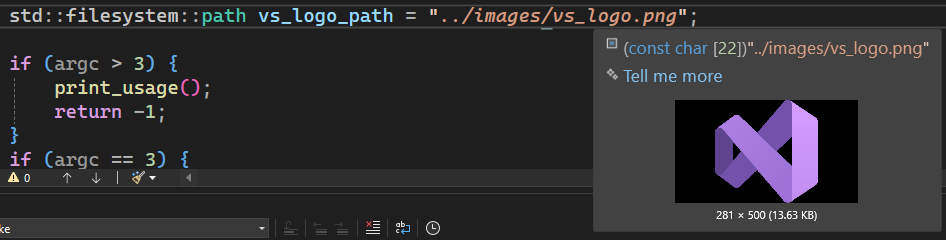
New Text Formatting Options
You can now choose italic, bold, strikethrough, or underline styles for text formatting in the Options > Environment > Fonts and Colors settings:
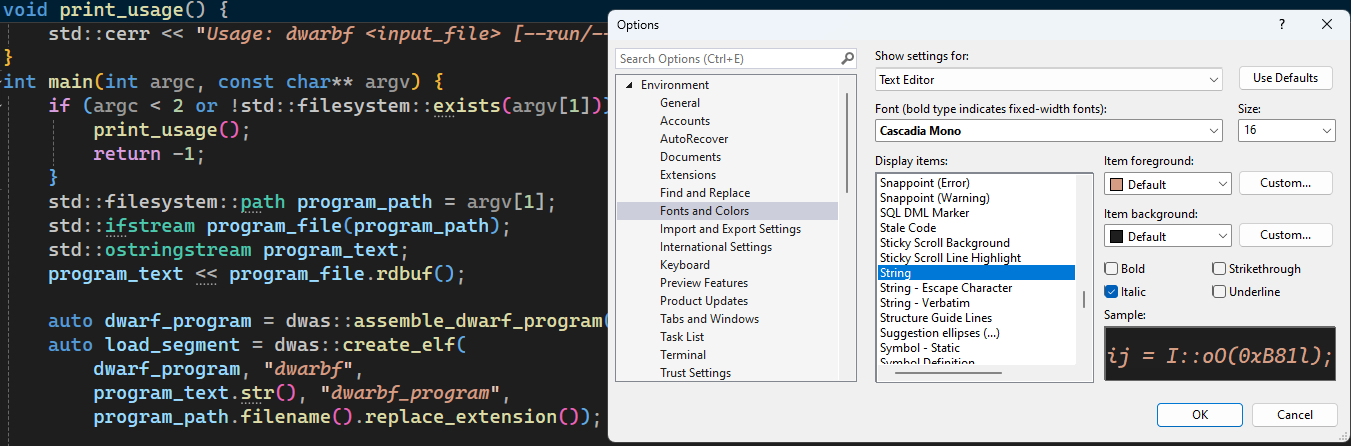
Creating Conditional Breakpoints and Tracepoints from Expressions
You can now create a conditional breakpoint or tracepoint directly from an expression in your source code from the right-click menu. This works on property or field names and values from autos, locals, watch windows, or DataTips:
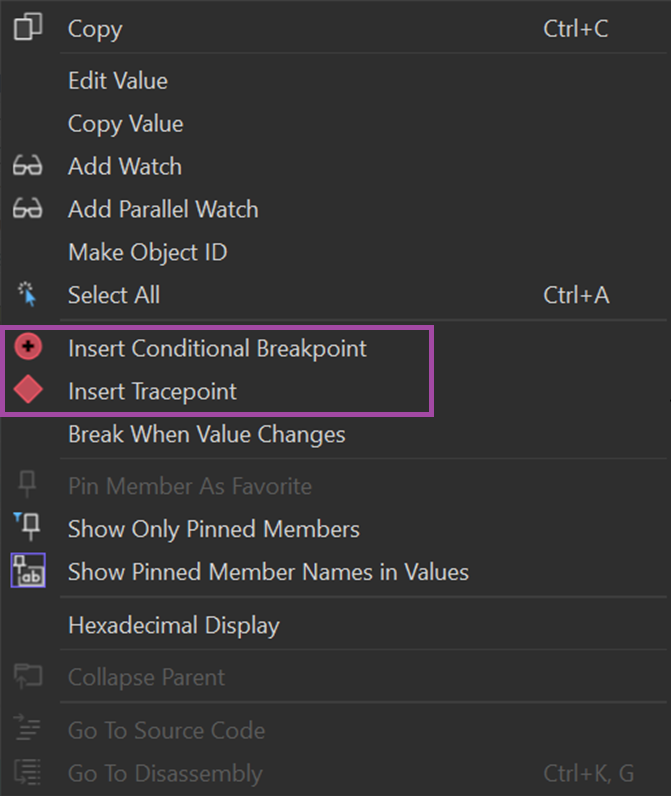
Attach to Process Dialog Revamp
The Attach to Process dialog has been improved for better functionality and user-friendliness. You can now easily switch between tree and list views, organize processes better with collapsible sections, and select code types with a simplified combobox. Moreover, the “Select/Track Window” feature is now easier to use, allowing two-way tracking: selecting a process highlights its window, and clicking on a window selects its process.

GitHub Copilot
We have unified the experience from the GitHub Copilot and Copilot Chat extensions and shipped them directly in Visual Studio. To install it, install the GitHub Copilot component in the Visual Studio Installer:
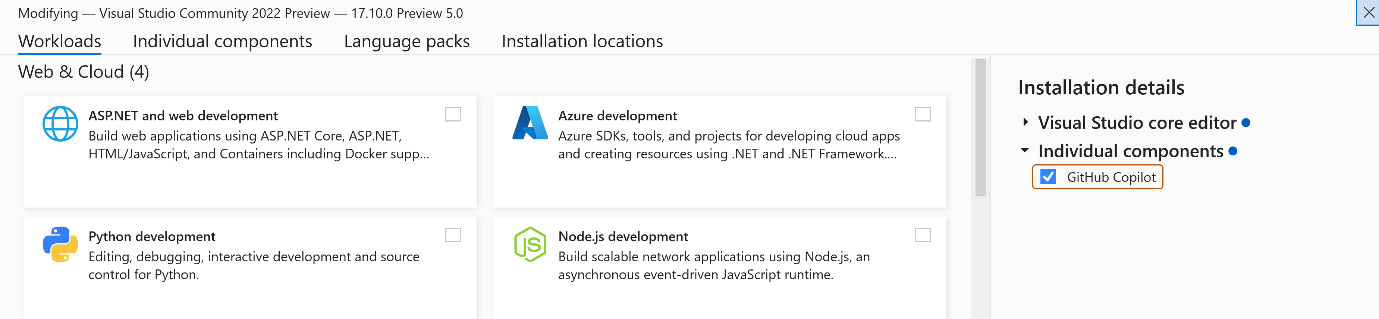
To use it, you’ll need an active GitHub Copilot subscription, and you can find the interface in the top-right corner of Visual Studio.
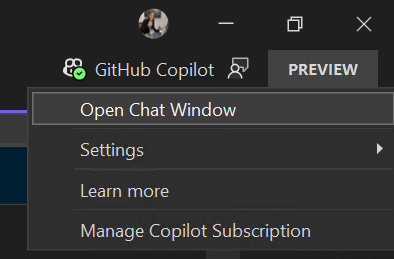
Send us your feedback
We are very much interested in your feedback to continue to improve this experience. The comments below are open. Feedback can also be shared through Visual Studio Developer Community . You can also reach us on Twitter ( @VisualC ), or via email at [email protected] .
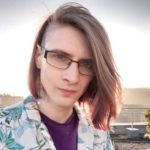
Sy Brand C++ Developer Advocate, C++ Team
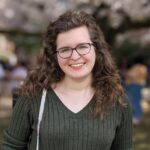
Leave a comment Cancel reply
Log in to join the discussion or edit/delete existing comments.
Very much appreciate the investments you’ve made in the native code tool chain in this release. I’d really like to see more of the same, particularly in the fields of: Code Analysis, scaling the solution for very large projects, local ML based navigational help for large code bases.
With the new version of the MSVC toolset, please consider documenting the behavior of the default value, and add a “latest” value to the corresponding project configuration: https://developercommunity.visualstudio.com/t/Default-value-of-MSVC-Toolset-is-not-doc/10398953

Insert/edit link
Enter the destination URL
Or link to existing content

IMAGES
VIDEO
COMMENTS
You have two choices for setting the default value of an instance variable (sometimes called an "instance field"): Using an initializer on the declaration. Using an assignment in a constructor. Say I have a class Example and an instance variable answer whose default should be 42. I can either:
1. If you need to set not null value or return default value instead you can use simple generic method. private <T> T getIfNotNullOrElse(T input, T defaultValue) {. return Optional.ofNullable(input).orElse(defaultValue);
2. Default Initialization of Arrays in Java. An array is an object, hence the default value of an uninitialized array (member variable) is null. For example: String [] names; // default is null. When the size of an array is initialized, then its components will have default values specified by the rules above.
In fact, the solution for default parameters using Parameter Object and Builder shines when the list of method parameters is much longer. Conclusion. Let's sum up. Java doesn't have a simple solution for default method parameters as available in other common programming languages. Yet, you can simulate it using other Java constructions and ...
The other optional parameters are milk (in ml), herbs (to add or not to add), and sugar (in tbsp). If any of their values are not provided, we assume the user doesn't want them. Let's see how to achieve this in Java using method overloading: this .name = name; this .milk = milk; this .herbs = herbs;
In Java, an initializer is a block of code that has no associated name or data type and is placed outside of any method, constructor, or another block of code. Java offers two types of initializers, static and instance initializers. Let's see how we can use each of them. 7.1. Instance Initializers.
Default parameters were introduced in Java 8 and are a part of the Java language specification. They work by allowing a developer to define a default value for a parameter when it is not explicitly passed in during a method call. To define a default parameter, simply assign a value to the parameter when it is declared in the method signature.
To overcome this issue, Java 8 has introduced the concept of default methods which allow the interfaces to have methods with implementation without affecting the classes that implement the interface. Output: 16. Default Method Executed. The default methods were introduced to provide backward compatibility so that existing interfaces can use the ...
A primitive type is predefined by the language and is named by a reserved keyword. Primitive values do not share state with other primitive values. The eight primitive data types supported by the Java programming language are: byte: The byte data type is an 8-bit signed two's complement integer. It has a minimum value of -128 and a maximum ...
variable operator value; Types of Assignment Operators in Java. The Assignment Operator is generally of two types. They are: 1. Simple Assignment Operator: The Simple Assignment Operator is used with the "=" sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
In Java, when a variable is declared but not initialized, it is assigned a default value based on its data type. The default values for the primitive data types in Java are as follows: byte: 0. short: 0. int: 0. long: 0L. float: 0.0f. double: 0.0d. char: '\u0000' (null character)
A lot of people mix up the default constructor for the no-argument constructor, but they are not the same in Java. Any constructor created by the programmer is not considered a default constructor in Java. Conclusion. In this article, we learned what constructors are and how we can create and use them to initialize our objects.
Creating the newListWithDefault () Method. If we need to initialize a mutable list with default values, we can create a list object and then add default values. Of course, we can create a static method to do this job: static <T> List<T> newListWithDefault(T value, int size) {. List<T> list = new ArrayList <>(size);
Quite often such things do have a function, in fact in Java in some situations it's required to do such an assignment. Even if not explicitly required, for clarity it's often a good idea to do such an assignment, even if only for readability.
At a high level, the JVM performs the following steps: First, the class is loaded and linked. Then, the "initialize" phase of this process processes the static variable initialization. Finally, the main method associated with the class is called. In the next section, we'll look at class variable initialization. 3.
PARTITION_ASSIGNMENT_STRATEGY_CONFIG, ... The RangeAssignor is the default strategy. The aims of this strategy is to co-localized partitions of several topics. ... let's create a new Java ...
Pattern matching offers an elegant way to deal with these challenges, improve code quality and reduce errors. Fundamentally, it lets us define patterns that describe data structures and types. We can then match values against these patterns; if a match is found, Java automatically extracts and assigns values as needed.
Assign a Default Value: If a variable might be null in certain situations, consider assigning a default value during initialization. This ensures it always points to something, even if not the intended object. ... (Java 8+): Lambdas and method references provide concise ways to perform null checks within higher-order functions (functions that ...
AssignCode.com. AssignCode is one of the best Java assignment help services that you can entrust with programming, mathematics, biology, engineering, physics, and chemistry. A large professional ...
0. In the first case you are declaring "int a" as a local variable (as declared inside a method) and local varible do not get default value. But instance variable are given default value both for static and non-static. Default value for instance variable: int = 0. float,double = 0.0. reference variable = null.
Similarly, we can set a zero-length String as the default value: @Value("${some.key:})" private String stringWithBlankDefaultValue; 3. Primitives. To set a default value for primitive types such as boolean and int, we use the literal value: @Value("${some.key:true}") private boolean booleanWithDefaultValue;
On the performance side, we improved the vectorized implementations of std::min_element, std::ranges::min and friends, made the copy/move assignment operators of std::expected trivial when expected, and more. C++ Productivity and Game Development. You can now use Build Insights to view your template instantiation information.
User configured concurrency gives customers flexibility in deciding based on the language and workload what concurrency they want to set per instance. So, for example, for Python customers they don't have to think about multithreading and can set concurrency =1 (which is also the default for Python apps).
In Java, you can use the @JsonInclude annotation provided by the Jackson library to assign a default value to a field when the field provided in JSON is null or empty. ... // Assign the default value here } // Getters and setters for yourField public String getYourField() { return yourField; } public void setYourField(String yourField) { this ...
The Java enum type provides a language-supported way to create and use constant values. By defining a finite set of values, the enum is more type safe than constant literal variables like String or int.. However, enum values are required to be valid identifiers, and we're encouraged to use SCREAMING_SNAKE_CASE by convention. Given those limitations, the enum value alone is not suitable for ...