Instantly share code, notes, and snippets.

DouglasRubin / Week 3 programming assignment_b.r
- Download ZIP
- Star ( 1 ) 1 You must be signed in to star a gist
- Fork ( 100 ) 100 You must be signed in to fork a gist
- Embed Embed this gist in your website.
- Share Copy sharable link for this gist.
- Clone via HTTPS Clone using the web URL.
- Learn more about clone URLs
- Save DouglasRubin/18d43a293a136ef8b15dbdf19ede386d to your computer and use it in GitHub Desktop.
setwd('C:/Users/rubind1/Documents/Coursera-R') | |
## | |
## I simply set the input x as a matrix | |
## and then set the solved value "s" as a null | |
## then I changed every reference to "mean" to "solve" | |
makeCacheMatrix <- function(x = matrix(sample(1:100,9),3,3)) { | |
s <- NULL | |
set <- function(y) { | |
x <<- y | |
s <<- NULL | |
} | |
get <- function() x | |
setsolve <- function(solve) s <<- solve | |
getsolve <- function() s | |
list(set = set, get = get, | |
setsolve = setsolve, | |
getsolve = getsolve) | |
} | |
## | |
## Same here, changed "mean" to "solve" and "m" to "s" | |
cacheSolve <- function(x, ...) { | |
s <- x$getsolve() | |
if(!is.null(s)) { | |
message("getting inversed matrix") | |
return(s) | |
} | |
data <- x$get() | |
s <- solve(data, ...) | |
x$setsolve(s) | |
s | |
} |
nithyabk commented May 7, 2020
Put comments here that give an overall description of what your, functions do, our aim in this experiment is to write a pair of functions, namely,, "makecachematrix" and "cachesolve" that cache the inverse of a matrix, write a short comment describing this function, makecachematrix is a function which creates a special "matrix" object that can, cache its inverse for the input (which is an invertible square matrix).
makeCacheMatrix <- function(x = matrix()) {
inv <- NULL set <- function(y) { x <<- y inv <<- NULL } get <- function() x setinv <- function(inverse) inv <<- inverse getinv <- function() inv list(set = set, get = get, setinv = setinv, getinv = getinv) }
cacheSolve is a function which computes the inverse of the special "matrix"
Returned by makecachematrix above. if the inverse has already been calculated, (and the matrix has not changed), then the cachesolve should retrieve the, inverse from the cache.
cacheSolve <- function(x, ...) { ## Return a matrix that is the inverse of 'x' inv <- x$getinv() if(!is.null(inv)) { message("getting cached result") return(inv) } data <- x$get() inv <- solve(data, ...) x$setinv(inv) inv }
---------------Checking the program------------------------
M <- matrix(rnorm(16),4,4), m1 <- makecachematrix(m), cachesolve(m1), [,1] [,2] [,3] [,4], [1,] -0.1653269 0.2592203 0.6176218 -0.7520955, [2,] 0.2828334 -0.1853499 0.4511382 0.2094365, [3,] 0.1434840 1.0413868 -0.3550853 -0.3261154, [4,] 0.1793583 -0.4252171 -0.4371493 -0.1749830.
Sorry, something went wrong.
nithyabk commented May 9, 2020
Caching the inverse of a matrix:, matrix inversion is usually a costly computation and there may be some, benefit to caching the inverse of a matrix rather than compute it repeatedly., below are a pair of functions that are used to create a special object that, stores a matrix and caches its inverse., this function creates a special "matrix" object that can cache its inverse..
makeCacheMatrix <- function(x = matrix()) { inv <- NULL set <- function(y) { x <<- y inv <<- NULL } get <- function() x setInverse <- function(inverse) inv <<- inverse getInverse <- function() inv list(set = set, get = get, setInverse = setInverse, getInverse = getInverse) }
This function computes the inverse of the special "matrix" created by
Makecachematrix above. if the inverse has already been calculated (and the, matrix has not changed), then it should retrieve the inverse from the cache..
cacheSolve <- function(x, ...) { ## Return a matrix that is the inverse of 'x' inv <- x$getInverse() if (!is.null(inv)) { message("getting cached data") return(inv) } mat <- x$get() inv <- solve(mat, ...) x$setInverse(inv) inv }
testing my function
source("ProgrammingAssignment2/cachematrix.R") my_matrix <- makeCacheMatrix(matrix(1:4, 2, 2)) my_matrix$get() [,1] [,2] [1,] 1 3 [2,] 2 4 my_matrix$getInverse() NULL cacheSolve(my_matrix) [,1] [,2] [1,] -2 1.5 [2,] 1 -0.5 cacheSolve(my_matrix) getting cached data [,1] [,2] [1,] -2 1.5 [2,] 1 -0.5 my_matrix$getInverse() [,1] [,2] [1,] -2 1.5 [2,] 1 -0.5 my_matrix$set(matrix(c(2, 2, 1, 4), 2, 2)) my_matrix$get() [,1] [,2] [1,] 2 1 [2,] 2 4 my_matrix$getInverse() NULL cacheSolve(my_matrix) [,1] [,2] [1,] 0.6666667 -0.1666667 [2,] -0.3333333 0.3333333 cacheSolve(my_matrix) getting cached data [,1] [,2] [1,] 0.6666667 -0.1666667 [2,] -0.3333333 0.3333333 my_matrix$getInverse() [,1] [,2] [1,] 0.6666667 -0.1666667 [2,] -0.3333333 0.3333333
gaganrvn commented May 26, 2020
This is really well done! You do an excellent job coding, but one opinion is to stick to getInverse and setInverse so as to make your code easier to read. Your code is very well done, and a small bit of indenting would make it perfect. Well Done!
nithyabk commented May 26, 2020
Thank you so much sir.
Vemiya commented May 28, 2020
NoeVelarde commented Aug 13, 2020
okamahen commented Nov 12, 2020
Good documentation, but indentation will clearly make this better :)
varelaz commented Feb 15, 2021
You didn't make a fork as was requested, but code is correct
VerdySeptian commented May 10, 2021
sherlu commented Aug 14, 2021
works well. Just the name of setsolve, getsolve etc are confusing because the work is to set/get inverse :)
Seirakos commented Sep 16, 2021
good work :3
Piku1997 commented Oct 31, 2021
Dijusharon commented Feb 21, 2022
how to submit in github

CaringPerson commented Jul 18, 2022
nice work and documentation
abdullah30 commented Feb 14, 2023
I did not get the point that how we can submit the assignment. Can you help me in getting it? Regards Abdullah
Saksham2805 commented Apr 23, 2023
phume2010 commented Apr 23, 2023
Your code is easy to follow
ZilolaEE commented Aug 22, 2023
markarecio commented Jan 18, 2024
Data Visualization Week 3 Programming Assignment 2
An information flow model for conflict and fission in small group.
This file implements Programming Assignment 2: Visualize Network Data for the Coursera Data Visualization Class.
This assignment uses R Programming Language to visualize data.
Q1. What is the data that you chose? Why?
Data from a voluntary association are used to construct a new formal model for a traditional anthropological problem, fission in small groups. The process leading to fission is viewed as an unequal flow of sentiments and information across the ties in a social network. This flow is unequal because it is uniquely constrained by the contextual range and sensitivity of each relationship in the network. The subsequent differential sharing of sentiments leads to the formation of subgroups with more internal stability than the group as a whole and results in fission.
Q2. Did you use a subset of the data? If so, what was it?
Ans: No I used the full data set as available from this link.
Q3. Are there any particular aspects of your visualization to which you would like to bring attention?
To improve the visualization and make it more clear:
- Colors (Hue,): I’ve used colors to indicate which conferences the nodes belong to. The colors corespondent to the colors as seen in the legend on the left.
- Clustering: All nodes are clustered.
The chart is made interactive:
- Hovering a node will highlight and color the links to it’s linked nodes.
- Hovering a node will temporary enlarge a node.
Q4. What do you think the data and your visualization show?
An edge is drawn if two individuals consistently were observed to interact outside the normal activities of the club (karate classes and club meetings). That is, an edge is drawn if the individuals could be said to be friends outside the club activities. All the edges in Figure 1 are non-directional (they represent interaction in both directions), and the graph is said to be symmetrical.
Loading the Data
The Data is obtained from University of Michigan Network Data: Zachary’s karate club social network of friendships between 34 members of a karate club at a US university in the 1970s. Please cite W. W. Zachary, An information flow model for conflict and fission in small groups, Journal of Anthropological Research 33, 452-473 (1977).
Loading the packages which will be used in analyzing the data
Convert the igraph data into something more suitable for networkD3
networkD3 requires edge references to nodes start from 0.
Create the interactive D3 plot.
Figure 1: This is the graphic representation of the social relationships among the 34 individuals in the karate club. A line is drawn between two points when the two individuals being represented consistently interacted in contexts outside those of karate classes, workouts, and club meetings. Each such line drawn is referred to as an edge.
Note: You can Zoom with the scroll wheel.
- Coursera 'Data Visualization in R with ggplot2' course by Johns Hopkins University: Week 3 Peer Review
- by Data Analyst / Quantitative Analysis
- Last updated over 2 years ago
- Hide Comments (–) Share Hide Toolbars
Twitter Facebook Google+
Or copy & paste this link into an email or IM:
Get the Reddit app
Coursera is a global online learning platform that offers anyone, anywhere, access to online courses and degrees from leading universities and companies
Peer assignments not getting graded
Hi. I'm currently doing a course on responsive website development. While in the first 2 parts everything was going smoothly, now in the 3rd part my peer reviewed assignments aren't getting graded.
I've submitted 4 assignments way before the "deadline", it's been at least a week and not even the first assignment has been graded, even though I asked for reviews in the discussion section. I've already reviewed others work. The date in the "your assignment should be graded by" part also changes and gets pushed back each day.
How long does it take to get graded? What happens if I've finished everything else but can't get thw certificate cause the assignments aren't getting graded? Do I contact coursera? I really don't wanna waste money here.
- Find a Course
- For Business
- For Educators
- Product News
Coursera launches a new suite of Academic Integrity features to help universities verify learning in an age of AI-assisted cheating
June 11, 2024
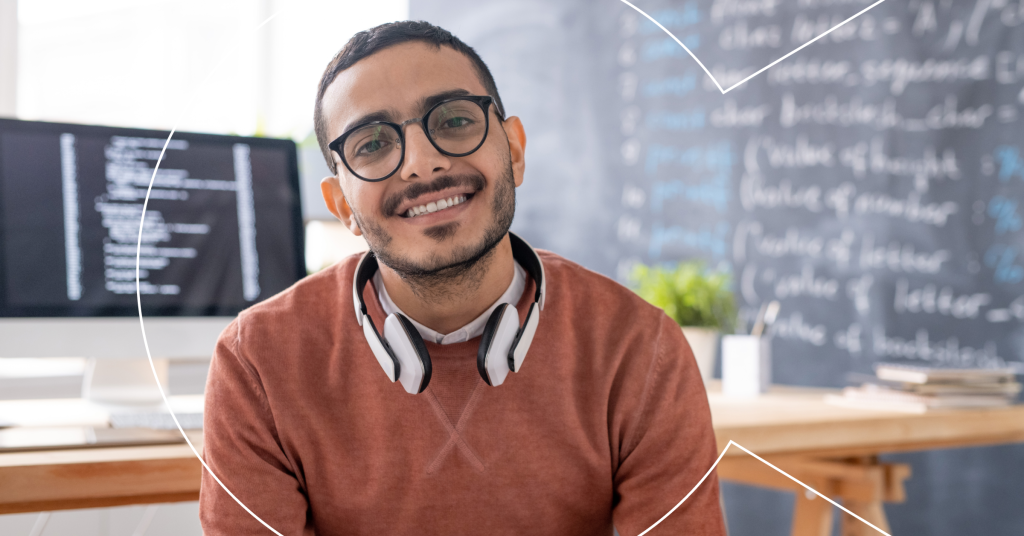
By Jeff Maggioncalda, CEO Coursera
Today, we’re excited to announce several new genAI-powered features designed to scale assessment creation and grading, strengthen academic integrity, and enhance learning and evaluation. These features, including AI-Assisted Grading, Proctoring and Lockdown Browser, and AI-based Viva Exams will help campuses deliver authentic learning to students while increasing the value of online assessments, courses, and certificates.
Online learning has become a powerful tool for institutions to better prepare students for a rapidly changing world. However, universities must ensure it meets the rigorous standards required for academic credit. While Generative AI introduces new risks for student misconduct, it also provides unprecedented opportunities for universities to enhance academic integrity at scale.
The features we launch today illustrate how new technologies can support a more authentic, verified learning experience for students, educators, and employers.
Scale Assessment Creation and Grading:
- AI Assessment Generator – Saves educators’ time by generating diverse math, text, and multiple-choice assessments tailored to courses and seamlessly integrating them into assignments.
- Question Banks and Variants – Provides a variety of questions and multiple variants for robust testing, making exams difficult to predict and easier to author.
- *AI-Assisted Grading – Streamlines grading by suggesting scores and feedback based on assignment analysis, with final decisions remaining with graders.
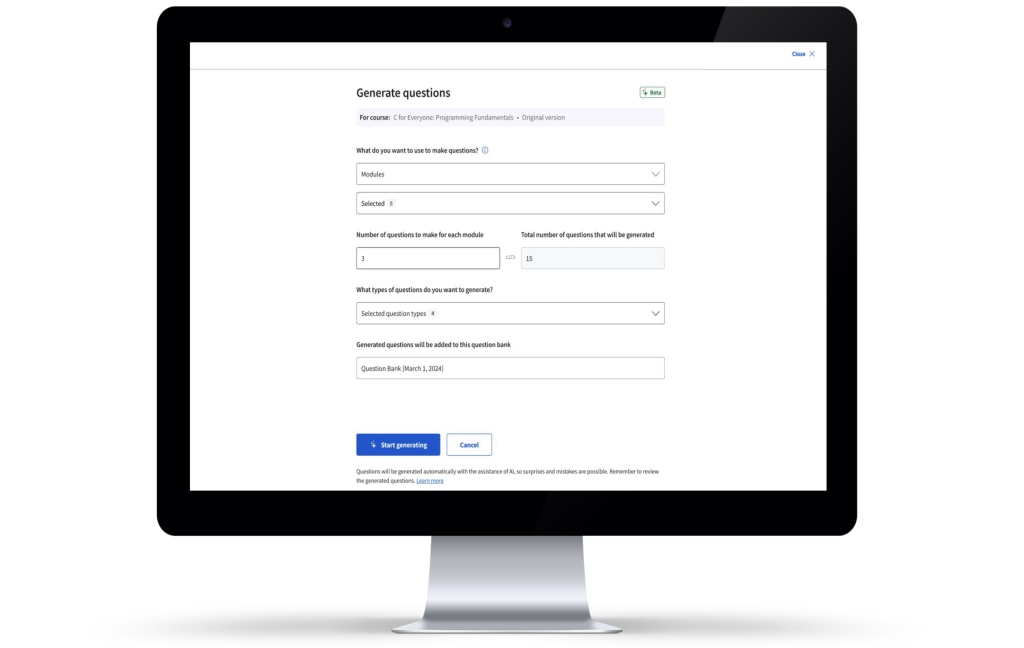
AI Assessment Generator Strengthen Academic Integrity:
- Graded Item Locking – Requires students to complete each lesson item, including readings, videos, and labs, to unlock graded items, promoting comprehensive learning and preventing skipping ahead.
- Time and Attempt Limits – Controls exam attempts to ensure fairness, discourage trial and error, and limit outside resource access, encouraging meaningful engagement and reflection.
- Proctoring and Lockdown Browser – Maintains exam integrity by blocking unauthorized resources and detecting unauthorized assistance during high-stakes exams.
- Plagiarism Detection – Instantly identifies content similar to previous submissions, deterring plagiarism and educating students on independent thinking and originality.
Enhance Learning and Evaluation:
- *Quiz Prep and Prior Learning Recaps with Coursera Coach – Provides a personalized tutor – powered by GenAI and grounded in our expert content – that provides interactive learning assistance, lecture summaries, guided practice with spaced repetition, and tailored pre-assessment reviews.
- AI Peer Reviews – Facilitates peer feedback with AI-powered insights, evaluating text-based submissions and generating grades using assignment rubrics. In a global pilot, 97% of surveyed Coursera Plus learners preferred AI grading over peer grading, citing better feedback quality, increased rigor, and reduced wait times.
- *AI-based Viva Exam – Analyzes free-form written submissions and grading criteria to dynamically generate custom follow-up questions that test the authenticity and critical thinking behind the student’s submission. Enables educators to conduct viva-style written exams, where students ‘show their work’ and graders receive detailed analysis.
AI-based Viva Exam Today, we also announced the launch of a new course from Vanderbilt University – Generative AI for University Leaders , aimed at better preparing academic institutions for the rapid change brought on by GenAI. The free course is taught by Professor Jules White, Senior Advisor to the Chancellor on Generative AI, and features guest experts including Andrew Ng of DeepLearning.AI, Mayank Shrivastava of SAP, and myself. The course covers everything from how GenAI works and why it matters to how university leaders can create a GenAI strategy and ensure its appropriate usage on campuses.
Together, the course and new features will help universities address the challenges posed by GenAI and uphold a culture of academic excellence. This launch builds on our recent recognition as the first online learning platform to receive ACE’s Authorized Instructional Platform designation , helping ensure student outcomes genuinely reflect their effort, mastery of course material, and skills. These are major steps towards boosting the reputation and value of online learning and industry micro-credentials, making it easier for universities and employers to recognize them for academic credit and as qualifications for employment.
To learn more about Coursera for Campus academic integrity features, visit coursera.org/campus/academic-integrity .
*These features are currently in pilot with select customers. They will become available to all campus customers after the pilot phase is complete.
Keep reading
- Presenting the 2024 Coursera Global Skills Report
- Announcing four new entry-level certificates and Universal Skills scholarship program from Microsoft to help learners land in-demand jobs
- Clemson University partners with Coursera to launch first degree in South Carolina with no application*
- Google launches AI Essentials course on Coursera to help learners boost their productivity and thrive
- Twelve Google and IBM Professional Certificates on Coursera receive ECTS credit recommendations
- Coursera Launches Generative AI Academy to Improve Executive and Foundational Literacy
- Introducing “Navigating Generative AI: A CEO Playbook”
- Nevada DETR and Coursera announce statewide program providing free job training to thousands of people
- University of Texas System and Coursera Launch the Most Comprehensive Industry Micro-Credential Program Offered by a U.S. University System
- Coursera announces new AI content and innovations to help HR and learning leaders drive organizational agility amid relentless disruption
- New Coursera survey shows high demand for industry micro-credentials from students and employers in tight labor market
- Coursera and Google partner with the University of Texas System to provide critical job skills to students across eight campuses
- What the world learned on Coursera in 2022
- Coursera partners with state university and workforce systems to prepare Louisiana’s workforce for jobs of the future
- Connecting Google certificates and university Specializations to help learners prepare for in-demand career fields
- Coursera partners with IFC and the European Commission to publish global study on women and online learning in emerging markets
- Coursera Launches Clips to Accelerate Skills Development through Short Videos and Lessons
- Preparing learners around the world for in-demand jobs with Career Academy and new entry-level certificates from Meta and IBM
- Coursera and Milken Center for Advancing the American Dream launch free nationwide skills training initiative for underserved Americans
- Coursera’s response to the humanitarian crisis in Ukraine
- Coursera announces 3 new job-relevant degrees from leading universities
- Coursera doubles down on Middle East with new leadership, content and platform features
- Coursera Women and Skills Report indicates a narrowing gender gap in online learning
- Coursera accelerates India growth plans
- Announcing the Coursera Global Skills Report 2021
- Launching “Generative AI for University Leaders”
- Coursera Coach: Leveraging GenAI to Empower Learners

IMAGES
VIDEO
COMMENTS
R-Programming-Assignment-Week-3. Introduction. This second programming assignment will require you to write an R function is able to cache potentially time-consuming computations. For example, taking the mean of a numeric vector is typically a fast operation. However, for a very long vector, it may take too long to compute the mean, especially ...
Original file line number Diff line number Diff line change @@ -1,15 +1,36 @@ ## Put comments here that give an overall description of what your ## functions do ## These functions written in partial fulfillment of Coursera Data Science: R Programming ## Week 3 Assignment; week beginning January 18, 2016; GitHub user: PamlaM ## Write a short comment describing this function
Week 3 programming assignment_b.r This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
This video contains the code for programming assignment-3 and the step by step instructions for submission of assignment which includes forking, cloning repo...
This second programming assignment will require you to write an R\nfunction that is able to cache potentially time-consuming computations.\nFor example, taking the mean of a numeric vector is typically a fast\noperation.
The following function calculates the mean of the special "vector" created with the above function. However, it first checks to see if the mean has already been calculated. If so, it gets the mean from the cache and skips the computation. Otherwise, it calculates the mean of the data and sets the value of the mean in the cache via the ...
Sign inRegister. R Programming Week 3 Programming Assignments 2: Lexical Scoping. by Louis Stanley. Last updatedover 1 year ago. HideComments(-)ShareHide Toolbars. ×. Post on: TwitterFacebookGoogle+. Or copy & paste this link into an email or IM:
To submit a peer-graded assignment: Navigate to the week or module that the peer-graded assignment is in, then open it. Review the Instructions for the assignment. The course instructor typically provides requirements, submission instructions, and tips. Complete the assignment by responding to each prompt.
This assignment uses R Programming Language to visualize data. Q1. What is the data that you chose? Why? Data from a voluntary association are used to construct a new formal model for a traditional anthropological problem, fission in small groups. The process leading to fission is viewed as an unequal flow of sentiments and information across ...
This week covers the basics to get you started up with R. The Background Materials lesson contains information about course mechanics and some videos on installing R. The Week 1 videos cover the history of R and S, go over the basic data types in R, and describe the functions for reading and writing data.
This specialization continues and develops on the material from the Data Science: Foundations using R specialization. It covers statistical inference, regression models, machine learning, and the development of data products. In the Capstone Project, you'll apply the skills learned by building a data product using real-world data.
Welcome to Introduction to R Programming for Data Science • 3 minutes • Preview module. Introduction to R Language • 2 minutes. Basic Data Types • 5 minutes. Math, Variables, and Strings • 4 minutes. R Environment • 4 minutes. Introduction to RStudio • 3 minutes. Writing and Running R in Jupyter Notebooks • 4 minutes. 1 reading ...
Try re-submitting your assignment so other learners can review and grade it. When you re-submit a peer-graded assignment, peer reviews and grades for your first submission will be deleted. In most courses, there's no penalty for resubmitting (except for some Degree and MasterTrack courses). If you received a 0 due to an honor code violation:
Click the My Learning tab. Find the course in the list, then open it. In the course sidebar, click Grades. Click the name of the assignment. Click My Submission. If you have grades or feedback, you'll see them at the bottom of each part of your assignment. Note: If you don't see grades and feedback, visit Solve problems with peer-graded ...
R Pubs by RStudio. Sign in Register R Programming - Week 3 Assignment; by Ken Wood; Last updated over 3 years ago; Hide Comments (-) Share Hide Toolbars
in this tutorial you have will learn way of grading your peer graded assignment
Coursera 'Data Visualization in R with ggplot2' course by Johns Hopkins University: Week 3 Peer Review; by Data Analyst / Quantitative Analysis
There are 4 modules in this course. In this course, you will learn the Grammar of Graphics, a system for describing and building graphs, and how the ggplot2 data visualization package for R applies this concept to basic bar charts, histograms, pie charts, scatter plots, line plots, and box plots. You will also learn how to further customize ...
I'm currently doing a course on responsive website development. While in the first 2 parts everything was going smoothly, now in the 3rd part my peer reviewed assignments aren't getting graded. I've submitted 4 assignments way before the "deadline", it's been at least a week and not even the first assignment has been graded, even though I asked ...
Commit your completed R file into YOUR git repository and push your git branch to the GitHub repository under your account. Submit to Coursera the URL to your GitHub repository that contains the completed R code for the assignment. Grading. This assignment will be graded via peer assessment.
Contribute to PaareshC/C-for-Everyone-Programming-Fundamentals-by-University-of-California-Santa-Cruz development by creating an account on GitHub. ... Peer graded Assignment Fix Dr P's mistake (week 3).txt ... Peer graded Assignment Fix Dr P's mistake (week 3).txt. Top. File metadata and controls. Code. Blame. 16 lines (14 loc) · 259 Bytes ...
AI Peer Reviews - Facilitates peer feedback with AI-powered insights, evaluating text-based submissions and generating grades using assignment rubrics. In a global pilot, 97% of surveyed Coursera Plus learners preferred AI grading over peer grading, citing better feedback quality, increased rigor, and reduced wait times.
RMarkdown Lab • 60 minutes. 6 plugins • Total 171 minutes. R for Data Science - Introduction • 1 minute. RStudio - What and Why • 15 minutes. Hands-On Programming with R - Chapter 2 • 60 minutes. R for Data Science - Chapter 4 • 30 minutes. Reproducible Research • 20 minutes. R for Data Science - RMarkdown • 45 minutes.
This file contains the final project on the Introduction to R Programming for Data Science course by IBM Skills Network and available on Coursera. This project does not include data visualiazation exercises and ittle to no emphasis is placed on data wrangling. Rather, it focuses on the basic steps in R programming as relating to Data Science.
The program is for students 5th to 12th grade. This permits at-home use of a device for completing coursework and working with peers. We also provide ipads as language support for newcomers. Common sense media: Common sense media is a free security and digital citizenship curriculum. Teachers provide lessons from the program 3 times a year.
Find helpful learner reviews, feedback, and ratings for Project Initiation: Starting a Successful Project from Google. Read stories and highlights from Coursera learners who completed Project Initiation: Starting a Successful Project and wanted to share their experience. everything is excellent. but i am facing the problem that says 'upgrade to submit' when i traied to ...
Programming on the Coursera Platform ... Informative but the last assignment on Week 4 is misleading in that you can only submit the search function but the instructions say that you can test your code and the program will test it as well. R. RK. 5. Reviewed on Nov 1, 2019. Course content is good, graded assignments are good, I just had ...