Java Tutorial
Java methods, java classes, java file handling, java how to's, java reference, java examples, java arrays.
Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value.
To declare an array, define the variable type with square brackets :
We have now declared a variable that holds an array of strings. To insert values to it, you can place the values in a comma-separated list, inside curly braces:
To create an array of integers, you could write:

Access the Elements of an Array
You can access an array element by referring to the index number.
This statement accesses the value of the first element in cars:
Try it Yourself »
Note: Array indexes start with 0: [0] is the first element. [1] is the second element, etc.
Change an Array Element
To change the value of a specific element, refer to the index number:
Array Length
To find out how many elements an array has, use the length property:
Test Yourself With Exercises
Create an array of type String called cars .
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
How to Declare and Initialize an Array in Java
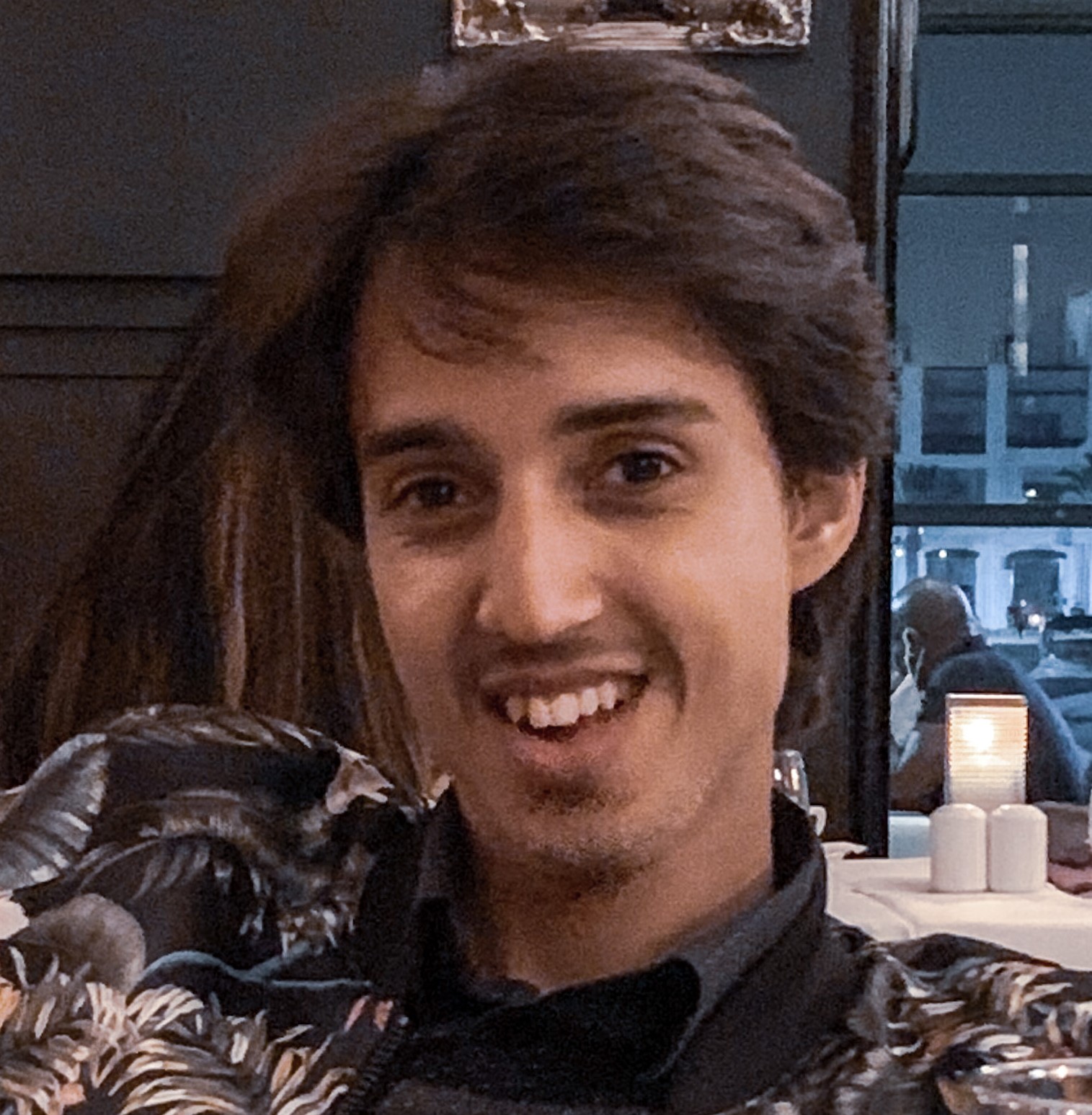
- Introduction
In this tutorial, we'll take a look at how to declare and initialize arrays in Java .
We declare an array in Java as we do other variables, by providing a type and name:
To initialize or instantiate an array as we declare it, meaning we assign values as when we create the array, we can use the following shorthand syntax:
Or, you could generate a stream of values and assign it back to the array:
To understand how this works, read more to learn the ins and outs of array declaration and instantiation!
- Array Declaration in Java
- Array Initialization in Java
- IntStream.range()
- IntStream.rangeClosed()
- IntStream.of()
- Java Array Loop Initialization
The declaration of an array object in Java follows the same logic as declaring a Java variable. We identify the data type of the array elements, and the name of the variable, while adding rectangular brackets [] to denote its an array.
Here are two valid ways to declare an array:
The second option is oftentimes preferred, as it more clearly denotes of which type intArray is.
Note that we've only created an array reference. No memory has been allocated to the array as the size is unknown, and we can't do much with it.
To use the array, we can initialize it with the new keyword, followed by the data type of our array, and rectangular brackets containing its size:
This allocates the memory for an array of size 10 . This size is immutable.
Java populates our array with default values depending on the element type - 0 for integers, false for booleans, null for objects, etc. Let's see more of how we can instantiate an array with values we want.
The slow way to initialize your array with non-default values is to assign values one by one:
In this case, you declared an integer array object containing 10 elements, so you can initialize each element using its index value.
The most common and convenient strategy is to declare and initialize the array simultaneously with curly brackets {} containing the elements of our array.
The following code initializes an integer array with three elements - 13, 14, and 15:
Keep in mind that the size of your array object will be the number of elements you specify inside the curly brackets. Therefore, that array object is of size three.
This method work for objects as well. If we wanted to initialize an array of three Strings, we would do it like this:
Java allows us to initialize the array using the new keyword as well:
It works the same way.
Check out our hands-on, practical guide to learning Git, with best-practices, industry-accepted standards, and included cheat sheet. Stop Googling Git commands and actually learn it!
Note : If you're creating a method that returns an initialized array, you will have to use the new keyword with the curly braces. When returning an array in a method, curly braces alone won't work:
If you're declaring and initializing an array of integers, you may opt to use the IntStream Java interface:
The above code creates an array of ten integers, containing the numbers 1 to 10:
The IntStream interface has a range() method that takes the beginning and the end of our sequence as parameters. Keep in mind that the second parameter is not included, while the first is.
We then use the method toArray() method to convert it to an array.
Note: IntStream is just one of few classes that can be used to create ranges. You can also use a DoubleStream or LongStream in any of these examples instead.
If you'd like to override that characteristic, and include the last element as well, you can use IntStream.rangeClosed() instead:
This produces an array of ten integers, from 1 to 10:
The IntStream.of() method functions very similarly to declaring an array with some set number of values, such as:
Here, we specify the elements in the of() call:
This produces an array with the order of elements preserved:
Or, you could even call the sorted() method on this, to sort the array as it's being initialized:
Which results in an array with this order of elements:
One of the most powerful techniques that you can use to initialize your array involves using a for loop to initialize it with some values.
Let's use a loop to initialize an integer array with values 0 to 9:
This is identical to any of the following, shorter options:
A loop is more ideal than the other methods when you have more complex logic to determine the value of the array element.
For example, with a for loop we can do things like making elements at even indices twice as large:
In this article, we discovered the different ways and methods you can follow to declare and initialize an array in Java. We've used curly braces {} , the new keyword and for loops to initialize arrays in Java, so that you have many options for different situations!
We've also covered a few ways to use the IntStream class to populate arrays with ranges of elements.
You might also like...
- Java: Finding Duplicate Elements in a Stream
- Spring Boot with Redis: HashOperations CRUD Functionality
- Spring Cloud: Hystrix
- Java Regular Expressions - How to Validate Emails
Improve your dev skills!
Get tutorials, guides, and dev jobs in your inbox.
No spam ever. Unsubscribe at any time. Read our Privacy Policy.
I am a very curious individual. Learning is my drive in life and technology is the language I speak. I enjoy the beauty of computer science and the art of programming.
In this article
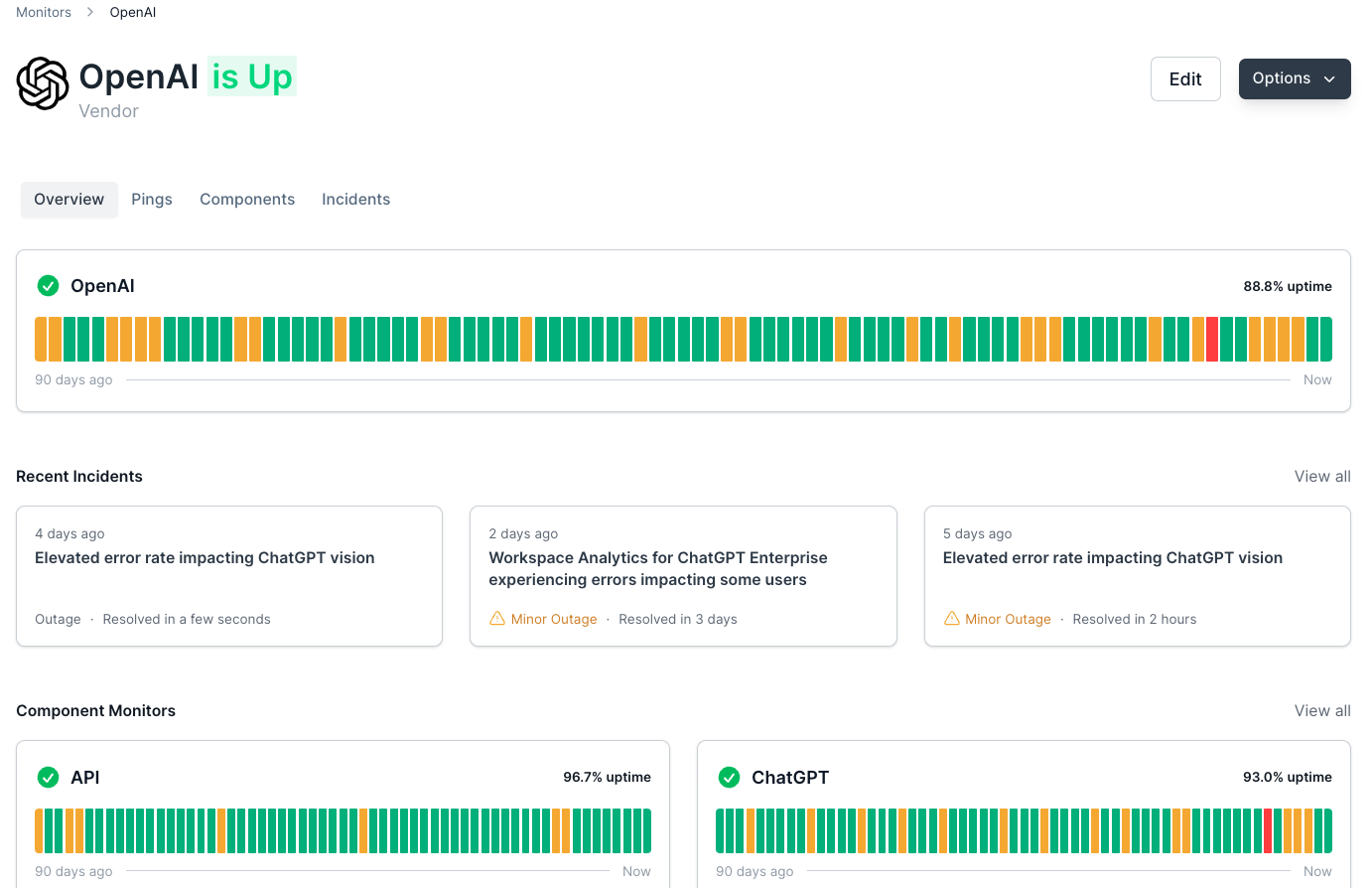
Monitor with Ping Bot
Reliable monitoring for your app, databases, infrastructure, and the vendors they rely on. Ping Bot is a powerful uptime and performance monitoring tool that helps notify you and resolve issues before they affect your customers.
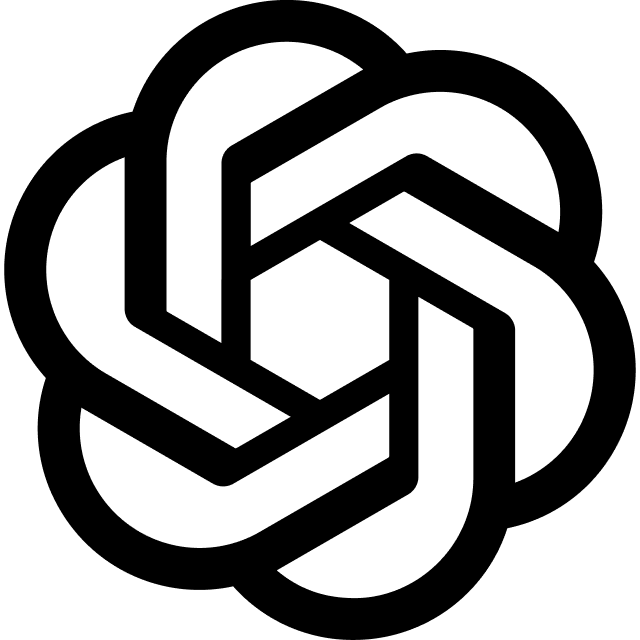
Vendor Alerts with Ping Bot
Get detailed incident alerts about the status of your favorite vendors. Don't learn about downtime from your customers, be the first to know with Ping Bot.
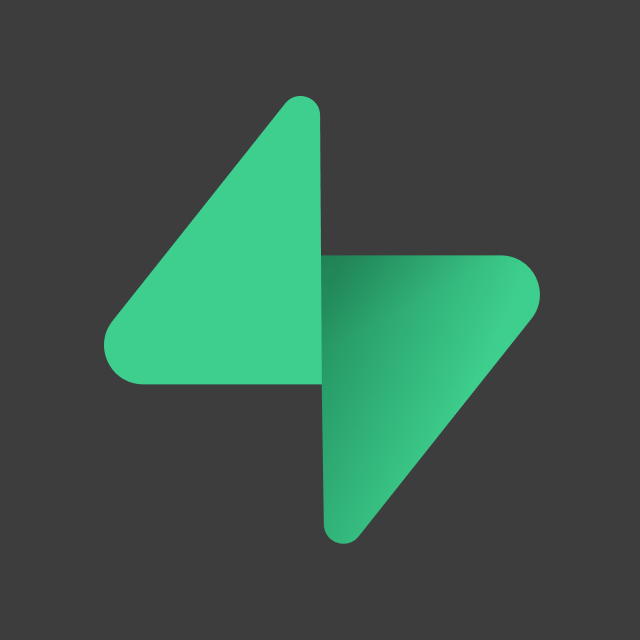
© 2013- 2024 Stack Abuse. All rights reserved.
In this article we cover arrays. An array is a container object that holds a fixed number of values of a single type. The length of an array is established when the array is created. After creation, its length is fixed.
Array definition
Arrays are used to store data of our applications. We declare arrays to be of a certain data type. We specify their length. And we initialize arrays with data. We have several methods for working with arrays. We can modify the elements, sort them, copy them or search for them.
Collections serve a similar purpose like arrays. They are more powerful than arrays. They will be described later in a separate chapter.
Initializing arrays
We create and initialize a numerical array. The contents of the array are printed to the console.
The Arrays class is a helper class which contains various methods for manipulating arrays. The toString method returns a string representation of the contents of the specified array. This method is helpful in debugging.
The one step creation and initialization can be further simplified by only specifying the numbers between the curly brackets.
An array of integers is created using the most simple way of array creation.
Accessing array elements
After the array is created, its elements can be accessed by their index. The index is a number placed inside square brackets which follow the array name.
In the example, we create an array of string names. We access each of the elements by its index and print them to the terminal.
Each of the elements of the array is printed to the console. With the names[0] construct, we refer to the first element of the names array.
We have an array of three integers. Each of the values will be multiplied by two.
Traversing arrays
An array of planet names is created. We use the for loop to print all the values.
Passing arrays to methods
In the next example, we pass an array to a method.
Inside the body of the method, a new array is created; it will contain the newly ordered elements.
Multidimensional arrays
So far we have been working with one-dimensional arrays. In Java, we can create multidimensional arrays. A multidimensional array is an array of arrays. In such an array, the elements are themselves arrays. In multidimensional arrays, we use two or more sets of brackets.
Two for loops are used to print all the six values from the two-dimensional array. The first index of the twodim[i][j] array refers to one of the inner arrays. The second index refers to the element of the chosen inner array.
Three-dimensional array n3 is created. It is an array that has elements which are themselves arrays of arrays.
Irregular arrays
This is a declaration and initialization of an irregular array. The three inner arrays have 2, 3, and 4 elements.
Array methods
In the code example, we present five methods of the Arrays class.
The copyOf method copies the specified number of elements to a new array.
Comparing arrays
The c array has two inner arrays. The elements of the inner arrays are equal to the a and b arrays.
Searching arrays
In the example, we search for the "Earth" string in an array of planets.
Download image
In the next example, we show how to download an image.
In this article we worked with arrays.
My name is Jan Bodnar and I am a passionate programmer with many years of programming experience. I have been writing programming articles since 2007. So far, I have written over 1400 articles and 8 e-books. I have over eight years of experience in teaching programming.
The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.
An array is a container object that holds a fixed number of values of a single type. The length of an array is established when the array is created. After creation, its length is fixed. You have seen an example of arrays already, in the main method of the "Hello World!" application. This section discusses arrays in greater detail.
An array of 10 elements.
Each item in an array is called an element , and each element is accessed by its numerical index . As shown in the preceding illustration, numbering begins with 0. The 9th element, for example, would therefore be accessed at index 8.
The following program, ArrayDemo , creates an array of integers, puts some values in the array, and prints each value to standard output.
The output from this program is:
In a real-world programming situation, you would probably use one of the supported looping constructs to iterate through each element of the array, rather than write each line individually as in the preceding example. However, the example clearly illustrates the array syntax. You will learn about the various looping constructs ( for , while , and do-while ) in the Control Flow section.
Declaring a Variable to Refer to an Array
The preceding program declares an array (named anArray ) with the following line of code:
Like declarations for variables of other types, an array declaration has two components: the array's type and the array's name. An array's type is written as type [] , where type is the data type of the contained elements; the brackets are special symbols indicating that this variable holds an array. The size of the array is not part of its type (which is why the brackets are empty). An array's name can be anything you want, provided that it follows the rules and conventions as previously discussed in the naming section. As with variables of other types, the declaration does not actually create an array; it simply tells the compiler that this variable will hold an array of the specified type.
Similarly, you can declare arrays of other types:
You can also place the brackets after the array's name:
However, convention discourages this form; the brackets identify the array type and should appear with the type designation.
Creating, Initializing, and Accessing an Array
One way to create an array is with the new operator. The next statement in the ArrayDemo program allocates an array with enough memory for 10 integer elements and assigns the array to the anArray variable.
If this statement is missing, then the compiler prints an error like the following, and compilation fails:
The next few lines assign values to each element of the array:
Each array element is accessed by its numerical index:
Alternatively, you can use the shortcut syntax to create and initialize an array:
Here the length of the array is determined by the number of values provided between braces and separated by commas.
You can also declare an array of arrays (also known as a multidimensional array) by using two or more sets of brackets, such as String[][] names . Each element, therefore, must be accessed by a corresponding number of index values.
In the Java programming language, a multidimensional array is an array whose components are themselves arrays. This is unlike arrays in C or Fortran. A consequence of this is that the rows are allowed to vary in length, as shown in the following MultiDimArrayDemo program:
Finally, you can use the built-in length property to determine the size of any array. The following code prints the array's size to standard output:
Copying Arrays
The System class has an arraycopy method that you can use to efficiently copy data from one array into another:
The two Object arguments specify the array to copy from and the array to copy to . The three int arguments specify the starting position in the source array, the starting position in the destination array, and the number of array elements to copy.
The following program, ArrayCopyDemo , declares an array of String elements. It uses the System.arraycopy method to copy a subsequence of array components into a second array:
Array Manipulations
Arrays are a powerful and useful concept used in programming. Java SE provides methods to perform some of the most common manipulations related to arrays. For instance, the ArrayCopyDemo example uses the arraycopy method of the System class instead of manually iterating through the elements of the source array and placing each one into the destination array. This is performed behind the scenes, enabling the developer to use just one line of code to call the method.
For your convenience, Java SE provides several methods for performing array manipulations (common tasks, such as copying, sorting and searching arrays) in the java.util.Arrays class. For instance, the previous example can be modified to use the copyOfRange method of the java.util.Arrays class, as you can see in the ArrayCopyOfDemo example. The difference is that using the copyOfRange method does not require you to create the destination array before calling the method, because the destination array is returned by the method:
As you can see, the output from this program is the same, although it requires fewer lines of code. Note that the second parameter of the copyOfRange method is the initial index of the range to be copied, inclusively, while the third parameter is the final index of the range to be copied, exclusively . In this example, the range to be copied does not include the array element at index 9 (which contains the string Lungo ).
Some other useful operations provided by methods in the java.util.Arrays class are:
Searching an array for a specific value to get the index at which it is placed (the binarySearch method).
Comparing two arrays to determine if they are equal or not (the equals method).
Filling an array to place a specific value at each index (the fill method).
Sorting an array into ascending order. This can be done either sequentially, using the sort method, or concurrently, using the parallelSort method introduced in Java SE 8. Parallel sorting of large arrays on multiprocessor systems is faster than sequential array sorting.
Creating a stream that uses an array as its source (the stream method). For example, the following statement prints the contents of the copyTo array in the same way as in the previous example:
See Aggregate Operations for more information about streams.
Converting an array to a string. The toString method converts each element of the array to a string, separates them with commas, then surrounds them with brackets. For example, the following statement converts the copyTo array to a string and prints it:
This statement prints the following:
About Oracle | Contact Us | Legal Notices | Terms of Use | Your Privacy Rights
Copyright © 1995, 2022 Oracle and/or its affiliates. All rights reserved.
Learn Java practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Get Started With Java
- Your First Java Program
- Java Comments
Java Fundamentals
- Java Variables and Literals
- Java Data Types (Primitive)
- Java Operators
- Java Basic Input and Output
- Java Expressions, Statements and Blocks
Java Flow Control
- Java if...else Statement
- Java Ternary Operator
- Java for Loop
Java for-each Loop
- Java while and do...while Loop
- Java break Statement
- Java continue Statement
- Java switch Statement
Java Arrays
Java Multidimensional Arrays
Java Copy Arrays
Java OOP(I)
- Java Class and Objects
- Java Methods
- Java Method Overloading
- Java Constructors
- Java Static Keyword
- Java Strings
- Java Access Modifiers
- Java this Keyword
- Java final keyword
- Java Recursion
- Java instanceof Operator
Java OOP(II)
- Java Inheritance
- Java Method Overriding
- Java Abstract Class and Abstract Methods
- Java Interface
- Java Polymorphism
- Java Encapsulation
Java OOP(III)
- Java Nested and Inner Class
- Java Nested Static Class
- Java Anonymous Class
- Java Singleton Class
- Java enum Constructor
- Java enum Strings
- Java Reflection
- Java Package
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java ArrayList
- Java Vector
- Java Stack Class
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet Class
- Java EnumSet
- Java LinkedHashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator Interface
- Java ListIterator Interface
Java I/o Streams
- Java I/O Streams
- Java InputStream Class
- Java OutputStream Class
- Java FileInputStream Class
- Java FileOutputStream Class
- Java ByteArrayInputStream Class
- Java ByteArrayOutputStream Class
- Java ObjectInputStream Class
- Java ObjectOutputStream Class
- Java BufferedInputStream Class
- Java BufferedOutputStream Class
- Java PrintStream Class
Java Reader/Writer
- Java File Class
- Java Reader Class
- Java Writer Class
- Java InputStreamReader Class
- Java OutputStreamWriter Class
- Java FileReader Class
- Java FileWriter Class
- Java BufferedReader
- Java BufferedWriter Class
- Java StringReader Class
- Java StringWriter Class
- Java PrintWriter Class
Additional Topics
- Java Keywords and Identifiers
- Java Operator Precedence
- Java Bitwise and Shift Operators
- Java Scanner Class
- Java Type Casting
- Java Wrapper Class
- Java autoboxing and unboxing
- Java Lambda Expressions
- Java Generics
- Nested Loop in Java
- Java Command-Line Arguments
Java Tutorials
Java Math max()
- Java Math min()
- Java ArrayList trimToSize()
An array is a collection of similar types of data.
For example, if we want to store the names of 100 people then we can create an array of the string type that can store 100 names.
Here, the above array cannot store more than 100 names. The number of values in a Java array is always fixed.
- How to declare an array in Java?
In Java, here is how we can declare an array.
- dataType - it can be primitive data types like int , char , double , byte , etc. or Java objects
- arrayName - it is an identifier
For example,
Here, data is an array that can hold values of type double .
But, how many elements can array this hold?
Good question! To define the number of elements that an array can hold, we have to allocate memory for the array in Java. For example,
Here, the array can store 10 elements. We can also say that the size or length of the array is 10.
In Java, we can declare and allocate the memory of an array in one single statement. For example,
- How to Initialize Arrays in Java?
In Java, we can initialize arrays during declaration. For example,
Here, we have created an array named age and initialized it with the values inside the curly brackets.
Note that we have not provided the size of the array. In this case, the Java compiler automatically specifies the size by counting the number of elements in the array (i.e. 5).
In the Java array, each memory location is associated with a number. The number is known as an array index. We can also initialize arrays in Java, using the index number. For example,
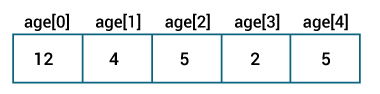
- Array indices always start from 0. That is, the first element of an array is at index 0.
- If the size of an array is n , then the last element of the array will be at index n-1 .
- How to Access Elements of an Array in Java?
We can access the element of an array using the index number. Here is the syntax for accessing elements of an array,
Let's see an example of accessing array elements using index numbers.
Example: Access Array Elements
In the above example, notice that we are using the index number to access each element of the array.
We can use loops to access all the elements of the array at once.
- Looping Through Array Elements
In Java, we can also loop through each element of the array. For example,
Example: Using For Loop
In the above example, we are using the for Loop in Java to iterate through each element of the array. Notice the expression inside the loop,
Here, we are using the length property of the array to get the size of the array.
We can also use the for-each loop to iterate through the elements of an array. For example,
Example: Using the for-each Loop
- Example: Compute Sum and Average of Array Elements
In the above example, we have created an array of named numbers . We have used the for...each loop to access each element of the array.
Inside the loop, we are calculating the sum of each element. Notice the line,
Here, we are using the length attribute of the array to calculate the size of the array. We then calculate the average using:
As you can see, we are converting the int value into double . This is called type casting in Java. To learn more about typecasting, visit Java Type Casting .
- Multidimensional Arrays
Arrays we have mentioned till now are called one-dimensional arrays. However, we can declare multidimensional arrays in Java.
A multidimensional array is an array of arrays. That is, each element of a multidimensional array is an array itself. For example,
Here, we have created a multidimensional array named matrix. It is a 2-dimensional array. To learn more, visit the Java multidimensional array .
- Java Copy Array
- Java Program to Print an Array
- Java Program to Concatenate two Arrays
- Java ArrayList to Array and Array to ArrayList
- Java Dynamic Array
Table of Contents
- Introduction
Sorry about that.
Our premium learning platform, created with over a decade of experience and thousands of feedbacks .
Learn and improve your coding skills like never before.
- Interactive Courses
- Certificates
- 2000+ Challenges
Related Tutorials
Java Tutorial
Java Library
How to Create an Array in Java – Array Declaration Example

Creating and manipulating arrays is an essential skill for any Java programmer. Arrays provide a way to store and organize multiple values of the same type, making it easier to work with large sets of data.
In this article, we will provide a step-by-step guide on how to create an array in Java, including how to initialize or create an array. We will also cover some advanced topics such as multi-dimensional arrays, array copying, and array sorting.
Whether you're new to Java programming or an experienced developer looking to refresh your skills, this article will provide you with the knowledge you need to become proficient in working with Java arrays.
What is an Array in Java?
In Java, an array is a data structure that can store a fixed-size sequence of elements of the same data type. An array is an object in Java, which means it can be assigned to a variable, passed as a parameter to a method, and returned as a value from a method.
Arrays in Java are zero-indexed, which means that the first element in an array has an index of 0, the second element has an index of 1, and so on. The length of an array is fixed when it is created and cannot be changed later.
Java arrays can store elements of any data type, including primitive types such as int, double, and boolean, as well as object types such as String and Integer. Arrays can also be multi-dimensional, meaning that they can have multiple rows and columns.
Arrays in Java are commonly used to store collections of data, such as a list of numbers, a set of strings, or a series of objects. By using arrays, we can access and manipulate collections of data more efficiently than using individual variables.
There are several ways to create an array in Java. In this section, we will cover some of the most common approaches to array declaration and initialization in Java.
How to Declare and Initialize and Array in Java in a Single Statement
Array declaration and initialization in a single statement is a concise and convenient way to create an array in Java. This approach allows you to declare and initialize an array using a single line of code.
To create an array in a single statement, you first declare the type of the array, followed by the name of the array, and then the values of the array enclosed in curly braces, separated by commas.
Here's an example:
In this example, we declare an array of integers named numbers and initialize it with the values 1, 2, 3, 4, and 5. The type of the array is int[] , indicating that it is an array of integers.
You can also create an array of a different data type, such as a string array. Here's an example:
In this example, we declare an array of strings named names and initialize it with the values "John", "Mary", "David", and "Sarah".
When using this approach, you don't need to specify the size of the array explicitly, as it is inferred.
How to Declare and Initialize an Array in Java in Separate Statements
Array declaration and initialization in separate statements is another way to create an array in Java. This approach involves declaring an array variable and then initializing it with values in a separate statement.
To create an array using this approach, you first declare the array variable using the syntax datatype[] arrayName , where datatype is the type of data the array will hold, and arrayName is the name of the array.
In this example, we declare an array of integers named numbers , but we haven't initialized it with any values yet.
To initialize the array with values, we use the new keyword followed by the type of data the array will hold, enclosed in square brackets, and the number of elements the array will contain. Here's an example:
In this example, we initialize the numbers array with the values 1, 2, 3, 4, and 5. Note that we use the syntax {value1, value2, ..., valueN} to specify the values of the array.
You can also initialize the array with values in separate statements, like this:
In this example, we declare a string array named names and initialize it with the values "John", "Mary", "David", and "Sarah" in a separate statement.
Using this approach, you can also initialize the array with default values by omitting the values in the initialization statement. For example:
In this example, we declare a boolean array named flags and initialize it with 5 elements. Since we haven't specified any values, each element is initialized with the default value of false .
How to Declare an Array in Java with Default Values
Array declaration with default values is a way to create an array in Java and initialize it with default values of the specified data type. This approach is useful when you need to create an array with a fixed size, but you don't have specific values to initialize it with.
To create an array with default values, you first declare the array variable using the syntax datatype[] arrayName = new datatype[length] , where datatype is the type of data the array will hold, arrayName is the name of the array, and length is the number of elements the array will contain. Here's an example:
In this example, we declare an array of integers named numbers with a length of 5. Since we haven't specified any values, each element in the array is initialized with the default value of 0.
You can also create an array of a different data type and initialize it with default values. For example:
In this example, we declare an array of booleans named flags with a length of 3. Since we haven't specified any values, each element in the array is initialized with the default value of false .
When using this approach, it's important to note that the default values of an array depend on its data type. For example, the default value of an integer is 0, while the default value of a boolean is false . If the array holds objects, the default value is null .
Array declaration with default values is useful when you need to create an array with a fixed size, but you don't yet have specific values to initialize it with. You can later assign values to the elements of the array using indexing.
Multi-Dimensional Arrays in Java
A multi-dimensional array is an array that contains other arrays. In Java, you can create multi-dimensional arrays with two or more dimensions. A two-dimensional array is an array of arrays, while a three-dimensional array is an array of arrays of arrays, and so on.
To create a two-dimensional array in Java, you first declare the array variable using the syntax datatype[][] arrayName , where datatype is the type of data the array will hold, and arrayName is the name of the array. Here's an example:
In this example, we declare a two-dimensional array of integers named matrix , but we haven't initialized it with any values yet.
To initialize the array with values, we use the new keyword followed by the type of data the array will hold, enclosed in square brackets, and the number of rows and columns the array will contain. Here's an example:
In this example, we initialize the matrix array with 3 rows and 4 columns. Note that we use the syntax new datatype[rows][columns] to specify the dimensions of the array.
Here's another example of declaring a two-dimensional array of integers:
You can also create a multi-dimensional array with default values by omitting the values in the initialization statement. For example:
In this example, we declare a two-dimensional array of booleans named flags with 2 rows and 3 columns. Since we haven't specified any values, each element in the array is initialized with the default value of false .
Multi-dimensional arrays are useful when you need to store data in a table or grid-like structure, such as a chess board or a spreadsheet.
Creating arrays is a fundamental skill in Java programming. In this article, we covered four different approaches to array declaration and initialization in Java, including single-statement declaration and initialization, separate declaration and initialization, default values, and multi-dimensional arrays.
Understanding these different techniques will help you write more effective Java code and work more effectively with Java arrays.
Let's connect on Twitter and on LinkedIn . You can also subscribe to my YouTube channel.
Happy Coding!
Hello 👋 my name is Shittu Olumide; I am a software engineer and technical writer, compassionate about the community and its members.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- Trending Categories

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Assigning arrays in Java.
While creating variables first of all we will declare them, initialize them, assign/re-assign values to them.
Similarly, while creating arrays −
You can declare an array just like a variable −
You can create an array just like an object using the new keyword −
You can initialize the array by assigning values to all the elements one by one using the index −

Assigning values to an array
When we assign primitive values of one type to a variable of other (datatype) implicitly they are converted.
But, when you try to assign a higher datatype to lower, at the time of compilation you will get an error saying “incompatible types: possible lossy conversion”
Therefore while assigning values to the elements of an array you can assign any value which will be cast implicitly.
But, if you try to assign incompatible types (higher types) to a variable a compile-time error will be generated saying “possible lossy conversion”.
Live Demo
Compile-time error
If you have an array of objects while assigning values to the elements of it, you need to make sure that the objects you assign should be of the same or, a subtype of the class (which is the type of the array).
But to an array of objects if you assign a value which is neither the declared type nor its subtype a compile-time error will be generated.
If you have created an array of objects of an interface type you need to make sure that the values, you assign to the elements of the array are the objects of the class that implements the said interface.

- Related Articles
- Assigning values to static final variables in java\n
- Assigning long values carefully in java to avoid overflow\n
- Final Arrays in Java
- String Arrays in Java
- Most Profit Assigning Work in C++
- Assigning function to variable in JavaScript?
- Compare Two Java int Arrays in Java
- Streams on Arrays in Java 8
- How to process Arrays in Java?
- What is length in Java Arrays?
- Intersection of two arrays in Java
- Merge two sorted arrays in Java
- Sort arrays of objects in Java
- Dump Multi-Dimensional arrays in Java
- Merge k sorted arrays in Java
Kickstart Your Career
Get certified by completing the course
Array Operations in Java
Last updated: January 8, 2024
It's finally here:
>> The Road to Membership and Baeldung Pro .
Going into ads, no-ads reading , and bit about how Baeldung works if you're curious :)
Azure Container Apps is a fully managed serverless container service that enables you to build and deploy modern, cloud-native Java applications and microservices at scale. It offers a simplified developer experience while providing the flexibility and portability of containers.
Of course, Azure Container Apps has really solid support for our ecosystem, from a number of build options, managed Java components, native metrics, dynamic logger, and quite a bit more.
To learn more about Java features on Azure Container Apps, visit the documentation page .
You can also ask questions and leave feedback on the Azure Container Apps GitHub page .
Java applications have a notoriously slow startup and a long warmup time. The CRaC (Coordinated Restore at Checkpoint) project from OpenJDK can help improve these issues by creating a checkpoint with an application's peak performance and restoring an instance of the JVM to that point.
To take full advantage of this feature, BellSoft provides containers that are highly optimized for Java applications. These package Alpaquita Linux (a full-featured OS optimized for Java and cloud environment) and Liberica JDK (an open-source Java runtime based on OpenJDK).
These ready-to-use images allow us to easily integrate CRaC in a Spring Boot application:
Improve Java application performance with CRaC support
Modern software architecture is often broken. Slow delivery leads to missed opportunities, innovation is stalled due to architectural complexities, and engineering resources are exceedingly expensive.
Orkes is the leading workflow orchestration platform built to enable teams to transform the way they develop, connect, and deploy applications, microservices, AI agents, and more.
With Orkes Conductor managed through Orkes Cloud, developers can focus on building mission critical applications without worrying about infrastructure maintenance to meet goals and, simply put, taking new products live faster and reducing total cost of ownership.
Try a 14-Day Free Trial of Orkes Conductor today.
To learn more about Java features on Azure Container Apps, you can get started over on the documentation page .
And, you can also ask questions and leave feedback on the Azure Container Apps GitHub page .
Whether you're just starting out or have years of experience, Spring Boot is obviously a great choice for building a web application.
Jmix builds on this highly powerful and mature Boot stack, allowing devs to build and deliver full-stack web applications without having to code the frontend. Quite flexibly as well, from simple web GUI CRUD applications to complex enterprise solutions.
Concretely, The Jmix Platform includes a framework built on top of Spring Boot, JPA, and Vaadin , and comes with Jmix Studio, an IntelliJ IDEA plugin equipped with a suite of developer productivity tools.
The platform comes with interconnected out-of-the-box add-ons for report generation, BPM, maps, instant web app generation from a DB, and quite a bit more:
>> Become an efficient full-stack developer with Jmix
DbSchema is a super-flexible database designer, which can take you from designing the DB with your team all the way to safely deploying the schema .
The way it does all of that is by using a design model , a database-independent image of the schema, which can be shared in a team using GIT and compared or deployed on to any database.
And, of course, it can be heavily visual, allowing you to interact with the database using diagrams, visually compose queries, explore the data, generate random data, import data or build HTML5 database reports.
>> Take a look at DBSchema
Get non-trivial analysis (and trivial, too!) suggested right inside your IDE or Git platform so you can code smart, create more value, and stay confident when you push.
Get CodiumAI for free and become part of a community of over 280,000 developers who are already experiencing improved and quicker coding.
Write code that works the way you meant it to:
>> CodiumAI. Meaningful Code Tests for Busy Devs
The AI Assistant to boost Boost your productivity writing unit tests - Machinet AI .
AI is all the rage these days, but for very good reason. The highly practical coding companion, you'll get the power of AI-assisted coding and automated unit test generation . Machinet's Unit Test AI Agent utilizes your own project context to create meaningful unit tests that intelligently aligns with the behavior of the code. And, the AI Chat crafts code and fixes errors with ease, like a helpful sidekick.
Simplify Your Coding Journey with Machinet AI :
>> Install Machinet AI in your IntelliJ
Since its introduction in Java 8, the Stream API has become a staple of Java development. The basic operations like iterating, filtering, mapping sequences of elements are deceptively simple to use.
But these can also be overused and fall into some common pitfalls.
To get a better understanding on how Streams work and how to combine them with other language features, check out our guide to Java Streams:
Download the E-book
Do JSON right with Jackson
Get the most out of the Apache HTTP Client
Get Started with Apache Maven:
Working on getting your persistence layer right with Spring?
Explore the eBook
Building a REST API with Spring?
Get started with Spring and Spring Boot, through the Learn Spring course:
Explore Spring Boot 3 and Spring 6 in-depth through building a full REST API with the framework:
>> The New “REST With Spring Boot”
Get started with Spring and Spring Boot, through the reference Learn Spring course:
>> LEARN SPRING
Yes, Spring Security can be complex, from the more advanced functionality within the Core to the deep OAuth support in the framework.
I built the security material as two full courses - Core and OAuth , to get practical with these more complex scenarios. We explore when and how to use each feature and code through it on the backing project .
You can explore the course here:
>> Learn Spring Security
Spring Data JPA is a great way to handle the complexity of JPA with the powerful simplicity of Spring Boot .
Get started with Spring Data JPA through the guided reference course:
>> CHECK OUT THE COURSE
1. Overview
Any Java developer knows that producing a clean, efficient solution when working with array operations isn’t always easy to achieve. Still, they’re a central piece in the Java ecosystem – and we’ll have to deal with them on several occasions.
For this reason, it’s good to have a ‘cheat sheet’ – a summary of the most common procedures to help us tackle the puzzle quickly. This tutorial will come in handy in those situations.
2. Arrays and Helper Classes
Before proceeding, it’s useful to understand what is an array in Java, and how to use it. If it’s your first time working with it in Java, we suggest having a look at this previous post where we covered all basic concepts.
Please note that the basic operations that an array supports are, in a certain way, limited. It’s not uncommon to see complex algorithms to execute relatively simple tasks when it comes to arrays.
For this reason, for most of our operations, we’ll be using helper classes and methods to assist us: the Arrays class provided by Java and the Apache’s ArrayUtils one.
To include the latter in our project, we’ll have to add the Apache Commons dependency:
We can check out the latest version of this artifact on Maven Central .
3. Get the First and Last Element of an Array
This is one of the most common and simple tasks thanks to the access-by-index nature of arrays.
Let’s start by declaring and initializing an int array that will be used in all our examples (unless we specify otherwise):
Knowing that the first item of an array is associated with the index value 0 and that it has a length attribute that we can use, then it’s simple to figure out how we can get these two elements:
4. Get a Random Value from an Array
By using the java.util.Random object we can easily get any value from our array:
5. Append a New Item to an Array
As we know, arrays hold a fixed size of values. Therefore, we can’t just add an item and exceed this limit.
We’ll need to start by declaring a new, larger array, and copy the elements of the base array to the second one.
Fortunately, the Arrays class provides a handy method to replicate the values of an array to a new different-sized structure:
Optionally, if the ArrayUtils class is accessible in our project, we can make use of its add method (or its addAll alternative) to accomplish our objective in a one-line statement:
As we can imagine, this method doesn’t modify the original array object; we have to assign its output to a new variable.
6. Insert a Value Between Two Values
Because of its indexed-values character, inserting an item in an array between two others is not a trivial job.
Apache considered this a typical scenario and implemented a method in its ArrayUtils class to simplify the solution:
We have to specify the index in which we want to insert the value, and the output will be a new array containing a larger number of elements.
The last argument is a variable argument (a.k.a. vararg ) thus we can insert any number of items in the array.
7. Compare Two Arrays
Even though arrays are Object s and therefore provide an equals method, they use the default implementation of it, relying only on reference equality.
We can anyhow invoke the java.util.Arrays ‘ equals method to check if two array objects contain the same values:
Note: this method is not effective for jagged arrays . The appropriate method to verify multi-dimensional structures’ equality is the Arrays.deepEquals one.
8. Check if an Array Is Empty
This is an uncomplicated assignment having in mind that we can use the length attribute of arrays:
Moreover, we also have a null-safe method in the ArrayUtils helper class that we can use:
This function still depends on the length of the data structure, which considers nulls and empty sub-arrays as valid values too, so we’ll have to keep an eye on these edge cases:
9. How to Shuffle the Elements of an Array
In order to shuffle the items in an array, we can use the ArrayUtil ‘s feature:
This is a void method and operates on the actual values of the array.
10. Box and Unbox Arrays
We often come across methods that support only Object -based arrays.
Again the ArrayUtils helper class comes in handy to get a boxed version of our primitive array:
The inverse operation is also possible:
11. Remove Duplicates from an Array
The easiest way of removing duplicates is by converting the array to a Set implementation.
As we may know, Collection s use Generics and hence don’t support primitive types.
For this reason, if we’re not handling object-based arrays as in our example, we’ll first need to box our values:
Note: we can use other techniques to convert between an array and a Set object as well.
Also, if we need to preserve the order of our elements, we must use a different Set implementation, such as a LinkedHashSet .
12. How to Print an Array
Same as with the equals method, the array’s toString function uses the default implementation provided by the Object class, which isn’t very useful.
Both Arrays and ArrayUtils classes ship with their implementations to convert the data structures to a readable String .
Apart from the slightly different format they use, the most important distinction is how they treat multi-dimensional objects.
The Java Util’s class provides two static methods we can use:
- toString : doesn’t work well with jagged arrays
- deepToString : supports any Object -based arrays but doesn’t compile with primitive array arguments
On the other hand, Apache’s implementation offers a single toString method that works correctly in any case:
13. Map an Array to Another Type
It’s often useful to apply operations on all array items, possibly converting them to another type of object.
With this objective in mind, we’ll try to create a flexible helper method using Generics:
If we don’t use Java 8 in our project, we can discard the Function argument, and create a method for each mapping that we need to carry out.
We can now reuse our generic method for different operations. Let’s create two test cases to illustrate this:
For primitive types, we’ll have to box our values first.
As an alternative, we can turn to Java 8’s Streams to carry out the mapping for us.
We’ll need to transform the array into a Stream of Object s first. We can do so with the Arrays.stream method.
For example, if we want to map our int values to a custom String representation, we’ll implement this:
14. Filter Values in an Array
Filtering out values from a collection is a common task that we might have to perform in more than one occasion.
This is because at the time we create the array that will receive the values, we can’t be sure of its final size. Therefore, we’ll rely on the Stream s approach again.
Imagine we want to remove all odd numbers from an array:
15. Other Common Array Operations
There are, of course, plenty of other array operations that we might need to perform.
Apart from the ones shown in this tutorial, we’ve extensively covered other operations in the dedicated posts:
- Check if a Java Array Contains a Value
- How to Copy an Array in Java
- Removing the First Element of an Array
- Finding the Min and Max in an Array with Java
- Find Sum and Average in a Java Array
- How to Invert an Array in Java
- Join and Split Arrays and Collections in Java
- Combining Different Types of Collections in Java
- Find All Pairs of Numbers in an Array That Add Up to a Given Sum
- Sorting in Java
- Efficient Word Frequency Calculator in Java
- Insertion Sort in Java
16. Conclusion
Arrays are one of the core functionalities of Java, and therefore it’s really important to understand how they work and to know what we can and can’t do with them.
In this tutorial, we learned how we can handle array operations appropriately in common scenarios.
As always, the full source code of the working examples is available over on our Github repo .
Looking for the ideal Linux distro for running modern Spring apps in the cloud?
Meet Alpaquita Linux : lightweight, secure, and powerful enough to handle heavy workloads.
This distro is specifically designed for running Java apps . It builds upon Alpine and features significant enhancements to excel in high-density container environments while meeting enterprise-grade security standards.
Specifically, the container image size is ~30% smaller than standard options, and it consumes up to 30% less RAM:
>> Try Alpaquita Containers now.
Explore the secure, reliable, and high-performance Test Execution Cloud built for scale. Right in your IDE:
Basically, write code that works the way you meant it to.
AI is all the rage these days, but for very good reason. The highly practical coding companion, you'll get the power of AI-assisted coding and automated unit test generation . Machinet's Unit Test AI Agent utilizes your own project context to create meaningful unit tests that intelligently aligns with the behavior of the code.
Get started with Spring Boot and with core Spring, through the Learn Spring course:
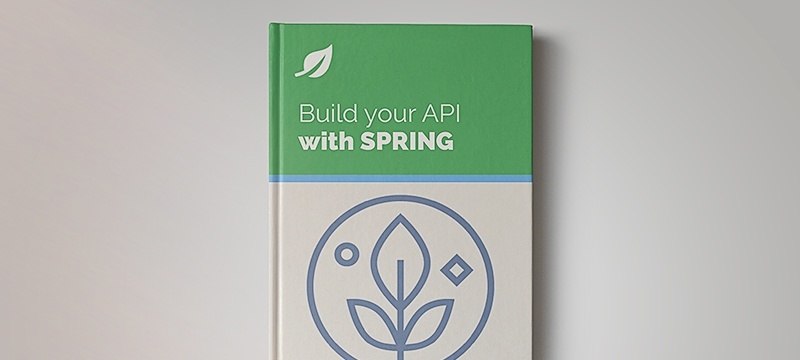

How to Create an Array in Java – Array Declaration Example

Arrays are one of the most commonly used data structures in Java. An array is an object that stores a fixed-size sequence of elements of the same type. Knowing how to create, initialize, and manipulate arrays is critical for any Java programmer.
In this comprehensive tutorial, we will explore arrays in Java in depth. We will cover:
- What are arrays and why they are useful
- Declaring array variables
- Initializing arrays
- Multidimensional arrays
- Accessing and modifying array elements
- Iterating over arrays with loops
- Sorting and searching arrays
- Copying arrays
- Array limitations
- And more…
By the end of this guide, you will have a deep understanding of arrays in Java with plenty of examples for reference. Let‘s get started!
What is an Array in Java?
An array in Java is an object that contains a fixed number of values of the same data type. The values that an array holds are called its elements.
For example, we can have an array that holds the numbers 1, 2, 3:
Here we have declared an integer array named numbers that contains three elements.
Arrays make it easy to organize data of the same type and access it via indices. Each slot or index in the array corresponds to an element.
In Java, arrays are zero-indexed – the first element is accessed via index 0, second element via index 1 and so on. The length of an array indicates how many total elements it can hold.
Arrays are extremely useful when you need to store multiple values of the same type, like scores from a game, names in a database, or coordinates in a graph.
Now let‘s see how to actually create arrays in Java code.
Declaring Arrays in Java
To use arrays in Java, you first need to declare a variable that can hold the array. Array declarations specify:
- Data type – the type of elements that the array will hold
- Name – a unique name to reference the array
- Brackets [] – indicates this is an array variable
Here is the general syntax for declaring an array in Java:
For example, to declare an array that can hold integers:
This statement declares a variable named numbers that can hold an integer array.
Note that when you declare an array, it merely allocates memory for the array reference and does not actually create the array itself or allocate memory for the elements.
To summarize, declaring an array variable is necessary before you work with arrays in Java. It reserves memory for the array reference. However, declaration alone does not reserve memory for the array elements themselves.
Initializing Arrays in Java
After declaring an array variable, the next step is to actually create the array in memory and assign it to the variable. This process is called array initialization.
Arrays can be initialized in a few different ways in Java:
1. Initialize During Declaration
You can initialize an array during declaration by including the array elements directly inside { } braces:
Here we have declared and initialized the numbers array in a single line. It is created with four integer elements already assigned.
When you initialize using { } braces directly, the Java compiler automatically counts the elements and sets the array length.
2. Separate Declaration and Initialization
Alternatively, you can first declare the array variable, then create the actual array separately:
Here‘s a breakdown of what‘s happening:
- First we declare an integer array named numbers
- Later, we create a new integer array of length 4 and assign it to numbers
Unlike brace initialization, using the new keyword allows you to specify the exact length or size of the array. The array length cannot be changed after creation.
And here is how you can initialize the array elements after determining its length:
By using index numbers, we can assign values to each element of the integers array.
3. Initialize with Default Values
The third way to initialize arrays in Java is by specifying only its length to fill it with default values:
Here we created an integer array of length 5. However, we did not explicitly initialize the elements.
In this case, the array is filled with the default value for the data type:
- 0 for numeric types like int, double etc.
- false for boolean
- null for objects like String
This creates an array with elements already set to default values.
In summary, there are a few different ways to initialize arrays in Java. The approach you take depends on whether you want to specify the values yourself or let Java initialize with defaults.
Multidimensional Arrays in Java
So far we have looked at single-dimensional arrays that represent a row of values. Java also provides multidimensional arrays that add additional dimensions.
Common types of multidimensional arrays include:
- Two dimensional arrays (2D) – represented as rows and columns
- Three dimensional arrays (3D) – represented as stacks of 2D arrays
And so on for higher dimensions.
Multidimensional arrays are useful for mathematical matrices, grids for games like chess or tic-tac-toe, and pixel data for images.
Declaring Multidimensional Arrays
To declare a multidimensional array in Java, add additional square brackets [] for each dimension:
The general syntax is:
For example, int[][][] declares a 3 dimensional integer array variable.
You can visualize each additional [] as representing an additional axis or dimension.
Initializing Multidimensional Arrays
Similar to single arrays, multidimensional arrays must also be initialized after declaration using either array literals or the new keyword.
For example, to initialize a 5 x 3 two-dimensional integer array (5 rows, 3 columns):
Here [5] specifies length along the first dimension (number of rows) and [3] specifies length along the second dimension (number of columns in each row).
You can also manually initialize elements similar to single arrays:
In this example:
- First { } contains rows
- Second { } contains columns within each row
The syntax extends similarly for higher dimensions.
For example, a 3 dimensional array would be declared and initialized as:
In summary, multidimensional arrays provide a mechanism to store tabular data structures in Java. The extra dimensions help organize data for easy access.
Accessing and Modifying Array Elements
A key benefit of using arrays is that you can read and update individual elements easily.
To access a particular element in an array, specify its index enclosed in square brackets [] after the array name:
Remember that arrays are zero indexed, so the index starts from 0.
You can also modify elements using index assignment:
The same concept applies for multidimensional arrays, with additional indices specifying positions along each dimension.
For example, in a 2D matrix array:
Using indices, you can easily read or modify any array element.
Iterating Arrays with Loops
A common task when working with arrays is to iterate through all the elements rather than accessing individual indices.
For example, you may want to perform some calculation on each element. Or search through elements to find a particular value.
In Java, you can iterate through arrays using standard for and enhanced for loops.
Here‘s an example printing all elements with a standard for loop:
Breaking down the pieces:
- i starts from 0 up to the array‘s length
- We access each element via its index
And printing using the enhanced for loop:
Where number takes the value of the current element automatically on each iteration.
The same idea extends to iterating multidimensional arrays using nested loops.
Here is an example printing a 2D matrix:
Often, iterating arrays with loops is more convenient than using hard-coded indices.
Sorting Arrays
Sorting refers to arranging the elements of an array in a certain order – numerically, alphabetically or by some condition.
For example, you may want to sort a list of names alphabetically or sort exam results from highest to lowest score.
The Arrays class provides useful static methods for sorting:
- sort() – sorts array elements alphabetically or numerically
- parallelSort() – optimized multi-threaded sorting
For instance, to sort an integer array:
You can also provide a custom Comparator to define complex sorting logic.
Here is an example sorting strings by length:
The compare() method determines how elements are sorted relative to each other.
In summary, Arrays.sort() provides a simple yet powerful way to sort the elements of any array.
Searching Elements in Arrays
Another common array operation is searching – finding the index of a particular value within the array.
The Arrays class contains several search methods:
- binarySearch() – faster search using Binary Search algorithm
- linearSearch() – simpler linear scan search
For example, to search for a value in a sorted integer array:
And linear search on an unsorted array:
Arrays.binarySearch() requires the array to be sorted while Arrays.asList().indexOf() performs a simple linear scan.
So when you need to check if a value exists in an array or find its index, use Java‘s built-in search methods.
Copying Arrays
There may be cases where you want to copy data between arrays rather than directly modify the original.
For example, when getting items from a database into an array for manipulation before storing back.
The System class provides useful methods for copying arrays:
- arraycopy() – copy elements from one array into another
- copyOf() – copy elements and place them in a new array
Here is an example of using arraycopy() :
This copies all 3 elements from source into destination .
And clone using copyOf() :
Where the second argument passed is the length of the copied array.
So if you want to reuse array data without directly modifying it, leverage Java‘s built-in copy methods.
Array Limitations
While arrays seem very flexible, there are some limitations to consider:
- Fixed length – once created, an array‘s size cannot be changed
- Fixed type – all elements in an array must be of the same data type. For example, you cannot insert a String into an int[]
- Contains only references for objects – arrays of custom objects store references rather references than actual objects
Because of these constraints, Java also includes more flexible structures like the ArrayList class from the Collections framework. ArrayLists can grow or shrink dynamically.
However, arrays still provide fast fixed-size storage and access which can be useful in many cases.
Arrays provide a simple yet powerful way to organize large amounts of data and access elements via indices.
In Java, you can declare array variables, initialize them to reserve memory, and then manipulate elements using indices or loops. The multidimensional and variable-length array capabilities make arrays flexible for most common programming scenarios.
Java also provides many utility methods to search, copy and transform array contents easily. Understanding how to leverage arrays and these helper methods will let you write code that efficiently manages data.
This guide covered arrays in Java in comprehensive detail along with plenty of code examples. You should now feel comfortable declaring, initializing and working with arrays in your Java projects.
Let me know if you have any other array-related questions!
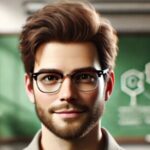
Dr. Alex Mitchell is a dedicated coding instructor with a deep passion for teaching and a wealth of experience in computer science education. As a university professor, Dr. Mitchell has played a pivotal role in shaping the coding skills of countless students, helping them navigate the intricate world of programming languages and software development.
Beyond the classroom, Dr. Mitchell is an active contributor to the freeCodeCamp community, where he regularly shares his expertise through tutorials, code examples, and practical insights. His teaching repertoire includes a wide range of languages and frameworks, such as Python, JavaScript, Next.js, and React, which he presents in an accessible and engaging manner.
Dr. Mitchell’s approach to teaching blends academic rigor with real-world applications, ensuring that his students not only understand the theory but also how to apply it effectively. His commitment to education and his ability to simplify complex topics have made him a respected figure in both the university and online learning communities.
Similar Posts

DAT File – How to Open the Mysterious .dat File Format
DAT files are common, yet mysterious. You may have come across a .dat file before and…

What is Glassmorphism? An In-Depth Guide to the New Design Trend with Code Examples
As a full-stack developer with over 5 years of interface design experience, I‘ve witnessed many UI…
An Introduction to Q-Learning: Reinforcement Learning from a Full-Stack Perspective
*Photo by [Franck V.](https://unsplash.com/@franckinjapan?utm_source=unsplash&utm_medium=referral&utm_content=creditCopyText) on [Unsplash](https://unsplash.com/s/photos/robot-arm?utm_source=unsplash&utm_medium=referral&utm_content=creditCopyText)* As a full-stack developer, I regularly apply machine learning techniques…

How to Build a Convolutional Neural Network for Sign Language Recognition: A 3600-word Practical Guide
Chapter 1: Introduction Sign languages are complete, complex visual-spatial languages that enable communication via hand shapes,…

Coding Interview Prep for Big Tech (FAANG) – And How I Became A Google Engineer
Transitioning from law to software engineering at Google in my late 30s was a massive challenge….

900 Free Computer Science Courses from the World’s Top Universities: The Ultimate Guide for Learners and Developers
As a full-stack developer and lifelong learner, I‘m fascinated by the world-class computer science education now…
- Java Course
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
Interesting facts about Array assignment in Java
Prerequisite : Arrays in Java
While working with arrays we have to do 3 tasks namely declaration, creation, initialization or Assignment. Declaration of array :
Creation of array :
Initialization of array :
Some important facts while assigning elements to the array:
Reference : https://docs.oracle.com/javase/specs/jls/se7/html/jls-10.html
Please Login to comment...
Similar reads.
- Interesting-Facts
- Java-Array-Programs
- Java-Arrays
- How to Get a Free SSL Certificate
- Best SSL Certificates Provider in India
- Elon Musk's xAI releases Grok-2 AI assistant
- What is OpenAI SearchGPT? How it works and How to Get it?
- Content Improvement League 2024: From Good To A Great Article
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
String Array Assignment in Java [duplicate]
Error at Line 2: Illegal Start of expression The code works if I say:
Why does this happen, and how should I do the required without generating an error? This also happens with other data types. Would appreciate if you could explain in layman terms .
- variable-assignment
- 4 The compiler want's you to specifiy that {"foo", ...} is meant to be an array, so use new String[]{"foo", ...} . Besides that, do yourself a favor and let variable names start with a lower case character, i.e. String foo instead of String Foo (and I personally like to have the array declaration at the type, i.e. String[] foo ). – Thomas Commented Sep 24, 2018 at 9:24
- Initialization and Assignment these are two words that makes the difference. I believe. :) – miiiii Commented Sep 24, 2018 at 9:28
- FYI: alternatively, for unmodifiable list rather than simple array, List<String> list = List.of( "foo" , "bar" ) ; as a single line or two lines. – Basil Bourque Commented Sep 24, 2018 at 9:30
- normally you have to use new String[] { ...} (this was an always must in earlier versions of Java), but, as a syntactic sugar, the compiler accepts just { ... } in a field declaration or variable declaration. Why? it is specified that way in the Java Language Specification (probably it would be to messy to accept it everywhere) – user85421 Commented Sep 24, 2018 at 9:44
2 Answers 2
{"foo","Foo"} is an array initializer and it isn't a complete array creation expression :
An array initializer may be specified in a declaration (§8.3, §9.3, §14.4), or as part of an array creation expression (§15.10), to create an array and provide some initial values. Java Specification
Use new String[] {"foo","Foo"} instead.
- What does '§' mean? – Stephen Allen Commented Sep 24, 2018 at 9:37
- @StephenAllen a link to a specification section – Andrew Tobilko Commented Sep 24, 2018 at 9:40
- 1 @StephenAllen actually it is just an abbreviation for Section Sign - apple.stackexchange.com/q/176968 or en.wiktionary.org/wiki § (happens to be a link to the given section in the Java Language Specification) – user85421 Commented Sep 24, 2018 at 9:52
You have to initialize the string array:
Modern IDEs give error for not initializing the array objects. You can refer more details here: http://grails.asia/java-string-array-declaration
Not the answer you're looking for? Browse other questions tagged java arrays variable-assignment or ask your own question .
- The Overflow Blog
- Where does Postgres fit in a world of GenAI and vector databases?
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Feedback requested: How do you use tag hover descriptions for curating and do...
- What does a new user need in a homepage experience on Stack Overflow?
- Staging Ground Reviewer Motivation
Hot Network Questions
- What was I thinking when I made this grid?
- Flight left while checked in passenger queued for boarding
- What did Jesus mean by 'cold water'' in Mtt 10:42?
- Why are most big lakes in North America aligned?
- How can I move TikZ pictures in 3D space?
- Historical U.S. political party "realignments"?
- \tl_gset_rescan with \c_str_cctab inserts unwanted tokens into resulting token list
- How to justify our beliefs so that it is not circular?
- A set of five (one is missing!)
- What did the Ancient Greeks think the stars were?
- What is the significance of bringing the door to Nippur in the Epic of Gilgamesh?
- Can light become a satellite of a black hole?
- Replacing a multi character pattern that includes a newline with some characters
- How can you trust a forensic scientist to have maintained the chain of custody?
- How bad would near constant dreary/gloomy/stormy weather on our or an Earthlike world be for us and the environment?
- ST_Curvetoline creates invalid geometries
- How would increasing atomic bond strength affect nuclear physics?
- Can I use "historically" to mean "for a long time" in "Historically, the Japanese were almost vegetarian"?
- What issues are there with my perspective on truth?
- Sci-fi short story about a dystopian future where all natural resources had been used up and people were struggling to survive
- Are quantum states like the W, Bell, GHZ, and Dicke state actually used in quantum computing research?
- The answer is not wrong
- Stealth Mosquitoes?
- I'm trying to remember a novel about an asteroid threatening to destroy the earth. I remember seeing the phrase "SHIVA IS COMING" on the cover

IMAGES
COMMENTS
Java does not provide a construct that will assign of multiple values to an existing array's elements. The initializer syntaxes can ONLY be used when creation a new array object. This can be at the point of declaration, or later on. But either way, the initializer is initializing a new array object, not updating an existing one.
Do refer to default array values in Java. Obtaining an array is a two-step process. First, you must declare a variable of the desired array type. Second, you must allocate the memory to hold the array, using new, and assign it to the array variable. Thus, in Java, all arrays are dynamically allocated.
Array Variable Assignment in Java. An array is a collection of similar types of data in a contiguous location in memory. After Declaring an array we create and assign it a value or variable. During the assignment variable of the array things, we have to remember and have to check the below condition. 1.
Static Array: Fixed size array (its size should be declared at the start and can not be changed later) Dynamic Array: No size limit is considered for this. (Pure dynamic arrays do not exist in Java. Instead, List is most encouraged.) To declare a static array of Integer, string, float, etc., use the below declaration and initialization statements.
Java Arrays. Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value. To declare an array, define the variable type with square brackets: We have now declared a variable that holds an array of strings. To insert values to it, you can place the values in a comma-separated list, inside ...
We can consider an array as a numbered list of cells, each cell being a variable holding a value. In Java, the numbering starts at 0. There are primitive type arrays and object type arrays. This means we can use arrays of int, float, boolean, …. But also arrays of String, Object and custom types as well. 3.
Java Array Loop Initialization; Array Declaration in Java. The declaration of an array object in Java follows the same logic as declaring a Java variable. We identify the data type of the array elements, and the name of the variable, while adding rectangular brackets [] to denote its an array. Here are two valid ways to declare an array:
Array Variable Assignment in Java. An array is a collection of similar types of data in a contiguous location in memory. After Declaring an array we create and assign it a value or variable. During the assignment variable of the array things, we have to remember and have to check the below condition. 1. Element Level Promotion Element-level ...
In the code example, we present five methods of the Arrays class. import java.util.Arrays; We will use the shorthand notation for the Arrays class. int[] a = { 5, 2, 4, 3, 1 }; We have an array of five integers. Arrays.sort(a); The sort method sorts the integers in an ascending order.
For your convenience, Java SE provides several methods for performing array manipulations (common tasks, such as copying, sorting and searching arrays) in the java.util.Arrays class. For instance, the previous example can be modified to use the copyOfRange method of the java.util.Arrays class, as you can see in the ArrayCopyOfDemo example.
To define the number of elements that an array can hold, we have to allocate memory for the array in Java. For example, // declare an array double[] data; // allocate memory. data = new double[10]; Here, the array can store 10 elements. We can also say that the size or length of the array is 10. In Java, we can declare and allocate the memory ...
So to create an array, you specify the data type that will be stored in the array followed by square brackets and then the name of the array. How to initialize an array in Java. To initialize an array simply means to assign values to the array. Let's initialize the arrays we declared in the previous section:
2. How to initialize an array in one line. You can initialize an array in one line with the basic syntax below: dataType [ ] nameOfArray = {value1, value2, value3, value4} With this method, you don't need to specify the size of the array, so you can put any number of values you want in it.
Java arrays can store elements of any data type, including primitive types such as int, double, and boolean, as well as object types such as String and Integer. ... You can later assign values to the elements of the array using indexing. Multi-Dimensional Arrays in Java. A multi-dimensional array is an array that contains other arrays. In Java ...
Advertisements. Assigning arrays in Java - While creating variables first of all we will declare them, initialize them, assign/re-assign values to them.Similarly, while creating arrays −You can declare an array just like a variable −int myArray [];You can create an array just like an object using the new keyword −myArray = new int [5];Yo.
var numbers = new int [ 7 ]; Copy. In the code above, we initialize a numbers array to hold seven elements of int type. After initialization, we can assign values to individual elements using their indices: numbers[ 0] = 10 ; numbers[ 1] = 20; Copy. Here, we add 10 and 20 to indices 0 and 1, respectively.
Copy. 4. Get a Random Value from an Array. By using the java.util.Random object we can easily get any value from our array: int anyValue = array[ new Random ().nextInt(array.length)]; Copy. 5. Append a New Item to an Array. As we know, arrays hold a fixed size of values.
Hence in order to add an element in the array, one of the following methods can be done: Create a new array of size n+1, where n is the size of the original array. Add the n elements of the original array in this array. Add the new element in the n+1 th position. Print the new array.
Initializing Arrays in Java. After declaring an array variable, the next step is to actually create the array in memory and assign it to the variable. This process is called array initialization. Arrays can be initialized in a few different ways in Java: 1. Initialize During Declaration
If you want to make a copy, you could use val1.clone() or Arrays.copyOf(): int[] val2 = Arrays.copyOf(val1, val1.length); Objects (including instances of collection classes, String, Integer etc) work in a similar manner, in that assigning one variable to another simply copies the reference, making both variables refer to the same object.
Array Variable Assignment in Java. An array is a collection of similar types of data in a contiguous location in memory. After Declaring an array we create and assign it a value or variable. During the assignment variable of the array things, we have to remember and have to check the below condition. 1. Element Level Promotion Element-level ...
An array initializer may be specified in a declaration (§8.3, §9.3, §14.4), or as part of an array creation expression (§15.10), to create an array and provide some initial values. Java Specification